svcore
1.9
|
RealTime.h
Go to the documentation of this file.
static RealTime fromTimeval(const struct timeval &)
Definition: RealTimeSV.cpp:90
static RealTime fromString(std::string)
Convert a string as obtained from toString back to a RealTime object.
Definition: RealTimeSV.cpp:230
static RealTime fromMilliseconds(int64_t msec)
Definition: RealTimeSV.cpp:64
static RealTime frame2RealTime(sv_frame_t frame, sv_samplerate_t sampleRate)
Convert a sample frame at the given sample rate into a RealTime.
Definition: RealTimeSV.cpp:498
std::string toXsdDuration() const
Return a string in xsd:duration format.
Definition: RealTimeSV.cpp:424
std::string toString(bool align=false) const
Return a human-readable debug-type string to full precision (probably not a format to show to a user ...
Definition: RealTimeSV.cpp:213
std::string toMSText(bool fixedDp, bool hms) const
Return a user-readable string to the nearest millisecond.
Definition: RealTimeSV.cpp:332
std::string toSecText() const
Return a user-readable string to the nearest second, in H:M:S form.
Definition: RealTimeSV.cpp:406
static sv_frame_t realTime2Frame(const RealTime &r, sv_samplerate_t sampleRate)
Convert a RealTime into a sample frame at the given sample rate.
Definition: RealTimeSV.cpp:490
std::ostream & operator<<(std::ostream &out, const RealTime &rt)
Definition: RealTimeSV.cpp:188
std::string toFrameText(int fps, bool hms, std::string frameDelimiter=":") const
Return a user-readable string in which seconds are divided into frames (presumably at a lower frame r...
Definition: RealTimeSV.cpp:367
std::string toText(bool fixedDp=false) const
Return a user-readable string to the nearest millisecond, typically in a form like HH:MM:SS...
Definition: RealTimeSV.cpp:274
static RealTime fromMicroseconds(int64_t usec)
Definition: RealTimeSV.cpp:77
static RealTime fromXsdDuration(std::string xsdd)
Definition: RealTimeSV.cpp:96
RealTime represents time values to nanosecond precision with accurate arithmetic and frame-rate conve...
Definition: RealTime.h:42
Generated by
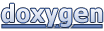