svcore
1.9
|
An immutable(-ish) type used for point and event representation in sparse models, as well as for interchange within the clipboard. More...
#include <Event.h>
Classes | |
struct | ExportNameOptions |
Public Member Functions | |
Event () | |
Event (sv_frame_t frame) | |
Event (sv_frame_t frame, QString label) | |
Event (sv_frame_t frame, float value, QString label) | |
Event (sv_frame_t frame, float value, sv_frame_t duration, QString label) | |
Event (sv_frame_t frame, float value, sv_frame_t duration, float level, QString label) | |
Event (const Event &event)=default | |
Event & | operator= (const Event &event)=default |
Event & | operator= (Event &&event)=default |
sv_frame_t | getFrame () const |
Event | withFrame (sv_frame_t frame) const |
bool | hasValue () const |
float | getValue () const |
Event | withValue (float value) const |
Event | withoutValue () const |
bool | hasDuration () const |
sv_frame_t | getDuration () const |
Event | withDuration (sv_frame_t duration) const |
Event | withoutDuration () const |
bool | hasLabel () const |
QString | getLabel () const |
Event | withLabel (QString label) const |
bool | hasUri () const |
QString | getURI () const |
Event | withURI (QString uri) const |
bool | hasLevel () const |
float | getLevel () const |
Event | withLevel (float level) const |
Event | withoutLevel () const |
bool | hasReferenceFrame () const |
sv_frame_t | getReferenceFrame () const |
bool | referenceFrameDiffers () const |
Event | withReferenceFrame (sv_frame_t frame) const |
Event | withoutReferenceFrame () const |
bool | operator== (const Event &p) const |
bool | operator!= (const Event &p) const |
bool | operator< (const Event &p) const |
void | toXml (QTextStream &stream, QString indent="", QString extraAttributes="", ExportNameOptions opts=ExportNameOptions()) const |
QString | toXmlString (QString indent="", QString extraAttributes="") const |
NoteData | toNoteData (sv_samplerate_t sampleRate, bool valueIsMidiPitch) const |
QVector< QString > | getStringExportHeaders (DataExportOptions opts, ExportNameOptions nameOpts) const |
QVector< QString > | toStringExportRow (DataExportOptions opts, sv_samplerate_t sampleRate) const |
uint | hash (uint seed=0) const |
Private Attributes | |
bool | m_haveValue: 1 |
bool | m_haveLevel: 1 |
bool | m_haveDuration: 1 |
bool | m_haveReferenceFrame: 1 |
float | m_value |
float | m_level |
sv_frame_t | m_frame |
sv_frame_t | m_duration |
sv_frame_t | m_referenceFrame |
QString | m_label |
QString | m_uri |
Detailed Description
An immutable(-ish) type used for point and event representation in sparse models, as well as for interchange within the clipboard.
An event always has a frame and (possibly empty) label, and optionally has numerical value, level, duration in frames, and a mapped reference frame. Event has an operator< defining a total ordering, by frame first and then by the other properties.
Event is based on the Clipboard::Point type up to SV v3.2.1 and is intended also to replace the custom point types previously found in sparse models.
Constructor & Destructor Documentation
|
inline |
|
inline |
|
inline |
|
inline |
Definition at line 82 of file Event.h.
References m_duration, and m_frame.
|
inline |
Definition at line 93 of file Event.h.
References Event(), m_duration, m_frame, and operator=().
|
default |
Member Function Documentation
|
inline |
Definition at line 113 of file Event.h.
References m_frame.
Referenced by EventSeries::add(), TextModel::add(), ImageModel::add(), SparseOneDimensionalModel::add(), BoxModel::add(), RegionModel::add(), SparseTimeValueModel::add(), NoteModel::add(), ImageModel::getData(), TextModel::getData(), SparseOneDimensionalModel::getData(), RegionModel::getData(), BoxModel::getData(), SparseTimeValueModel::getData(), NoteModel::getData(), ImageModel::getFrameForRow(), TextModel::getFrameForRow(), SparseOneDimensionalModel::getFrameForRow(), RegionModel::getFrameForRow(), BoxModel::getFrameForRow(), SparseTimeValueModel::getFrameForRow(), NoteModel::getFrameForRow(), Labeller::getValueFor(), Labeller::label(), EventSeries::remove(), and Labeller::subdivide().
|
inline |
Definition at line 115 of file Event.h.
References m_frame.
