svcore
1.9
|
#include <Transform.h>
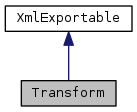
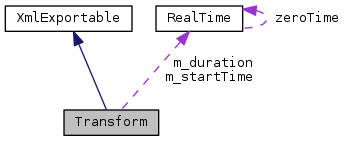
Public Types | |
enum | Type { FeatureExtraction, RealTimeEffect, UnknownType } |
enum | SummaryType { Minimum = 0, Maximum = 1, Mean = 2, Median = 3, Mode = 4, Sum = 5, Variance = 6, StandardDeviation = 7, Count = 8, NoSummary = 999 } |
typedef std::map< QString, float > | ParameterMap |
typedef std::map< QString, QString > | ConfigurationMap |
enum | { NO_ID = -1 } |
typedef int | ExportId |
Public Member Functions | |
Transform () | |
Construct a new Transform with default data and no identifier. More... | |
Transform (QString xml) | |
Construct a Transform by parsing the given XML data string. More... | |
virtual | ~Transform () |
bool | operator== (const Transform &) const |
Compare two Transforms. More... | |
bool | operator< (const Transform &) const |
Order two Transforms, so that they can be used as keys in containers. More... | |
void | setIdentifier (TransformId id) |
TransformId | getIdentifier () const |
Type | getType () const |
QString | getPluginIdentifier () const |
QString | getOutput () const |
void | setPluginIdentifier (QString pluginIdentifier) |
void | setOutput (QString output) |
const ParameterMap & | getParameters () const |
void | setParameters (const ParameterMap &pm) |
void | setParameter (QString name, float value) |
const ConfigurationMap & | getConfiguration () const |
void | setConfiguration (const ConfigurationMap &cm) |
void | setConfigurationValue (QString name, QString value) |
SummaryType | getSummaryType () const |
void | setSummaryType (SummaryType type) |
QString | getPluginVersion () const |
void | setPluginVersion (QString version) |
QString | getProgram () const |
void | setProgram (QString program) |
int | getStepSize () const |
void | setStepSize (int s) |
int | getBlockSize () const |
void | setBlockSize (int s) |
WindowType | getWindowType () const |
void | setWindowType (WindowType type) |
RealTime | getStartTime () const |
void | setStartTime (RealTime t) |
RealTime | getDuration () const |
void | setDuration (RealTime d) |
sv_samplerate_t | getSampleRate () const |
void | setSampleRate (sv_samplerate_t rate) |
void | toXml (QTextStream &stream, QString indent="", QString extraAttributes="") const override |
Stream this exportable object out to XML on a text stream. More... | |
void | setFromXmlAttributes (const QXmlAttributes &) |
Set the main transform data from the given XML attributes. More... | |
QString | getErrorString () const |
ExportId | getExportId () const |
Return the numerical export identifier for this object. More... | |
virtual QString | toXmlString (QString indent="", QString extraAttributes="") const |
Convert this exportable object to XML in a string. More... | |
Static Public Member Functions | |
static TransformId | getIdentifierForPluginOutput (QString pluginIdentifier, QString output="") |
static SummaryType | stringToSummaryType (QString) |
static QString | summaryTypeToString (SummaryType) |
static QString | encodeEntities (QString) |
static QString | encodeColour (int r, int g, int b) |
Protected Member Functions | |
template<typename A , typename B > | |
bool | mapLessThan (const std::map< A, B > &a, const std::map< A, B > &b) const |
Static Protected Member Functions | |
static QString | createIdentifier (QString type, QString soName, QString label, QString output) |
static void | parseIdentifier (QString identifier, QString &type, QString &soName, QString &label, QString &output) |
Protected Attributes | |
TransformId | m_id |
ParameterMap | m_parameters |
ConfigurationMap | m_configuration |
SummaryType | m_summaryType |
QString | m_pluginVersion |
QString | m_program |
int | m_stepSize |
int | m_blockSize |
WindowType | m_windowType |
RealTime | m_startTime |
RealTime | m_duration |
sv_samplerate_t | m_sampleRate |
QString | m_errorString |
Detailed Description
Definition at line 34 of file Transform.h.
Member Typedef Documentation
typedef std::map<QString, float> Transform::ParameterMap |
Definition at line 86 of file Transform.h.
typedef std::map<QString, QString> Transform::ConfigurationMap |
Definition at line 92 of file Transform.h.
|
inherited |
Definition at line 33 of file XmlExportable.h.
Member Enumeration Documentation
enum Transform::Type |
Enumerator | |
---|---|
FeatureExtraction | |
RealTimeEffect | |
UnknownType |
Definition at line 71 of file Transform.h.
Enumerator | |
---|---|
Minimum | |
Maximum | |
Mean | |
Median | |
Mode | |
Sum | |
Variance | |
StandardDeviation | |
Count | |
NoSummary |
Definition at line 98 of file Transform.h.
|
inherited |
Enumerator | |
---|---|
NO_ID |
Definition at line 28 of file XmlExportable.h.
Constructor & Destructor Documentation
Transform::Transform | ( | ) |
Construct a new Transform with default data and no identifier.
