svcore
1.9
|
#include <Preferences.h>
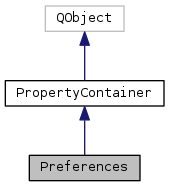
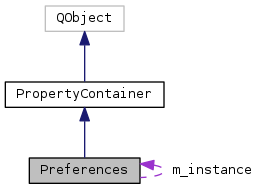
Public Types | |
enum | SpectrogramSmoothing { NoSpectrogramSmoothing, SpectrogramInterpolated } |
enum | SpectrogramXSmoothing { NoSpectrogramXSmoothing, SpectrogramXInterpolated } |
enum | PropertyBoxLayout { VerticallyStacked, Layered } |
!! harmonise with PaneStack More... | |
enum | BackgroundMode { BackgroundFromTheme, DarkBackground, LightBackground } |
enum | TimeToTextMode { TimeToTextMs, TimeToTextUs, TimeToText24Frame, TimeToText25Frame, TimeToText30Frame, TimeToText50Frame, TimeToText60Frame } |
enum | PropertyType { ToggleProperty, RangeProperty, ValueProperty, ColourProperty, ColourMapProperty, UnitsProperty, InvalidProperty } |
typedef QString | PropertyName |
typedef std::vector< PropertyName > | PropertyList |
Public Slots | |
void | setProperty (const PropertyName &, int) override |
void | setSpectrogramSmoothing (SpectrogramSmoothing smoothing) |
void | setSpectrogramXSmoothing (SpectrogramXSmoothing smoothing) |
void | setTuningFrequency (double freq) |
void | setPropertyBoxLayout (PropertyBoxLayout layout) |
void | setWindowType (WindowType type) |
void | setRunPluginsInProcess (bool r) |
void | setOmitTempsFromRecentFiles (bool omit) |
void | setTemporaryDirectoryRoot (QString tempDirRoot) |
void | setFixedSampleRate (sv_samplerate_t) |
void | setRecordMono (bool) |
void | setResampleOnLoad (bool) |
void | setUseGaplessMode (bool) |
void | setNormaliseAudio (bool) |
void | setBackgroundMode (BackgroundMode mode) |
void | setTimeToTextMode (TimeToTextMode mode) |
void | setShowHMS (bool show) |
void | setOctaveOfMiddleC (int oct) |
void | setViewFontSize (int size) |
void | setShowSplash (bool) |
virtual Command * | getSetPropertyCommand (const PropertyName &, int value) |
Obtain a command that sets the given property, which can be added to the command history for undo/redo. More... | |
virtual Command * | getSetPropertyCommand (QString nameString, QString valueString) |
As above, but returning a command. More... | |
virtual void | setPropertyFuzzy (QString nameString, QString valueString) |
Set a property using a fuzzy match. More... | |
Signals | |
void | propertyChanged (PropertyContainer::PropertyName) |
Public Member Functions | |
PropertyList | getProperties () const override |
Get a list of the names of all the supported properties on this container. More... | |
QString | getPropertyLabel (const PropertyName &) const override |
Return the human-readable (and i18n'ised) name of a property. More... | |
PropertyType | getPropertyType (const PropertyName &) const override |
Return the type of the given property, or InvalidProperty if the property is not supported on this container. More... | |
int | getPropertyRangeAndValue (const PropertyName &, int *, int *, int *) const override |
Return the minimum and maximum values for the given property and its current value in this container. More... | |
QString | getPropertyValueLabel (const PropertyName &, int value) const override |
If the given property is a ValueProperty, return the display label to be used for the given value for that property. More... | |
QString | getPropertyContainerName () const override |
QString | getPropertyContainerIconName () const override |
SpectrogramSmoothing | getSpectrogramSmoothing () const |
SpectrogramXSmoothing | getSpectrogramXSmoothing () const |
double | getTuningFrequency () const |
WindowType | getWindowType () const |
bool | getRunPluginsInProcess () const |
PropertyBoxLayout | getPropertyBoxLayout () const |
int | getViewFontSize () const |
bool | getOmitTempsFromRecentFiles () const |
QString | getTemporaryDirectoryRoot () const |
bool | getRecordMono () const |
True if we should always mix down recorded audio to a single channel regardless of how many channels the device opens. More... | |
