svcore
1.9
|
#include <Pitch.h>
Static Public Member Functions | |
static double | getFrequencyForPitch (int midiPitch, double centsOffset=0, double concertA=0.0) |
Return the frequency at the given MIDI pitch plus centsOffset cents (1/100ths of a semitone). More... | |
static int | getPitchForFrequency (double frequency, double *centsOffsetReturn=0, double concertA=0.0) |
Return the nearest MIDI pitch to the given frequency. More... | |
static int | getPitchForFrequency (double frequency, float *centsOffsetReturn, double concertA=0.0) |
Compatibility version of getPitchForFrequency accepting float pointer argument. More... | |
static int | getPitchForFrequencyDifference (double frequencyA, double frequencyB, double *centsOffsetReturn=0, double concertA=0.0) |
Return the nearest MIDI pitch range to the given frequency range, that is, the difference in MIDI pitch values between the higher and lower frequencies. More... | |
static int | getPitchForFrequencyDifference (double frequencyA, double frequencyB, float *centsOffsetReturn, double concertA=0.0) |
Compatibility version of getPitchForFrequencyDifference accepting float pointer argument. More... | |
static int | getPitchForNoteAndOctave (int note, int octave) |
Return the MIDI pitch for the given note number (0-12 where 0 is C) and octave number. More... | |
static void | getNoteAndOctaveForPitch (int midiPitch, int ¬e, int &octave) |
Return the note number (0-12 where 0 is C) and octave number for the given MIDI pitch. More... | |
static QString | getPitchLabel (int midiPitch, double centsOffset=0, bool useFlats=false) |
Return a string describing the given MIDI pitch, with optional cents offset. More... | |
static QString | getPitchLabelForFrequency (double frequency, double concertA=0.0, bool useFlats=false) |
Return a string describing the nearest MIDI pitch to the given frequency, with cents offset. More... | |
static QString | getLabelForPitchRange (int semis, double cents=0) |
Return a string describing the given pitch range in octaves, semitones and cents. More... | |
static bool | isFrequencyInMidiRange (double frequency, double concertA=0.0) |
Return true if the given frequency falls within the range of MIDI note pitches, plus or minus half a semitone. More... | |
Detailed Description
Member Function Documentation
|
static |
Return the frequency at the given MIDI pitch plus centsOffset cents (1/100ths of a semitone).
centsOffset does not have to be in any particular range or sign.
If concertA is non-zero, use that as the reference frequency for the A at MIDI pitch 69; otherwise use the tuning frequency specified in the application preferences (default 440Hz).
Definition at line 23 of file Pitch.cpp.
References Preferences::getInstance(), and Preferences::getTuningFrequency().
Referenced by NoteData::getFrequency(), and FFTModel::getPeakPickWindowSize().
|
static |
Return the nearest MIDI pitch to the given frequency.
If centsOffsetReturn is non-NULL, return in *centsOffsetReturn the number of cents (1/100ths of a semitone) difference between the given frequency and that of the returned MIDI pitch. The cents offset will be in the range [-50,50).
If concertA is non-zero, use that as the reference frequency for the A at MIDI pitch 69; otherwise use the tuning frequency specified in the application preferences (default 440Hz).
Definition at line 35 of file Pitch.cpp.
References Preferences::getInstance(), and Preferences::getTuningFrequency().
Referenced by getPitchForFrequency(), getPitchLabelForFrequency(), isFrequencyInMidiRange(), and Event::toNoteData().
|
inlinestatic |
Compatibility version of getPitchForFrequency accepting float pointer argument.
Definition at line 57 of file Pitch.h.
References getPitchForFrequency(), and getPitchForFrequencyDifference().
|
static |
Return the nearest MIDI pitch range to the given frequency range, that is, the difference in MIDI pitch values between the higher and lower frequencies.
If centsOffsetReturn is non-NULL, return in *centsOffsetReturn the number of cents (1/100ths of a semitone) difference between the given frequency difference and the returned MIDI pitch range. The cents offset will be in the range [-50,50).
If concertA is non-zero, use that as the reference frequency for the A at MIDI pitch 69; otherwise use the tuning frequency specified in the application preferences (default 440Hz).
Definition at line 61 of file Pitch.cpp.
References Preferences::getInstance(), and Preferences::getTuningFrequency().
Referenced by getPitchForFrequency(), and getPitchForFrequencyDifference().
|
inlinestatic |
Compatibility version of getPitchForFrequencyDifference accepting float pointer argument.
Definition at line 89 of file Pitch.h.
References getLabelForPitchRange(), getNoteAndOctaveForPitch(), getPitchForFrequencyDifference(), getPitchForNoteAndOctave(), getPitchLabel(), getPitchLabelForFrequency(), and isFrequencyInMidiRange().
|
static |
Return the MIDI pitch for the given note number (0-12 where 0 is C) and octave number.
The octave numbering system is based on the application preferences (default is C4 = middle C, though in previous SV releases that was C3).
Definition at line 104 of file Pitch.cpp.
References Preferences::getInstance(), and Preferences::getOctaveOfLowestMIDINote().
Referenced by getPitchForFrequencyDifference().
|
static |
Return the note number (0-12 where 0 is C) and octave number for the given MIDI pitch.
The octave numbering system is based on the application preferences (default is C4 = middle C, though in previous SV releases that was C3).
Definition at line 111 of file Pitch.cpp.
References Preferences::getInstance(), and Preferences::getOctaveOfLowestMIDINote().
Referenced by getPitchForFrequencyDifference(), and getPitchLabel().
|
static |
Return a string describing the given MIDI pitch, with optional cents offset.
This consists of the note name, octave number, and optional cents. The octave numbering system is based on the application preferences (default is C4 = middle C, though in previous SV releases that was C3).
For example, "A#3" (A# in octave 3) or "C2-12c" (C in octave 2, minus 12 cents).
If useFlats is true, spell notes with flats instead of sharps, e.g. Bb3 instead of A#3.
Definition at line 135 of file Pitch.cpp.
References flatNotes, getNoteAndOctaveForPitch(), and notes.
Referenced by getPitchForFrequencyDifference(), getPitchLabelForFrequency(), and MIDIFileReader::loadTrack().
|
static |
Return a string describing the nearest MIDI pitch to the given frequency, with cents offset.
If concertA is non-zero, use that as the reference frequency for the A at MIDI pitch 69; otherwise use the tuning frequency specified in the application preferences (default 440Hz).
If useFlats is true, spell notes with flats instead of sharps, e.g. Bb3 instead of A#3.
Definition at line 151 of file Pitch.cpp.
References Preferences::getInstance(), getPitchForFrequency(), getPitchLabel(), and Preferences::getTuningFrequency().
Referenced by getPitchForFrequencyDifference().
|
static |
Return a string describing the given pitch range in octaves, semitones and cents.
This is in the form e.g. "1'2+4c".
Definition at line 164 of file Pitch.cpp.
Referenced by getPitchForFrequencyDifference().
|
static |
Return true if the given frequency falls within the range of MIDI note pitches, plus or minus half a semitone.
This is equivalent to testing whether getPitchForFrequency returns a pitch in the MIDI range (0 to 127 inclusive) with any cents offset.
If concertA is non-zero, use that as the reference frequency for the A at MIDI pitch 69; otherwise use the tuning frequency specified in the application preferences (default 440Hz).
Definition at line 205 of file Pitch.cpp.
References getPitchForFrequency().
Referenced by getPitchForFrequencyDifference().
The documentation for this class was generated from the following files:
Generated by
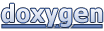