svcore
1.9
|
Event.h
Go to the documentation of this file.
Event(sv_frame_t frame, float value, sv_frame_t duration, float level, QString label)
Definition: Event.h:93
Event & operator=(const Event &event)=default
Note record used when constructing synthetic events for sonification.
Definition: NoteData.h:25
QString toXmlString(QString indent="", QString extraAttributes="") const
Definition: Event.h:329
static RealTime frame2RealTime(sv_frame_t frame, sv_samplerate_t sampleRate)
Convert a sample frame at the given sample rate into a RealTime.
Definition: RealTimeSV.cpp:498
QVector< QString > getStringExportHeaders(DataExportOptions opts, ExportNameOptions nameOpts) const
Definition: Event.h:379
Omit the level attribute from exported events.
Definition: DataExportOptions.h:33
std::string toString(bool align=false) const
Return a human-readable debug-type string to full precision (probably not a format to show to a user ...
Definition: RealTimeSV.cpp:213
QVector< QString > toStringExportRow(DataExportOptions opts, sv_samplerate_t sampleRate) const
Definition: Event.h:421
Definition: Event.h:282
void toXml(QTextStream &stream, QString indent="", QString extraAttributes="", ExportNameOptions opts=ExportNameOptions()) const
Definition: Event.h:294
Event(sv_frame_t frame, float value, sv_frame_t duration, QString label)
Definition: Event.h:82
An immutable(-ish) type used for point and event representation in sparse models, as well as for inte...
Definition: Event.h:55
NoteData toNoteData(sv_samplerate_t sampleRate, bool valueIsMidiPitch) const
Definition: Event.h:338
static int getPitchForFrequency(double frequency, double *centsOffsetReturn=0, double concertA=0.0)
Return the nearest MIDI pitch to the given frequency.
Definition: Pitch.cpp:35
Use sample frames rather than seconds for time and duration values.
Definition: DataExportOptions.h:45
Generated by
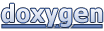