sv_samplerate_t | getFixedSampleRate () const |
If we should always resample audio to the same rate, return it; otherwise (the normal case) return 0. More... | |
bool | getResampleOnLoad () const |
True if we should resample second or subsequent audio file to match first audio file's rate. More... | |
bool | getUseGaplessMode () const |
True if mp3 files should be loaded "gaplessly", i.e. compensating for encoder/decoder delay and padding. More... | |
bool | getNormaliseAudio () const |
True if audio files should be loaded with normalisation (max == 1) More... | |
BackgroundMode | getBackgroundMode () const |
TimeToTextMode | getTimeToTextMode () const |
bool | getShowHMS () const |
int | getOctaveOfMiddleC () const |
int | getOctaveOfLowestMIDINote () const |
bool | getShowSplash () const |
virtual QString | getPropertyIconName (const PropertyName &) const |
Return an icon for the property, if any. More... | |
virtual QString | getPropertyGroupName (const PropertyName &) const |
If this property has something in common with other properties on this container, return a name that can be used to group them (in order to save screen space, for example). More... | |
virtual QString | getPropertyValueIconName (const PropertyName &, int value) const |
If the given property is a ValueProperty, return the icon to be used for the given value for that property, if any. More... | |
virtual RangeMapper * | getNewPropertyRangeMapper (const PropertyName &) const |
If the given property is a RangeProperty, return a new RangeMapper object mapping its integer range onto an underlying floating point value range for human-intelligible display, if appropriate. More... | |
virtual std::shared_ptr< PlayParameters > | getPlayParameters () |
Return the play parameters for this layer, if any. More... | |
Static Public Member Functions | |
static Preferences * | getInstance () |
Protected Member Functions | |
virtual bool | convertPropertyStrings (QString nameString, QString valueString, PropertyName &name, int &value) |
Private Types | |
enum | OctaveNumberingSystem { C0_Centre, C3_Logic, C4_ASA, C5_Sonar } |
Private Member Functions | |
Preferences () | |
virtual | ~Preferences () |
Static Private Member Functions | |
static int | getOctaveOfMiddleCInSystem (OctaveNumberingSystem s) |
static OctaveNumberingSystem | getSystemWithMiddleCInOctave (int o) |
Private Attributes | |
SpectrogramSmoothing | m_spectrogramSmoothing |
SpectrogramXSmoothing | m_spectrogramXSmoothing |
double | m_tuningFrequency |
PropertyBoxLayout | m_propertyBoxLayout |
WindowType | m_windowType |
bool | m_runPluginsInProcess |
bool | m_omitRecentTemps |
QString | m_tempDirRoot |
sv_samplerate_t | m_fixedSampleRate |
bool | m_recordMono |
bool | m_resampleOnLoad |
bool | m_gapless |
bool | m_normaliseAudio |
int | m_viewFontSize |
BackgroundMode | m_backgroundMode |
TimeToTextMode | m_timeToTextMode |
bool | m_showHMS |
int | m_octave |
bool | m_showSplash |
Static Private Attributes | |
static Preferences * | m_instance = nullptr |
Detailed Description
Definition at line 23 of file Preferences.h.
Member Typedef Documentation
|
inherited |
Definition at line 36 of file PropertyContainer.h.
|
inherited |
Definition at line 37 of file PropertyContainer.h.
Member Enumeration Documentation
Enumerator | |
---|---|
NoSpectrogramSmoothing | |
SpectrogramInterpolated |
Definition at line 38 of file Preferences.h.
Enumerator | |
---|---|
NoSpectrogramXSmoothing | |
SpectrogramXInterpolated |
Definition at line 43 of file Preferences.h.
!! harmonise with PaneStack
Enumerator | |
---|---|
VerticallyStacked | |
Layered |
Definition at line 56 of file Preferences.h.
Enumerator | |
---|---|
BackgroundFromTheme | |
DarkBackground | |
LightBackground |
Definition at line 84 of file Preferences.h.