Referenced by ImageModel::getSetDataCommand(), TextModel::getSetDataCommand(), SparseOneDimensionalModel::getSetDataCommand(), RegionModel::getSetDataCommand(), BoxModel::getSetDataCommand(), SparseTimeValueModel::getSetDataCommand(), NoteModel::getSetDataCommand(), Labeller::subdivide(), and EventSeries::toStringExportRows().
|
inline |
|
inline |
Definition at line 122 of file Event.h.
References m_haveValue, and m_value.
Referenced by BoxModel::add(), RegionModel::add(), SparseTimeValueModel::add(), NoteModel::add(), TextModel::getData(), RegionModel::getData(), BoxModel::getData(), SparseTimeValueModel::getData(), NoteModel::getData(), BoxModel::getSetDataCommand(), and Labeller::revalue().
|
inline |
Definition at line 124 of file Event.h.
References m_haveValue, and m_value.
Referenced by TextModel::getSetDataCommand(), RegionModel::getSetDataCommand(), BoxModel::getSetDataCommand(), SparseTimeValueModel::getSetDataCommand(), NoteModel::getSetDataCommand(), and Labeller::revalue().
|
inline |
Definition at line 130 of file Event.h.
References m_haveValue, and m_value.
Referenced by ImageModel::add(), and SparseOneDimensionalModel::add().
|
inline |
Definition at line 137 of file Event.h.
References m_haveDuration.
Referenced by EventSeries::add(), and EventSeries::remove().
|
inline |
Definition at line 138 of file Event.h.
References m_duration, and m_haveDuration.
Referenced by EventSeries::add(), BoxModel::add(), RegionModel::add(), NoteModel::add(), RegionModel::getData(), BoxModel::getData(), NoteModel::getData(), and EventSeries::remove().
|
inline |
Definition at line 140 of file Event.h.
References m_duration, m_frame, and m_haveDuration.
Referenced by RegionModel::getSetDataCommand(), BoxModel::getSetDataCommand(), and NoteModel::getSetDataCommand().
|
inline |
Definition at line 150 of file Event.h.
References m_duration, and m_haveDuration.
Referenced by TextModel::add(), ImageModel::add(), SparseOneDimensionalModel::add(), and SparseTimeValueModel::add().
|
inline |
Definition at line 158 of file Event.h.
References m_label.
Referenced by SparseOneDimensionalModel::add(), SparseTimeValueModel::add(), ImageModel::getData(), TextModel::getData(), SparseOneDimensionalModel::getData(), RegionModel::getData(), BoxModel::getData(), SparseTimeValueModel::getData(), NoteModel::getData(), Labeller::getValueFor(), Labeller::label(), and Labeller::subdivide().
|
inline |
Definition at line 160 of file Event.h.
References m_label.
Referenced by ImageModel::getSetDataCommand(), TextModel::getSetDataCommand(), SparseOneDimensionalModel::getSetDataCommand(), RegionModel::getSetDataCommand(), BoxModel::getSetDataCommand(), SparseTimeValueModel::getSetDataCommand(), NoteModel::getSetDataCommand(), Labeller::label(), and Labeller::subdivide().
|
inline |
|
inline |
Definition at line 169 of file Event.h.
References m_uri.
Referenced by ImageModel::getSetDataCommand().
|
inline |
Definition at line 175 of file Event.h.
References m_haveLevel.
|
inline |
Definition at line 176 of file Event.h.
References m_haveLevel, and m_level.
Referenced by BoxModel::add(), BoxModel::getData(), and NoteModel::getData().
|
inline |
Definition at line 178 of file Event.h.
References m_haveLevel, and m_level.
Referenced by BoxModel::getSetDataCommand(), and NoteModel::getSetDataCommand().
|
inline |
Definition at line 184 of file Event.h.
References m_haveLevel, and m_level.
Referenced by TextModel::add(), and ImageModel::add().
|
inline |
Definition at line 191 of file Event.h.
References m_haveReferenceFrame.
|
inline |
Definition at line 192 of file Event.h.
References m_frame, m_haveReferenceFrame, and m_referenceFrame.
|
inline |
Definition at line 196 of file Event.h.
References m_frame, m_haveReferenceFrame, and m_referenceFrame.
|
inline |
Definition at line 200 of file Event.h.
References m_haveReferenceFrame, and m_referenceFrame.
|
inline |
Definition at line 206 of file Event.h.
References m_haveReferenceFrame, and m_referenceFrame.
|
inline |
Definition at line 213 of file Event.h.
References m_duration, m_frame, m_haveDuration, m_haveLevel, m_haveReferenceFrame, m_haveValue, m_label, m_level, m_referenceFrame, m_uri, and m_value.
