svcore
1.9
|
Preferences.h
Go to the documentation of this file.
Definition: Preferences.h:57
sv_samplerate_t getFixedSampleRate() const
If we should always resample audio to the same rate, return it; otherwise (the normal case) return 0...
Definition: Preferences.h:73
Definition: Preferences.h:97
SpectrogramSmoothing m_spectrogramSmoothing
Definition: Preferences.h:156
void setFixedSampleRate(sv_samplerate_t)
Definition: Preferences.cpp:603
int getOctaveOfLowestMIDINote() const
Definition: Preferences.h:108
PropertyList getProperties() const override
Get a list of the names of all the supported properties on this container.
Definition: Preferences.cpp:95
QString getPropertyValueLabel(const PropertyName &, int value) const override
If the given property is a ValueProperty, return the display label to be used for the given value for...
Definition: Preferences.cpp:334
SpectrogramXSmoothing getSpectrogramXSmoothing() const
Definition: Preferences.h:49
bool getResampleOnLoad() const
True if we should resample second or subsequent audio file to match first audio file's rate...
Definition: Preferences.h:76
int getPropertyRangeAndValue(const PropertyName &, int *, int *, int *) const override
Return the minimum and maximum values for the given property and its current value in this container...
Definition: Preferences.cpp:248
void setSpectrogramXSmoothing(SpectrogramXSmoothing smoothing)
Definition: Preferences.cpp:466
QString getPropertyContainerIconName() const override
Definition: Preferences.cpp:410
Definition: Preferences.h:98
Definition: Preferences.h:93
PropertyBoxLayout getPropertyBoxLayout() const
Definition: Preferences.h:60
void setTimeToTextMode(TimeToTextMode mode)
Definition: Preferences.cpp:644
SpectrogramXSmoothing m_spectrogramXSmoothing
Definition: Preferences.h:157
static int getOctaveOfMiddleCInSystem(OctaveNumberingSystem s)
Definition: Preferences.cpp:689
void setSpectrogramSmoothing(SpectrogramSmoothing smoothing)
Definition: Preferences.cpp:449
QString getTemporaryDirectoryRoot() const
Definition: Preferences.h:66
Definition: Preferences.h:92
bool getNormaliseAudio() const
True if audio files should be loaded with normalisation (max == 1)
Definition: Preferences.h:82
void setOmitTempsFromRecentFiles(bool omit)
Definition: Preferences.cpp:535
bool getOmitTempsFromRecentFiles() const
Definition: Preferences.h:64
bool getRecordMono() const
True if we should always mix down recorded audio to a single channel regardless of how many channels ...
Definition: Preferences.h:70
Definition: Preferences.h:150
void setProperty(const PropertyName &, int) override
Definition: Preferences.cpp:416
SpectrogramSmoothing getSpectrogramSmoothing() const
Definition: Preferences.h:48
Definition: Preferences.h:94
void setPropertyBoxLayout(PropertyBoxLayout layout)
Definition: Preferences.cpp:496
void setTemporaryDirectoryRoot(QString tempDirRoot)
Definition: Preferences.cpp:548
QString getPropertyContainerName() const override
Definition: Preferences.cpp:404
PropertyType getPropertyType(const PropertyName &) const override
Return the type of the given property, or InvalidProperty if the property is not supported on this co...
Definition: Preferences.cpp:184
static OctaveNumberingSystem getSystemWithMiddleCInOctave(int o)
Definition: Preferences.cpp:701
Definition: PropertyContainer.h:29
Definition: Preferences.h:149
Definition: Preferences.h:148
Definition: Preferences.h:85
Definition: Preferences.h:87
Definition: Preferences.h:86
std::vector< PropertyName > PropertyList
Definition: PropertyContainer.h:37
Definition: Preferences.h:23
void setRunPluginsInProcess(bool r)
Definition: Preferences.cpp:522
QString getPropertyLabel(const PropertyName &) const override
Return the human-readable (and i18n'ised) name of a property.
Definition: Preferences.cpp:121
Definition: Preferences.h:96
void setBackgroundMode(BackgroundMode mode)
Definition: Preferences.cpp:629
Definition: Preferences.h:151
Definition: Preferences.h:58
TimeToTextMode getTimeToTextMode() const
Definition: Preferences.h:100
bool getUseGaplessMode() const
True if mp3 files should be loaded "gaplessly", i.e. compensating for encoder/decoder delay and paddi...
Definition: Preferences.h:79
Definition: Preferences.h:95
Generated by
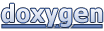