svcore
1.9
|
Profiler.h
Go to the documentation of this file.
void accumulate(const char *id, clock_t time, RealTime rt)
Definition: Profiler.cpp:51
RealTime represents time values to nanosecond precision with accurate arithmetic and frame-rate conve...
Definition: RealTime.h:42
Generated by
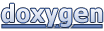