svcore
1.9
|
SparseTimeValueModel.h
Go to the documentation of this file.
std::atomic< bool > m_haveExtents
Definition: SparseTimeValueModel.h:373
void toXml(QTextStream &stream, QString indent="", QString extraAttributes="") const override
Stream this exportable object out to XML on a text stream.
Definition: Model.cpp:204
static PlayParameterRepository * getInstance()
Definition: PlayParameterRepository.cpp:26
Command * getRemoveRowCommand(int row) override
Return a command to delete the row with the given index.
Definition: SparseTimeValueModel.h:315
bool isColumnTimeValue(int column) const override
Return true if the column is the frame time of the item, or an alternative representation of it (i...
Definition: SparseTimeValueModel.h:234
Command * getSetDataCommand(int row, int column, const QVariant &value, int role) override
Return a command to set the value in the given cell, for the given role, to the contents of the suppl...
Definition: SparseTimeValueModel.h:284
EventVector getEventsCovering(sv_frame_t f) const
Definition: SparseTimeValueModel.h:164
bool getNearestEventMatching(sv_frame_t startSearchAt, std::function< bool(Event)> predicate, EventSeries::Direction direction, Event &found) const
Definition: SparseTimeValueModel.h:177
sv_samplerate_t getSampleRate() const override
Return the frame rate in frames per second.
Definition: SparseTimeValueModel.h:102
QVariant getData(int row, int column, int role) const override
Get the value in the given cell, for the given role.
Definition: SparseTimeValueModel.h:265
EventVector getEventsStartingAt(sv_frame_t frame) const
Retrieve all events starting at exactly the given frame.
Definition: EventSeries.h:143
QVector< QVector< QString > > toStringExportRows(DataExportOptions options, sv_frame_t startFrame, sv_frame_t duration) const override
Emit events starting within the given range as string rows ready for conversion to an e...
Definition: SparseTimeValueModel.h:356
static QVariant adaptFrameForRole(sv_frame_t frame, sv_samplerate_t rate, int role)
Definition: TabularModel.h:124
void setCompletion(int completion, bool update=true)
Definition: SparseTimeValueModel.h:125
QString getTypeName() const override
Return the type of the model.
Definition: SparseTimeValueModel.h:88
int getRowForFrame(sv_frame_t frame) const override
Return the number of the first row whose frame time is not less than the given one.
Definition: SparseTimeValueModel.h:246
std::atomic< float > m_valueMaximum
Definition: SparseTimeValueModel.h:372
Event getEventByIndex(int index) const
Return the event at the given numerical index in the series, where 0 = the first event and count()-1 ...
Definition: EventSeries.cpp:547
void update(sv_frame_t frame, sv_frame_t duration)
Definition: DeferredNotifier.h:43
EventVector getAllEvents() const
Retrieve all events, in their natural order.
Definition: EventSeries.cpp:464
bool isSparse() const override
Return true if this is a sparse model.
Definition: SparseTimeValueModel.h:89
sv_frame_t getStartFrame() const
Return the frame of the first event in the series.
Definition: EventSeries.cpp:265
QVector< QVector< QString > > toStringExportRows(DataExportOptions options, sv_frame_t startFrame, sv_frame_t duration, sv_samplerate_t sampleRate, sv_frame_t resolution, Event fillEvent) const
Emit events starting within the given range as string rows ready for conversion to an e...
Definition: EventSeries.cpp:615
void toXml(QTextStream &out, QString indent="", QString extraAttributes="") const override
XmlExportable methods.
Definition: SparseTimeValueModel.h:327
Definition: DeferredNotifier.h:25
float getValueMaximum() const
Definition: SparseTimeValueModel.h:121
Definition: TabularModel.h:58
void makeDeferredNotifications()
Definition: DeferredNotifier.h:53
std::atomic< bool > m_haveTextLabels
Definition: SparseTimeValueModel.h:374
QVector< QString > getStringExportHeaders(DataExportOptions options, Event::ExportNameOptions) const
Return a label for each column that would be written by toStringExportRows.
Definition: EventSeries.cpp:604
Definition: TabularModel.h:58
int getIndexForEvent(const Event &e) const
Return the index of the first event in the series that does not compare inferior to the given event...
Definition: EventSeries.cpp:557
QString getHeading(int column) const override
Return the heading for a given column, e.g.
Definition: SparseTimeValueModel.h:250
SortType getSortType(int column) const override
Return the sort type (numeric or alphabetical) for the column.
Definition: SparseTimeValueModel.h:260
void toXml(QTextStream &out, QString indent, QString extraAttributes) const override
Emit to XML as a dataset element.
Definition: EventSeries.cpp:567
bool hasTextLabels() const
Definition: SparseTimeValueModel.h:118
EventVector getEventsStartingWithin(sv_frame_t f, sv_frame_t duration) const
Definition: SparseTimeValueModel.h:171
float getValueMinimum() const
Definition: SparseTimeValueModel.h:120
bool getDefaultPlayAudible() const override
Definition: SparseTimeValueModel.h:106
EventVector getEventsCovering(sv_frame_t frame) const
Retrieve all events that cover the given frame.