Enumerator | |
---|---|
TimeToTextMs | |
TimeToTextUs | |
TimeToText24Frame | |
TimeToText25Frame | |
TimeToText30Frame | |
TimeToText50Frame | |
TimeToText60Frame |
Definition at line 91 of file Preferences.h.
|
private |
Enumerator | |
---|---|
C0_Centre | |
C3_Logic | |
C4_ASA | |
C5_Sonar |
Definition at line 147 of file Preferences.h.
|
inherited |
Enumerator | |
---|---|
ToggleProperty | |
RangeProperty | |
ValueProperty | |
ColourProperty | |
ColourMapProperty | |
UnitsProperty | |
InvalidProperty |
Definition at line 39 of file PropertyContainer.h.
Constructor & Destructor Documentation
|
private |
Definition at line 37 of file Preferences.cpp.
References BackgroundFromTheme, HanningWindow, m_backgroundMode, m_fixedSampleRate, m_gapless, m_normaliseAudio, m_octave, m_propertyBoxLayout, m_recordMono, m_resampleOnLoad, m_runPluginsInProcess, m_showHMS, m_showSplash, m_spectrogramSmoothing, m_spectrogramXSmoothing, m_tempDirRoot, m_timeToTextMode, m_tuningFrequency, m_viewFontSize, m_windowType, TimeToTextMs, and VerticallyStacked.
Referenced by getInstance(), and getShowSplash().
|
privatevirtual |
Definition at line 90 of file Preferences.cpp.
Referenced by getShowSplash().
Member Function Documentation
|
static |
Definition at line 31 of file Preferences.cpp.
References m_instance, and Preferences().
Referenced by RecentFiles::addFile(), Pitch::getFrequencyForPitch(), Pitch::getNoteAndOctaveForPitch(), Pitch::getPitchForFrequency(), Pitch::getPitchForFrequencyDifference(), Pitch::getPitchForNoteAndOctave(), Pitch::getPitchLabelForFrequency(), LADSPAPluginFactory::getPortDefault(), PluginPathSetter::getSupportedKeys(), FeatureExtractionPluginFactory::instance(), ReadOnlyWaveFileModel::ReadOnlyWaveFileModel(), PluginScan::scan(), and RealTime::toText().
|
overridevirtual |
Get a list of the names of all the supported properties on this container.
These should be fixed (i.e. not internationalized).
Reimplemented from PropertyContainer.
Definition at line 95 of file Preferences.cpp.
|
overridevirtual |
Return the human-readable (and i18n'ised) name of a property.
Implements PropertyContainer.
Definition at line 121 of file Preferences.cpp.
|
overridevirtual |
Return the type of the given property, or InvalidProperty if the property is not supported on this container.
Reimplemented from PropertyContainer.
Definition at line 184 of file Preferences.cpp.
References PropertyContainer::InvalidProperty, PropertyContainer::RangeProperty, PropertyContainer::ToggleProperty, and PropertyContainer::ValueProperty.
|
overridevirtual |
Return the minimum and maximum values for the given property and its current value in this container.
Min and/or max may be passed as NULL if their values are not required.
!! freq mapping
Reimplemented from PropertyContainer.
Definition at line 248 of file Preferences.cpp.
References BlackmanHarrisWindow, getSystemWithMiddleCInOctave(), HanningWindow, Layered, m_backgroundMode, m_octave, m_omitRecentTemps, m_propertyBoxLayout, m_runPluginsInProcess, m_showHMS, m_showSplash, m_spectrogramSmoothing, m_spectrogramXSmoothing, m_timeToTextMode, m_viewFontSize, m_windowType, RectangularWindow, SpectrogramInterpolated, and SpectrogramXInterpolated.
|
overridevirtual |
If the given property is a ValueProperty, return the display label to be used for the given value for that property.
Reimplemented from PropertyContainer.
Definition at line 334 of file Preferences.cpp.
