svcore
1.9
|
FeatureWriter.h
Go to the documentation of this file.
QString m_transformId
Definition: FeatureWriter.h:84
virtual ~FailedToOpenOutputStream()
Definition: FeatureWriter.h:74
virtual void write(QString trackid, const Transform &transform, const Vamp::Plugin::OutputDescriptor &output, const Vamp::Plugin::FeatureList &features, std::string summaryType="")=0
Definition: FeatureWriter.h:44
virtual void setTrackMetadata(QString, TrackMetadata)
Definition: FeatureWriter.h:65
virtual void testOutputFile(QString, TransformId)
Throw FailedToOpenOutputStream if we can already tell that we will be unable to write to the output f...
Definition: FeatureWriter.h:110
virtual void setNofM(int, int)
Notify the writer that we are about to start extraction for input file N of M (where N is 1...
Definition: FeatureWriter.h:93
virtual void setParameters(map< string, string > &)
Definition: FeatureWriter.h:56
FailedToOpenOutputStream(QString trackId, QString transformId)
Definition: FeatureWriter.h:70
virtual QString getWriterTag() const =0
Definition: FeatureWriter.h:37
virtual string getDescription() const =0
Definition: FeatureWriter.h:60
Definition: Transform.h:34
virtual void finish()=0
const char * what() const override
Definition: FeatureWriter.h:75
virtual ParameterList getSupportedParameters() const
Definition: FeatureWriter.h:52
RealTime represents time values to nanosecond precision with accurate arithmetic and frame-rate conve...
Definition: RealTime.h:42
Generated by
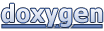