svcore
1.9
|
RealTime represents time values to nanosecond precision with accurate arithmetic and frame-rate conversion functions. More...
#include <RealTime.h>

Public Member Functions | |
int | usec () const |
int | msec () const |
RealTime () | |
RealTime (int s, int n) | |
RealTime (const RealTime &r) | |
RealTime (const Vamp::RealTime &r) | |
double | toDouble () const |
Vamp::RealTime | toVampRealTime () const |
RealTime & | operator= (const RealTime &r) |
RealTime | operator+ (const RealTime &r) const |
RealTime | operator- (const RealTime &r) const |
RealTime | operator- () const |
bool | operator< (const RealTime &r) const |
bool | operator> (const RealTime &r) const |
bool | operator== (const RealTime &r) const |
bool | operator!= (const RealTime &r) const |
bool | operator>= (const RealTime &r) const |
bool | operator<= (const RealTime &r) const |
RealTime | operator* (int m) const |
RealTime | operator/ (int d) const |
RealTime | operator* (double m) const |
RealTime | operator/ (double d) const |
double | operator/ (const RealTime &r) const |
Return the ratio of two times. More... | |
std::string | toString (bool align=false) const |
Return a human-readable debug-type string to full precision (probably not a format to show to a user directly). More... | |
std::string | toText (bool fixedDp=false) const |
Return a user-readable string to the nearest millisecond, typically in a form like HH:MM:SS.mmm. More... | |
std::string | toMSText (bool fixedDp, bool hms) const |
Return a user-readable string to the nearest millisecond. More... | |
std::string | toFrameText (int fps, bool hms, std::string frameDelimiter=":") const |
Return a user-readable string in which seconds are divided into frames (presumably at a lower frame rate than audio rate, e.g. More... | |
std::string | toSecText () const |
Return a user-readable string to the nearest second, in H:M:S form. More... | |
std::string | toXsdDuration () const |
Return a string in xsd:duration format. More... | |
Static Public Member Functions | |
static RealTime | fromSeconds (double sec) |
static RealTime | fromMilliseconds (int64_t msec) |
static RealTime | fromMicroseconds (int64_t usec) |
static RealTime | fromTimeval (const struct timeval &) |
static RealTime | fromXsdDuration (std::string xsdd) |
static RealTime | fromString (std::string) |
Convert a string as obtained from toString back to a RealTime object. More... | |
static sv_frame_t | realTime2Frame (const RealTime &r, sv_samplerate_t sampleRate) |
Convert a RealTime into a sample frame at the given sample rate. More... | |
static RealTime | frame2RealTime (sv_frame_t frame, sv_samplerate_t sampleRate) |
Convert a sample frame at the given sample rate into a RealTime. More... | |
Public Attributes | |
int | sec |
int | nsec |
Static Public Attributes | |
static const RealTime | zeroTime |
Detailed Description
RealTime represents time values to nanosecond precision with accurate arithmetic and frame-rate conversion functions.
Definition at line 42 of file RealTime.h.
Constructor & Destructor Documentation
|
inline |
Definition at line 50 of file RealTime.h.
Referenced by frame2RealTime_i(), fromMicroseconds(), fromMilliseconds(), fromSeconds(), fromString(), fromTimeval(), fromXsdDuration(), operator+(), operator-(), and operator/().
RealTime::RealTime | ( | int | s, |
int | n | ||
) |
Definition at line 44 of file RealTimeSV.cpp.
References nsec, ONE_BILLION, and sec.
|
inline |
Definition at line 53 of file RealTime.h.
|
inline |
Definition at line 56 of file RealTime.h.
References fromMicroseconds(), fromMilliseconds(), fromSeconds(), fromTimeval(), fromXsdDuration(), msec(), toDouble(), and usec().
Member Function Documentation
|
inline |
Definition at line 47 of file RealTime.h.
Referenced by RealTime().
|
inline |
Definition at line 48 of file RealTime.h.
Referenced by RealTime(), and toMSText().
|
static |
Definition at line 54 of file RealTimeSV.cpp.
References fromSeconds(), ONE_BILLION, and RealTime().
Referenced by MIDIFileReader::calculateTempoTimestamps(), frame2RealTime(), fromSeconds(), fromXsdDuration(), MIDIFileReader::getTimeForMIDITime(), operator*(), operator/(), and RealTime().
|
static |
Definition at line 64 of file RealTimeSV.cpp.
References RealTime(), sec, and zeroTime.
Referenced by RealTime().
|
static |
Definition at line 77 of file RealTimeSV.cpp.
References RealTime(), sec, and zeroTime.