References BackgroundFromTheme, BartlettWindow, BlackmanHarrisWindow, BlackmanWindow, C0_Centre, C3_Logic, C4_ASA, C5_Sonar, DarkBackground, GaussianWindow, HammingWindow, HanningWindow, LightBackground, NoSpectrogramSmoothing, NoSpectrogramXSmoothing, NuttallWindow, ParzenWindow, RectangularWindow, SpectrogramInterpolated, SpectrogramXInterpolated, TimeToText24Frame, TimeToText25Frame, TimeToText30Frame, TimeToText50Frame, TimeToText60Frame, TimeToTextMs, and TimeToTextUs.
|
overridevirtual |
Implements PropertyContainer.
Definition at line 404 of file Preferences.cpp.
|
overridevirtual |
Implements PropertyContainer.
Definition at line 410 of file Preferences.cpp.
|
inline |
Definition at line 48 of file Preferences.h.
References m_spectrogramSmoothing.
|
inline |
Definition at line 49 of file Preferences.h.
References m_spectrogramXSmoothing.
|
inline |
Definition at line 50 of file Preferences.h.
References m_tuningFrequency.
Referenced by Pitch::getFrequencyForPitch(), Pitch::getPitchForFrequency(), Pitch::getPitchForFrequencyDifference(), Pitch::getPitchLabelForFrequency(), and LADSPAPluginFactory::getPortDefault().
|
inline |
Definition at line 51 of file Preferences.h.
References m_windowType.
|
inline |
Definition at line 53 of file Preferences.h.
References m_runPluginsInProcess.
Referenced by PluginPathSetter::getSupportedKeys(), and PluginScan::scan().
|
inline |
Definition at line 60 of file Preferences.h.
References m_propertyBoxLayout.
|
inline |
Definition at line 62 of file Preferences.h.
References m_viewFontSize.
|
inline |
Definition at line 64 of file Preferences.h.
References m_omitRecentTemps.
Referenced by RecentFiles::addFile().
|
inline |
Definition at line 66 of file Preferences.h.
References m_tempDirRoot.
|
inline |
True if we should always mix down recorded audio to a single channel regardless of how many channels the device opens.
Definition at line 70 of file Preferences.h.
References m_recordMono.
|
inline |
If we should always resample audio to the same rate, return it; otherwise (the normal case) return 0.
Definition at line 73 of file Preferences.h.
References m_fixedSampleRate.
|
inline |
True if we should resample second or subsequent audio file to match first audio file's rate.
Definition at line 76 of file Preferences.h.
References m_resampleOnLoad.
|
inline |
True if mp3 files should be loaded "gaplessly", i.e. compensating for encoder/decoder delay and padding.
Definition at line 79 of file Preferences.h.
References m_gapless.
Referenced by ReadOnlyWaveFileModel::ReadOnlyWaveFileModel().
|
inline |
True if audio files should be loaded with normalisation (max == 1)
Definition at line 82 of file Preferences.h.
References m_normaliseAudio.
Referenced by ReadOnlyWaveFileModel::ReadOnlyWaveFileModel().
|
inline |
Definition at line 89 of file Preferences.h.
References m_backgroundMode.
|
inline |
Definition at line 100 of file Preferences.h.
References m_timeToTextMode.
Referenced by RealTime::toText().
|
inline |
Definition at line 102 of file Preferences.h.
References m_showHMS.
Referenced by RealTime::toText().
|
inline |
Definition at line 104 of file Preferences.h.
References getOctaveOfMiddleCInSystem(), getSystemWithMiddleCInOctave(), and m_octave.
Referenced by getOctaveOfLowestMIDINote().
|
inline |
Definition at line 108 of file Preferences.h.
References getOctaveOfMiddleC().
Referenced by Pitch::getNoteAndOctaveForPitch(), and Pitch::getPitchForNoteAndOctave().
|
inline |
Definition at line 112 of file Preferences.h.
References m_showSplash, Preferences(), setBackgroundMode(), setFixedSampleRate(), setNormaliseAudio(), setOctaveOfMiddleC(), setOmitTempsFromRecentFiles(), setProperty(), setPropertyBoxLayout(), setRecordMono(), setResampleOnLoad(), setRunPluginsInProcess(), setShowHMS(), setShowSplash(), setSpectrogramSmoothing(), setSpectrogramXSmoothing(), setTemporaryDirectoryRoot(), setTimeToTextMode(), setTuningFrequency(), setUseGaplessMode(), setViewFontSize(), setWindowType(), and ~Preferences().
|
overrideslot |
!!