Referenced by operator!=().
|
inline |
Definition at line 236 of file Event.h.
References operator==().
|
inline |
Definition at line 240 of file Event.h.
References m_duration, m_frame, m_haveDuration, m_haveLevel, m_haveReferenceFrame, m_haveValue, m_label, m_level, m_referenceFrame, m_uri, and m_value.
|
inline |
Definition at line 294 of file Event.h.
References XmlExportable::encodeEntities(), m_duration, m_frame, m_haveDuration, m_haveLevel, m_haveReferenceFrame, m_haveValue, m_label, m_level, m_referenceFrame, m_uri, and m_value.
Referenced by toXmlString().
|
inline |
|
inline |
Definition at line 338 of file Event.h.
References NoteData::frequency, Pitch::getPitchForFrequency(), NoteData::isMidiPitchQuantized, m_duration, m_frame, m_haveDuration, m_haveLevel, m_haveValue, m_level, and m_value.
|
inline |
Definition at line 379 of file Event.h.
References DataExportOmitLevel, DataExportWriteTimeInFrames, Event::ExportNameOptions::levelAttributeName, m_haveDuration, m_haveLevel, m_haveValue, m_uri, Event::ExportNameOptions::uriAttributeName, and Event::ExportNameOptions::valueAttributeName.
|
inline |
Definition at line 421 of file Event.h.
References DataExportOmitLevel, DataExportWriteTimeInFrames, RealTime::frame2RealTime(), m_duration, m_frame, m_haveDuration, m_haveLevel, m_haveValue, m_label, m_level, m_uri, m_value, and RealTime::toString().
Referenced by EventSeries::toStringExportRows().
|
inline |
Definition at line 462 of file Event.h.
References m_duration, m_frame, m_haveDuration, m_haveLevel, m_haveReferenceFrame, m_haveValue, m_label, m_level, m_referenceFrame, m_uri, m_value, and qHash().
Referenced by qHash().
Member Data Documentation
|
private |
Definition at line 477 of file Event.h.
Referenced by getStringExportHeaders(), getValue(), hash(), hasValue(), operator<(), operator==(), toNoteData(), toStringExportRow(), toXml(), withoutValue(), and withValue().
|
private |
Definition at line 478 of file Event.h.
Referenced by getLevel(), getStringExportHeaders(), hash(), hasLevel(), operator<(), operator==(), toNoteData(), toStringExportRow(), toXml(), withLevel(), and withoutLevel().
|
private |
Definition at line 479 of file Event.h.
Referenced by getDuration(), getStringExportHeaders(), hasDuration(), hash(), operator<(), operator==(), toNoteData(), toStringExportRow(), toXml(), withDuration(), and withoutDuration().
|
private |
Definition at line 480 of file Event.h.
Referenced by getReferenceFrame(), hash(), hasReferenceFrame(), operator<(), operator==(), referenceFrameDiffers(), toXml(), withoutReferenceFrame(), and withReferenceFrame().
|
private |
Definition at line 481 of file Event.h.
Referenced by getValue(), hash(), operator<(), operator==(), toNoteData(), toStringExportRow(), toXml(), withoutValue(), and withValue().
|
private |
Definition at line 482 of file Event.h.
Referenced by getLevel(), hash(), operator<(), operator==(), toNoteData(), toStringExportRow(), toXml(), withLevel(), and withoutLevel().
|
private |
Definition at line 483 of file Event.h.
Referenced by Event(), getFrame(), getReferenceFrame(), hash(), operator<(), operator==(), referenceFrameDiffers(), toNoteData(), toStringExportRow(), toXml(), withDuration(), and withFrame().
|
private |
Definition at line 484 of file Event.h.
Referenced by Event(), getDuration(), hash(), operator<(), operator==(), toNoteData(), toStringExportRow(), toXml(), withDuration(), and withoutDuration().
|
private |
Definition at line 485 of file Event.h.
Referenced by getReferenceFrame(), hash(), operator<(), operator==(), referenceFrameDiffers(), toXml(), withoutReferenceFrame(), and withReferenceFrame().
|
private |
Definition at line 486 of file Event.h.
Referenced by getLabel(), hash(), hasLabel(), operator<(), operator==(), toStringExportRow(), toXml(), and withLabel().
|
private |
Definition at line 487 of file Event.h.
Referenced by getStringExportHeaders(), getURI(), hash(), hasUri(), operator<(), operator==(), toStringExportRow(), toXml(), and withURI().
The documentation for this class was generated from the following file:
Generated by
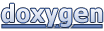