Referenced by RealTime().
|
static |
Definition at line 90 of file RealTimeSV.cpp.
References RealTime().
Referenced by Profiler::end(), Profiler::Profiler(), RealTime(), and Profiler::update().
|
static |
Definition at line 96 of file RealTimeSV.cpp.
References fromSeconds(), and RealTime().
Referenced by RDFImporterImpl::getDataModelsSparse(), RDFTransformFactoryImpl::getTransforms(), and RealTime().
double RealTime::toDouble | ( | ) | const |
Definition at line 181 of file RealTimeSV.cpp.
References nsec, ONE_BILLION, and sec.
Referenced by FeatureExtractionModelTransformer::addFeature(), and RealTime().
|
inline |
Definition at line 66 of file RealTime.h.
Definition at line 68 of file RealTime.h.
Definition at line 72 of file RealTime.h.
References nsec, RealTime(), and sec.
Definition at line 75 of file RealTime.h.
References nsec, RealTime(), and sec.
|
inline |
Definition at line 78 of file RealTime.h.
References RealTime().
|
inline |
Definition at line 82 of file RealTime.h.
|
inline |
Definition at line 87 of file RealTime.h.
|
inline |
Definition at line 92 of file RealTime.h.
|
inline |
Definition at line 96 of file RealTime.h.
|
inline |
Definition at line 100 of file RealTime.h.
|
inline |
Definition at line 105 of file RealTime.h.
References frame2RealTime(), fromString(), nsec, operator*(), operator/(), realTime2Frame(), sec, toFrameText(), toMSText(), toSecText(), toString(), toText(), and toXsdDuration().
RealTime RealTime::operator* | ( | int | m | ) | const |
Definition at line 431 of file RealTimeSV.cpp.
References fromSeconds(), nsec, ONE_BILLION, and sec.
Referenced by operator<=().
RealTime RealTime::operator/ | ( | int | d | ) | const |
Definition at line 439 of file RealTimeSV.cpp.
References nsec, ONE_BILLION, RealTime(), and sec.
Referenced by operator<=().
RealTime RealTime::operator* | ( | double | m | ) | const |
Definition at line 450 of file RealTimeSV.cpp.
References fromSeconds(), nsec, ONE_BILLION, and sec.
RealTime RealTime::operator/ | ( | double | d | ) | const |
Definition at line 458 of file RealTimeSV.cpp.
References fromSeconds(), nsec, ONE_BILLION, and sec.
double RealTime::operator/ | ( | const RealTime & | r | ) | const |
Return the ratio of two times.
Definition at line 466 of file RealTimeSV.cpp.
References nsec, ONE_BILLION, and sec.
std::string RealTime::toString | ( | bool | align = false | ) | const |
Return a human-readable debug-type string to full precision (probably not a format to show to a user directly).
If align is true, prepend " " to the start of positive values so that they line up with negative ones (which start with "-").
Definition at line 213 of file RealTimeSV.cpp.
References zeroTime.
Referenced by TabularModel::adaptFrameForRole(), operator<=(), PathPoint::toDelimitedDataString(), Event::toStringExportRow(), Transform::toXml(), toXsdDuration(), and CSVFeatureWriter::writeFeature().
|
static |
Convert a string as obtained from toString back to a RealTime object.
Definition at line 230 of file RealTimeSV.cpp.
References nsec, RealTime(), and sec.
Referenced by operator<=(), and Transform::setFromXmlAttributes().
std::string RealTime::toText | ( | bool | fixedDp = false | ) | const |
Return a user-readable string to the nearest millisecond, typically in a form like HH:MM:SS.mmm.
The exact format will depend on the application preferences for time display precision and hours:minutes:seconds format – this function simply dispatches to toMSText or toFrameText with appropriate arguments depending on the preferences.
If fixedDp is true, the result will be padded to 3 dp, i.e. millisecond resolution, even if the number of milliseconds is a multiple of 10.
Definition at line 274 of file RealTimeSV.cpp.
References Preferences::getInstance(), Preferences::getShowHMS(), Preferences::getTimeToTextMode(), Preferences::TimeToText24Frame, Preferences::TimeToText25Frame, Preferences::TimeToText30Frame, Preferences::TimeToText50Frame, Preferences::TimeToText60Frame, Preferences::TimeToTextMs, Preferences::TimeToTextUs, toFrameText(), toMSText(), and zeroTime.
Referenced by TabularModel::adaptFrameForRole(), DenseThreeDimensionalModel::getData(), and operator<=().
std::string RealTime::toMSText | ( | bool | fixedDp, |
bool | hms | ||
) | const |
Return a user-readable string to the nearest millisecond.