Definition at line 416 of file Preferences.cpp.
References getOctaveOfMiddleCInSystem(), Layered, setBackgroundMode(), setOctaveOfMiddleC(), setOmitTempsFromRecentFiles(), setPropertyBoxLayout(), setRunPluginsInProcess(), setShowHMS(), setShowSplash(), setSpectrogramSmoothing(), setSpectrogramXSmoothing(), setTimeToTextMode(), setViewFontSize(), setWindowType(), and VerticallyStacked.
Referenced by getShowSplash().
|
slot |
Definition at line 449 of file Preferences.cpp.
References m_spectrogramSmoothing, and PropertyContainer::propertyChanged().
Referenced by getShowSplash(), and setProperty().
|
slot |
Definition at line 466 of file Preferences.cpp.
References m_spectrogramXSmoothing, and PropertyContainer::propertyChanged().
Referenced by getShowSplash(), and setProperty().
|
slot |
Definition at line 483 of file Preferences.cpp.
References m_tuningFrequency, and PropertyContainer::propertyChanged().
Referenced by getShowSplash().
|
slot |
Definition at line 496 of file Preferences.cpp.
References m_propertyBoxLayout, and PropertyContainer::propertyChanged().
Referenced by getShowSplash(), and setProperty().
|
slot |
Definition at line 509 of file Preferences.cpp.
References m_windowType, and PropertyContainer::propertyChanged().
Referenced by getShowSplash(), and setProperty().
|
slot |
Definition at line 522 of file Preferences.cpp.
References m_runPluginsInProcess, and PropertyContainer::propertyChanged().
Referenced by getShowSplash(), and setProperty().
|
slot |
Definition at line 535 of file Preferences.cpp.
References m_omitRecentTemps, and PropertyContainer::propertyChanged().
Referenced by getShowSplash(), and setProperty().
|
slot |
Definition at line 548 of file Preferences.cpp.
References m_tempDirRoot, and PropertyContainer::propertyChanged().
Referenced by getShowSplash().
|
slot |
Definition at line 603 of file Preferences.cpp.
References m_fixedSampleRate, and PropertyContainer::propertyChanged().
Referenced by getShowSplash().
|
slot |
Definition at line 564 of file Preferences.cpp.
References m_recordMono, and PropertyContainer::propertyChanged().
Referenced by getShowSplash().
|
slot |
Definition at line 577 of file Preferences.cpp.
References m_resampleOnLoad, and PropertyContainer::propertyChanged().
Referenced by getShowSplash().
|
slot |
Definition at line 590 of file Preferences.cpp.
References m_gapless, and PropertyContainer::propertyChanged().
Referenced by getShowSplash().
|
slot |
Definition at line 616 of file Preferences.cpp.
References m_normaliseAudio, and PropertyContainer::propertyChanged().
Referenced by getShowSplash().
|
slot |
Definition at line 629 of file Preferences.cpp.
References m_backgroundMode, and PropertyContainer::propertyChanged().
Referenced by getShowSplash(), and setProperty().
|
slot |
Definition at line 644 of file Preferences.cpp.
References m_timeToTextMode, and PropertyContainer::propertyChanged().
Referenced by getShowSplash(), and setProperty().
|
slot |
Definition at line 659 of file Preferences.cpp.
References m_showHMS, and PropertyContainer::propertyChanged().
Referenced by getShowSplash(), and setProperty().
|
slot |
Definition at line 674 of file Preferences.cpp.
References m_octave, and PropertyContainer::propertyChanged().
Referenced by getShowSplash(), and setProperty().
|
slot |
Definition at line 713 of file Preferences.cpp.
References m_viewFontSize, and PropertyContainer::propertyChanged().
Referenced by getShowSplash(), and setProperty().
|
slot |
Definition at line 728 of file Preferences.cpp.