Definition: EventSeries.cpp:420
EventVector getAllEvents() const
Definition: SparseTimeValueModel.h:158
EventVector getEventsStartingAt(sv_frame_t f) const
Definition: SparseTimeValueModel.h:174
static QVariant adaptValueForRole(float value, QString unit, int role)
Definition: TabularModel.h:133
EventVector getEventsSpanning(sv_frame_t frame, sv_frame_t duration) const
Retrieve all events any part of which falls within the range in frames defined by the given frame f a...
Definition: EventSeries.cpp:294
SparseTimeValueModel(sv_samplerate_t sampleRate, int resolution, float valueMinimum, float valueMaximum, bool notifyOnAdd=true)
Definition: SparseTimeValueModel.h:62
QVector< QString > getStringExportHeaders(DataExportOptions options) const override
Return a label for each column that would be written by toStringExportRows.
Definition: SparseTimeValueModel.h:351
std::atomic< int > m_completion
Definition: SparseTimeValueModel.h:377
std::atomic< float > m_valueMinimum
Definition: SparseTimeValueModel.h:371
Model is the base class for all data models that represent any sort of data on a time scale based on ...
Definition: Model.h:51
TabularModel is an abstract base class for models that support direct access to data in a tabular for...
Definition: TabularModel.h:35
void completionChanged(ModelId myId)
Emitted when some internal processing has advanced a stage, but the model has not changed externally...
bool isEditable() const override
Return true if the model is user-editable, false otherwise.
Definition: SparseTimeValueModel.h:282
sv_frame_t getEndFrame() const
Return the frame plus duration of the event in the series that ends last.
Definition: EventSeries.cpp:273
ExportId getExportId() const
Return the numerical export identifier for this object.
Definition: XmlExportable.cpp:71
virtual ~SparseTimeValueModel()
Definition: SparseTimeValueModel.h:83
bool getNearestEventMatching(sv_frame_t startSearchAt, std::function< bool(const Event &)> predicate, Direction direction, Event &found) const
Return the first event for which the given predicate returns true, searching events with start frames...
Definition: EventSeries.cpp:508
sv_frame_t getStartFrame() const override
Return the first audio frame spanned by the model.
Definition: SparseTimeValueModel.h:92
A model representing a wiggly-line plot with points at arbitrary intervals of the model resolution...
Definition: SparseTimeValueModel.h:34
void modelChangedWithin(ModelId myId, sv_frame_t startFrame, sv_frame_t endFrame)
Emitted when a model has been edited (or more data retrieved from cache, in the case of a cached mode...
sv_frame_t getTrueEndFrame() const override
Return the audio frame at the end of the model.
Definition: SparseTimeValueModel.h:95
EventVector getEventsWithin(sv_frame_t frame, sv_frame_t duration, int overspill=0) const
Retrieve all events falling wholly within the range in frames defined by the given frame f and durati...
Definition: EventSeries.cpp:342
bool containsEvent(const Event &e) const
Definition: SparseTimeValueModel.h:155
An immutable(-ish) type used for point and event representation in sparse models, as well as for inte...
Definition: Event.h:55
EventVector getEventsWithin(sv_frame_t f, sv_frame_t duration, int overspill=0) const
Definition: SparseTimeValueModel.h:167
QString getScaleUnits() const
Definition: SparseTimeValueModel.h:108
void addPlayable(int id, const Playable *)
Register a playable.
Definition: PlayParameterRepository.cpp:36
Definition: Command.h:26
SparseTimeValueModel(sv_samplerate_t sampleRate, int resolution, bool notifyOnAdd=true)
Definition: SparseTimeValueModel.h:41
void setScaleUnits(QString units)
Definition: SparseTimeValueModel.h:112
Container storing a series of events, with or without durations, and supporting the ability to query ...
Definition: EventSeries.h:49
sv_frame_t getFrameForRow(int row) const override
Return the frame time for the given row.
Definition: SparseTimeValueModel.h:238
sv_samplerate_t m_sampleRate
Definition: SparseTimeValueModel.h:368
int getCompletion() const override
Return an estimated percentage value showing how far through any background operation used to calcula...
Definition: SparseTimeValueModel.h:123
Interface for classes that can be modified through these commands.
Definition: EventCommands.h:26
int getColumnCount() const override
Return the number of columns (values/labels/etc per item).
Definition: SparseTimeValueModel.h:230
EventVector getEventsSpanning(sv_frame_t f, sv_frame_t duration) const
Definition: SparseTimeValueModel.h:161
EventVector getEventsStartingWithin(sv_frame_t frame, sv_frame_t duration) const
Retrieve all events starting within the range in frames defined by the given frame f and duration d...
Definition: EventSeries.cpp:395
void modelChanged(ModelId myId)
Emitted when a model has been edited (or more data retrieved from cache, in the case of a cached mode...
Command * getInsertRowCommand(int row) override
Return a command to insert a new row before the row with the given index.
Definition: SparseTimeValueModel.h:306
Command to add or remove a series of events to or from an editable, with undo.
Definition: EventCommands.h:115
bool isOK() const override
Return true if the model was constructed successfully.
Definition: SparseTimeValueModel.h:90
Generated by
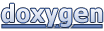