svcore
1.9
|
CSVFeatureWriter.cpp
Go to the documentation of this file.
52 return "Write features in comma-separated (CSV) format. If transforms are being written to a single file or to stdout, the first column in the output will contain the input audio filename, or an empty string if the feature hails from the same audio file as its predecessor. If transforms are being written to multiple files, the audio filename column will be omitted. Subsequent columns will contain the feature timestamp, then any or all of duration, values, and label.";
67 p.description = "Omit the filename column. May result in confusion if sending more than one audio file's features to the same CSV output.";
77 p.description = "Show start and end time instead of start and duration, for features with duration.";
82 p.description = "Include durations (or end times) even for features without duration, by using the gap to the next feature instead.";
87 p.description = "Specify the number of significant digits to use when printing transform outputs. Outputs are represented internally using single-precision floating-point, so digits beyond the 8th or 9th place are usually meaningless. The default is 6.";
120 SVCERR << "CSVFeatureWriter: ERROR: Invalid or out-of-range value for number of significant digits: " << i->second << endl;
QTextStream * getOutputStream(QString, TransformId, QTextCodec *)
Definition: FileFeatureWriter.cpp:317
void writeFeature(DataId, QTextStream &, const Vamp::Plugin::Feature &f, const Vamp::Plugin::Feature *optionalNextFeature, std::string summaryType)
Definition: CSVFeatureWriter.cpp:207
ParameterList getSupportedParameters() const override
Definition: CSVFeatureWriter.cpp:56
Definition: FeatureWriter.h:44
std::string toString(bool align=false) const
Return a human-readable debug-type string to full precision (probably not a format to show to a user ...
Definition: RealTimeSV.cpp:213
string getDescription() const override
Definition: CSVFeatureWriter.cpp:50
void setParameters(map< string, string > ¶ms) override
Definition: FileFeatureWriter.cpp:123
static sv_frame_t realTime2Frame(const RealTime &r, sv_samplerate_t sampleRate)
Convert a RealTime into a sample frame at the given sample rate.
Definition: RealTimeSV.cpp:490
void setParameters(map< string, string > ¶ms) override
Definition: CSVFeatureWriter.cpp:95
Definition: Transform.h:34
PendingSummaryTypes m_pendingSummaryTypes
Definition: CSVFeatureWriter.h:70
Definition: FileFeatureWriter.h:38
ParameterList getSupportedParameters() const override
Definition: FileFeatureWriter.cpp:72
void write(QString trackid, const Transform &transform, const Vamp::Plugin::OutputDescriptor &output, const Vamp::Plugin::FeatureList &features, std::string summaryType="") override
Definition: CSVFeatureWriter.cpp:130
RealTime represents time values to nanosecond precision with accurate arithmetic and frame-rate conve...
Definition: RealTime.h:42
Generated by
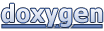