svcore
1.9
|
LADSPAPluginFactory.cpp
Go to the documentation of this file.
84 SVCERR << "WARNING: LADSPAPluginFactory::enumeratePlugins: couldn't get descriptor for identifier " << *i << endl;
98 // SVCERR << "LADSPAPluginFactory: cat for " << *i << " found in taxonomy as " << m_taxonomy[descriptor->UniqueID] << endl;
102 // SVCERR << "LADSPAPluginFactory: cat for " << *i << " not found (despite having " << m_fallbackCategories.size() << " fallbacks)" << endl;
373 SVCERR << "WARNING: LADSPAPluginFactory::getLADSPADescriptor: loadLibrary failed for " << soname << endl;
384 SVCERR << "WARNING: LADSPAPluginFactory::getLADSPADescriptor: No descriptor function in library " << soname << endl;
396 SVCERR << "WARNING: LADSPAPluginFactory::getLADSPADescriptor: No such plugin as " << label << " in library " << soname << endl;
413 SVCERR << "LADSPAPluginFactory::loadLibrary: Library \"" << soName << "\" exists, but failed to load it" << endl;
459 SVCERR << "LADSPAPluginFactory::loadLibrary: Failed to locate plugin library \"" << soName << "\"" << endl;
657 SVCERR << "WARNING: LADSPAPluginFactory::discoverPlugins: No descriptor function in " << soname << endl;
687 // SVCERR << "set id \"" << identifier << "\" to cat \"" << m_taxonomy[identifier] << "\" from LRDF" << endl;
725 // SVCERR << "Default for this port (" << defs->items[j].pid << ", " << defs->items[j].label << ") is " << defs->items[j].value << "; applying this to port number " << i << " with name " << descriptor->PortNames[i] << endl;
768 SVCERR << "WARNING: LADSPAPluginFactory::discoverPlugins - can't unload " << libraryHandle << endl;
788 // SVDEBUG << "LADSPAPluginFactory::generateFallbackCategories: path element " << pluginPath[i] << endl;
795 // SVDEBUG << "LADSPAPluginFactory::generateFallbackCategories: directory " << path[i] << " has " << dir.count() << " .cat files" << endl;
800 // SVDEBUG << "LADSPAPluginFactory::generateFallbackCategories: about to open " << (path[i]+ "/" + dir[j]) << endl;
std::map< QString, QString > m_libraries
Definition: LADSPAPluginFactory.h:91
std::vector< std::string > controlOutputPortNames
Definition: RealTimePluginFactory.h:47
virtual ~LADSPAPluginFactory()
Definition: LADSPAPluginFactory.cpp:52
void unloadLibrary(QString soName)
Definition: LADSPAPluginFactory.cpp:463
int getPortDisplayHint(const LADSPA_Descriptor *, int port)
Definition: LADSPAPluginFactory.cpp:317
static QString canonicalise(QString identifier)
Definition: PluginIdentifier.cpp:35
QList< Candidate > getCandidateLibrariesFor(PluginType) const
Return the candidate plugin libraries of the given type that were found by helpers during the startup...
Definition: PluginScan.cpp:132
QString getPluginLibraryPath(QString identifier) override
Get the full file path (including both directory and filename) of the library file that provides a gi...
Definition: LADSPAPluginFactory.cpp:68
LibraryHandleMap m_libraryHandles
Definition: LADSPAPluginFactory.h:102
bool getEnvUtf8(std::string variable, std::string &value)
Return the value of the given environment variable by reference.
Definition: System.cpp:344
virtual void generateTaxonomy(QString uri, QString base)
Definition: LADSPAPluginFactory.cpp:822
void discoverPlugins() override
Look up the plugin path and find the plugins in it.