References m_showSplash, and PropertyContainer::propertyChanged().
Referenced by getShowSplash(), and setProperty().
|
staticprivate |
Definition at line 689 of file Preferences.cpp.
References C0_Centre, C3_Logic, C4_ASA, and C5_Sonar.
Referenced by getOctaveOfMiddleC(), and setProperty().
|
staticprivate |
Definition at line 701 of file Preferences.cpp.
References C0_Centre, C3_Logic, C4_ASA, and C5_Sonar.
Referenced by getOctaveOfMiddleC(), and getPropertyRangeAndValue().
|
virtualinherited |
Return an icon for the property, if any.
Definition at line 35 of file PropertyContainer.cpp.
|
virtualinherited |
If this property has something in common with other properties on this container, return a name that can be used to group them (in order to save screen space, for example).
e.g. "Window Type" and "Window Size" might both have a group name of "Window". If this property is not groupable, return the empty string.
Definition at line 41 of file PropertyContainer.cpp.
|
virtualinherited |
If the given property is a ValueProperty, return the icon to be used for the given value for that property, if any.
Definition at line 63 of file PropertyContainer.cpp.
|
virtualinherited |
If the given property is a RangeProperty, return a new RangeMapper object mapping its integer range onto an underlying floating point value range for human-intelligible display, if appropriate.
The RangeMapper should be allocated with new, and the caller takes responsibility for deleting it. Return NULL (as in the default implementation) if there is no such mapping.
Definition at line 69 of file PropertyContainer.cpp.
Referenced by PropertyContainer::convertPropertyStrings().
|
inlinevirtualinherited |
Return the play parameters for this layer, if any.
The return value is a shared_ptr that, if not null, can be passed to e.g. PlayParameterRepository::EditCommand to change the parameters.
Definition at line 121 of file PropertyContainer.h.
References PropertyContainer::getSetPropertyCommand(), PropertyContainer::propertyChanged(), PropertyContainer::setProperty(), and PropertyContainer::setPropertyFuzzy().
|
signalinherited |
Referenced by PropertyContainer::getPlayParameters(), setBackgroundMode(), setFixedSampleRate(), setNormaliseAudio(), setOctaveOfMiddleC(), setOmitTempsFromRecentFiles(), setPropertyBoxLayout(), setRecordMono(), setResampleOnLoad(), setRunPluginsInProcess(), setShowHMS(), setShowSplash(), setSpectrogramSmoothing(), setSpectrogramXSmoothing(), setTemporaryDirectoryRoot(), setTimeToTextMode(), setTuningFrequency(), setUseGaplessMode(), setViewFontSize(), and setWindowType().
|
virtualslotinherited |
Obtain a command that sets the given property, which can be added to the command history for undo/redo.
Returns NULL if the property is already set to the given value.
Definition at line 81 of file PropertyContainer.cpp.
References PropertyContainer::getPropertyRangeAndValue().
Referenced by PropertyContainer::getPlayParameters(), and PropertyContainer::getSetPropertyCommand().
|
virtualslotinherited |
As above, but returning a command.
Definition at line 104 of file PropertyContainer.cpp.
References PropertyContainer::convertPropertyStrings(), and PropertyContainer::getSetPropertyCommand().
|
virtualslotinherited |
Set a property using a fuzzy match.
Compare nameString with the property labels and underlying names, and if it matches one (with preference given to labels), try to convert valueString appropriately and set it. The valueString should contain a value label for value properties, a mapped value for range properties, "on" or "off" for toggle properties, a colour or unit name, or the underlying integer value for the property.
Note that as property and value labels may be translatable, the results of this function may vary by locale. It is intended for handling user-originated strings, not persistent storage.
The default implementation should work for most subclasses.
Definition at line 89 of file PropertyContainer.cpp.
References PropertyContainer::convertPropertyStrings(), and PropertyContainer::setProperty().
Referenced by PropertyContainer::getPlayParameters().
|
protectedvirtualinherited |
Definition at line 119 of file PropertyContainer.cpp.