If fixedDp is true, the result will be padded to 3 dp, i.e. millisecond resolution, even if the number of milliseconds is a multiple of 10.
If hms is true, results may be returned in the form HH:MM:SS.mmm (if the time is large enough). If hms is false, the result will always be a (fractional) number of seconds.
Unlike toText, this function does not depend on the application preferences.
Definition at line 332 of file RealTimeSV.cpp.
References msec(), sec, writeSecPart(), and zeroTime.
Referenced by operator<=(), and toText().
std::string RealTime::toFrameText | ( | int | fps, |
bool | hms, | ||
std::string | frameDelimiter = ":" |
||
) | const |
Return a user-readable string in which seconds are divided into frames (presumably at a lower frame rate than audio rate, e.g.
24 or 25 video frames), in a form like HH:MM:SS:FF. fps gives the number of frames per second, and must be integral (29.97 not supported).
Unlike toText, this function does not depend on the application preferences.
Definition at line 367 of file RealTimeSV.cpp.
References nsec, ONE_BILLION, sec, writeSecPart(), and zeroTime.
Referenced by operator<=(), and toText().
std::string RealTime::toSecText | ( | ) | const |
Return a user-readable string to the nearest second, in H:M:S form.
Does not include milliseconds or frames. The result will be suffixed "s" if it contains only seconds (no hours or minutes).
Unlike toText, this function does not depend on the application preferences.
Definition at line 406 of file RealTimeSV.cpp.
References sec, writeSecPart(), and zeroTime.
Referenced by operator<=().
std::string RealTime::toXsdDuration | ( | ) | const |
Return a string in xsd:duration format.
Definition at line 424 of file RealTimeSV.cpp.
References toString().
Referenced by operator<=(), and RDFTransformFactoryImpl::writeTransformToRDF().
|
static |
Convert a RealTime into a sample frame at the given sample rate.
Definition at line 490 of file RealTimeSV.cpp.
References nsec, sec, and zeroTime.
Referenced by FeatureExtractionModelTransformer::addFeature(), RDFImporterImpl::getDataModelsSparse(), MIDIFileReader::loadTrack(), operator<=(), RealTimeEffectModelTransformer::run(), DSSIPluginInstance::run(), FeatureExtractionModelTransformer::run(), DSSIPluginInstance::runGrouped(), RDFFeatureWriter::writeDenseRDF(), and CSVFeatureWriter::writeFeature().
|
static |
Convert a sample frame at the given sample rate into a RealTime.
Definition at line 498 of file RealTimeSV.cpp.
References frame2RealTime_i(), fromSeconds(), sec, and zeroTime.
Referenced by TabularModel::adaptFrameForRole(), DenseThreeDimensionalModel::getData(), MIDIFileReader::loadTrack(), operator<=(), RealTimeEffectModelTransformer::run(), FeatureExtractionModelTransformer::run(), PathPoint::toDelimitedDataString(), Event::toStringExportRow(), BoxModel::toStringExportRows(), and RDFExporter::write().
Member Data Documentation
int RealTime::sec |
Definition at line 44 of file RealTime.h.
Referenced by frame2RealTime(), frame2RealTime_i(), fromMicroseconds(), fromMilliseconds(), fromString(), operator*(), operator+(), operator-(), operator/(), operator<(), operator<<(), operator<=(), operator=(), operator==(), operator>(), operator>=(), RealTime(), realTime2Frame(), DSSIPluginInstance::sendEvent(), toDouble(), toFrameText(), toMSText(), toSecText(), and writeSecPart().
int RealTime::nsec |
Definition at line 45 of file RealTime.h.
Referenced by frame2RealTime_i(), fromString(), operator*(), operator+(), operator-(), operator/(), operator<(), operator<<(), operator<=(), operator=(), operator==(), operator>(), operator>=(), RealTime(), realTime2Frame(), DSSIPluginInstance::sendEvent(), toDouble(), and toFrameText().
|
static |
Definition at line 204 of file RealTime.h.
Referenced by MIDIFileReader::calculateTempoTimestamps(), frame2RealTime(), fromMicroseconds(), fromMilliseconds(), MIDIFileReader::getTimeForMIDITime(), RDFTransformFactoryImpl::getTransforms(), operator<<(), realTime2Frame(), toFrameText(), toMSText(), toSecText(), toString(), toText(), MIDIFileReader::updateTempoMap(), and RDFTransformFactoryImpl::writeTransformToRDF().
The documentation for this struct was generated from the following files:
Generated by
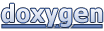