Definition: LADSPAPluginFactory.cpp:595
static void parseIdentifier(QString identifier, QString &type, QString &soName, QString &label)
Definition: PluginIdentifier.cpp:43
Definition: LADSPAPluginInstance.h:36
static QString createIdentifier(QString type, QString soName, QString label)
Definition: PluginIdentifier.cpp:26
float getPortDefault(const LADSPA_Descriptor *, int port)
Definition: LADSPAPluginFactory.cpp:199
unsigned int audioInputPortCount
Definition: RealTimePluginFactory.h:44
unsigned int parameterCount
Definition: RealTimePluginFactory.h:43
unsigned int controlOutputPortCount
Definition: RealTimePluginFactory.h:46
static std::vector< QString > getPluginPath()
Definition: LADSPAPluginFactory.cpp:523
std::set< std::weak_ptr< RealTimePluginInstance >, std::owner_less< std::weak_ptr< RealTimePluginInstance > > > m_instances
Definition: LADSPAPluginFactory.h:99
virtual void generateFallbackCategories()
Definition: LADSPAPluginFactory.cpp:774
virtual PluginScan::PluginType getPluginType() const
Definition: LADSPAPluginFactory.h:74
static sv_samplerate_t m_sampleRate
Definition: RealTimePluginFactory.h:112
float getPortMaximum(const LADSPA_Descriptor *, int port)
Definition: LADSPAPluginFactory.cpp:176
static QString BUILTIN_PLUGIN_SONAME
Definition: PluginIdentifier.h:44
void unloadUnusedLibraries()
Definition: LADSPAPluginFactory.cpp:474
float getPortQuantization(const LADSPA_Descriptor *, int port)
Definition: LADSPAPluginFactory.cpp:303
virtual std::vector< QString > getLRDFPath(QString &baseUri)
Definition: LADSPAPluginFactory.cpp:571
std::map< unsigned long, std::map< int, float > > m_portDefaults
Definition: LADSPAPluginFactory.h:96
std::map< QString, RealTimePluginDescriptor > m_rtDescriptors
Definition: LADSPAPluginFactory.h:92
QString getPluginCategory(QString identifier) override
Get category metadata about a plugin (without instantiating it).
Definition: LADSPAPluginFactory.cpp:852
std::map< QString, QString > m_taxonomy
Definition: LADSPAPluginFactory.h:94
void enumeratePlugins(std::vector< QString > &list) override
Append to the given list descriptions of all the available plugins and their ports.
Definition: LADSPAPluginFactory.cpp:74
std::vector< QString > m_identifiers
Definition: LADSPAPluginFactory.h:90
virtual void discoverPluginsFrom(QString soName)
Definition: LADSPAPluginFactory.cpp:643
std::map< unsigned long, QString > m_lrdfTaxonomy
Definition: LADSPAPluginFactory.h:95
static RealTimePluginFactory * instance(QString pluginType)
Definition: RealTimePluginFactory.cpp:43
const std::vector< QString > & getPluginIdentifiers() const override
Return a reference to a list of all plugin identifiers that can be created by this factory...
Definition: LADSPAPluginFactory.cpp:62
void loadLibrary(QString soName)
Definition: LADSPAPluginFactory.cpp:402
RealTimePluginDescriptor getPluginDescriptor(QString identifier) const override
Get some basic information about a plugin (rapidly).
Definition: LADSPAPluginFactory.cpp:136
float getPortMinimum(const LADSPA_Descriptor *, int port)
Definition: LADSPAPluginFactory.cpp:149
virtual const LADSPA_Descriptor * getLADSPADescriptor(QString identifier)
Definition: LADSPAPluginFactory.cpp:365
std::shared_ptr< RealTimePluginInstance > instantiatePlugin(QString identifier, int clientId, int position, sv_samplerate_t sampleRate, int blockSize, int channels) override
Instantiate a plugin.
Definition: LADSPAPluginFactory.cpp:332
unsigned int audioOutputPortCount
Definition: RealTimePluginFactory.h:45
#define PLUGIN_GLOB
Generated by
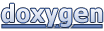