References PropertyContainer::ColourMapProperty, PropertyContainer::ColourProperty, UnitDatabase::getInstance(), PropertyContainer::getNewPropertyRangeMapper(), RangeMapper::getPositionForValue(), PropertyContainer::getProperties(), PropertyContainer::getPropertyLabel(), PropertyContainer::getPropertyRangeAndValue(), PropertyContainer::getPropertyType(), PropertyContainer::getPropertyValueLabel(), UnitDatabase::getUnitId(), PropertyContainer::InvalidProperty, PropertyContainer::RangeProperty, SVDEBUG, PropertyContainer::ToggleProperty, PropertyContainer::UnitsProperty, and PropertyContainer::ValueProperty.
Referenced by PropertyContainer::getSetPropertyCommand(), and PropertyContainer::setPropertyFuzzy().
Member Data Documentation
|
staticprivate |
Definition at line 141 of file Preferences.h.
Referenced by getInstance().
|
private |
Definition at line 156 of file Preferences.h.
Referenced by getPropertyRangeAndValue(), getSpectrogramSmoothing(), Preferences(), and setSpectrogramSmoothing().
|
private |
Definition at line 157 of file Preferences.h.
Referenced by getPropertyRangeAndValue(), getSpectrogramXSmoothing(), Preferences(), and setSpectrogramXSmoothing().
|
private |
Definition at line 158 of file Preferences.h.
Referenced by getTuningFrequency(), Preferences(), and setTuningFrequency().
|
private |
Definition at line 159 of file Preferences.h.
Referenced by getPropertyBoxLayout(), getPropertyRangeAndValue(), Preferences(), and setPropertyBoxLayout().
|
private |
Definition at line 160 of file Preferences.h.
Referenced by getPropertyRangeAndValue(), getWindowType(), Preferences(), and setWindowType().
|
private |
Definition at line 161 of file Preferences.h.
Referenced by getPropertyRangeAndValue(), getRunPluginsInProcess(), Preferences(), and setRunPluginsInProcess().
|
private |
Definition at line 162 of file Preferences.h.
Referenced by getOmitTempsFromRecentFiles(), getPropertyRangeAndValue(), and setOmitTempsFromRecentFiles().
|
private |
Definition at line 163 of file Preferences.h.
Referenced by getTemporaryDirectoryRoot(), Preferences(), and setTemporaryDirectoryRoot().
|
private |
Definition at line 164 of file Preferences.h.
Referenced by getFixedSampleRate(), Preferences(), and setFixedSampleRate().
|
private |
Definition at line 165 of file Preferences.h.
Referenced by getRecordMono(), Preferences(), and setRecordMono().
|
private |
Definition at line 166 of file Preferences.h.
Referenced by getResampleOnLoad(), Preferences(), and setResampleOnLoad().
|
private |
Definition at line 167 of file Preferences.h.
Referenced by getUseGaplessMode(), Preferences(), and setUseGaplessMode().
|
private |
Definition at line 168 of file Preferences.h.
Referenced by getNormaliseAudio(), Preferences(), and setNormaliseAudio().
|
private |
Definition at line 169 of file Preferences.h.
Referenced by getPropertyRangeAndValue(), getViewFontSize(), Preferences(), and setViewFontSize().
|
private |
Definition at line 170 of file Preferences.h.
Referenced by getBackgroundMode(), getPropertyRangeAndValue(), Preferences(), and setBackgroundMode().
|
private |
Definition at line 171 of file Preferences.h.
Referenced by getPropertyRangeAndValue(), getTimeToTextMode(), Preferences(), and setTimeToTextMode().
|
private |
Definition at line 172 of file Preferences.h.
Referenced by getPropertyRangeAndValue(), getShowHMS(), Preferences(), and setShowHMS().
|
private |
Definition at line 173 of file Preferences.h.
Referenced by getOctaveOfMiddleC(), getPropertyRangeAndValue(), Preferences(), and setOctaveOfMiddleC().
|
private |
Definition at line 174 of file Preferences.h.
Referenced by getPropertyRangeAndValue(), getShowSplash(), Preferences(), and setShowSplash().
The documentation for this class was generated from the following files:
Generated by
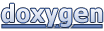