svcore
1.9
|
PluginIdentifier.cpp
Go to the documentation of this file.
static QString canonicalise(QString identifier)
Definition: PluginIdentifier.cpp:35
static QString RESERVED_PROJECT_DIRECTORY_KEY
Definition: PluginIdentifier.h:47
static void parseIdentifier(QString identifier, QString &type, QString &soName, QString &label)
Definition: PluginIdentifier.cpp:43
static QString createIdentifier(QString type, QString soName, QString label)
Definition: PluginIdentifier.cpp:26
static bool areIdentifiersSimilar(QString id1, QString id2)
Definition: PluginIdentifier.cpp:54
static QString BUILTIN_PLUGIN_SONAME
Definition: PluginIdentifier.h:44
Generated by
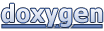