The Transform object will be meaningless until some data and an identifier have been set on it.
To construct a Transform for use with a particular transform identifier, use TransformFactory::getDefaultTransformFor.
Definition at line 34 of file Transform.cpp.
Transform::Transform | ( | QString | xml | ) |
Construct a Transform by parsing the given XML data string.
This is the inverse of toXmlString. If this fails, getErrorString() will return a non-empty string.
Definition at line 43 of file Transform.cpp.
References m_errorString, setConfigurationValue(), setFromXmlAttributes(), and setParameter().
|
virtual |
Definition at line 104 of file Transform.cpp.
Member Function Documentation
bool Transform::operator== | ( | const Transform & | t | ) | const |
Compare two Transforms.
They only compare equal if every data element matches.
Definition at line 109 of file Transform.cpp.
References m_blockSize, m_configuration, m_duration, m_id, m_parameters, m_program, m_sampleRate, m_startTime, m_stepSize, m_summaryType, and m_windowType.
bool Transform::operator< | ( | const Transform & | t | ) | const |
Order two Transforms, so that they can be used as keys in containers.
Definition at line 134 of file Transform.cpp.
References m_blockSize, m_configuration, m_duration, m_id, m_parameters, m_program, m_sampleRate, m_startTime, m_stepSize, m_summaryType, and m_windowType.
void Transform::setIdentifier | ( | TransformId | id | ) |
Definition at line 173 of file Transform.cpp.
References m_id.
Referenced by TransformFactory::getDefaultTransformFor(), TransformFactory::getTransformInputDomain(), TransformFactory::instantiateDefaultPluginFor(), and setFromXmlAttributes().
TransformId Transform::getIdentifier | ( | ) | const |
Definition at line 179 of file Transform.cpp.
References m_id.
Referenced by ModelTransformerFactory::getConfigurationForTransform(), TransformFactory::getPluginConfigurationXml(), TransformFactory::instantiatePluginFor(), RealTimeEffectModelTransformer::RealTimeEffectModelTransformer(), TransformFactory::setParametersFromPluginConfigurationXml(), CSVFeatureWriter::write(), RDFFeatureWriter::write(), RDFFeatureWriter::writeDenseRDF(), RDFFeatureWriter::writeLocalFeatureTypes(), and RDFFeatureWriter::writeSparseRDF().
Transform::Type Transform::getType | ( | ) | const |
Definition at line 203 of file Transform.cpp.
References FeatureExtraction, getPluginIdentifier(), RealTimePluginFactory::instanceFor(), and RealTimeEffect.
Referenced by TransformFactory::getTransformInputDomain(), and TransformFactory::instantiateDefaultPluginFor().
QString Transform::getPluginIdentifier | ( | ) | const |
Definition at line 213 of file Transform.cpp.
References m_id.
Referenced by ModelTransformerFactory::getConfigurationForTransform(), getType(), FeatureExtractionModelTransformer::initialise(), TransformFactory::instantiateDefaultPluginFor(), RealTimeEffectModelTransformer::RealTimeEffectModelTransformer(), setOutput(), RDFFeatureWriter::write(), and RDFTransformFactoryImpl::writeTransformToRDF().
QString Transform::getOutput | ( | ) | const |
Definition at line 219 of file Transform.cpp.
References m_id.
Referenced by areTransformsSimilar(), RealTimeEffectModelTransformer::RealTimeEffectModelTransformer(), setPluginIdentifier(), and RDFTransformFactoryImpl::writeTransformToRDF().
void Transform::setPluginIdentifier | ( | QString | pluginIdentifier | ) |
Definition at line 225 of file Transform.cpp.
References getOutput(), and m_id.
Referenced by RDFTransformFactoryImpl::getTransforms().
void Transform::setOutput | ( | QString | output | ) |
Definition at line 231 of file Transform.cpp.
References getPluginIdentifier(), and m_id.
Referenced by areTransformsSimilar(), and RDFTransformFactoryImpl::setOutput().
|
static |
Definition at line 237 of file Transform.cpp.
Referenced by TransformFactory::populateUninstalledTransforms().
const Transform::ParameterMap & Transform::getParameters | ( | ) | const |
Definition at line 244 of file Transform.cpp.
References m_parameters.
Referenced by TransformFactory::setPluginParameters(), and RDFTransformFactoryImpl::writeTransformToRDF().
void Transform::setParameters | ( | const ParameterMap & | pm | ) |
Definition at line 250 of file Transform.cpp.
References m_parameters.
Referenced by TransformFactory::setParametersFromPlugin().
void Transform::setParameter | ( | QString | name, |
float | value | ||
) |
Definition at line 256 of file Transform.cpp.
References m_parameters.
Referenced by RDFTransformFactoryImpl::setParameters(), and Transform().
const Transform::ConfigurationMap & Transform::getConfiguration | ( | ) | const |
Definition at line 263 of file Transform.cpp.
References m_configuration.
Referenced by TransformFactory::setPluginParameters().
void Transform::setConfiguration | ( | const ConfigurationMap & | cm | ) |
Definition at line 269 of file Transform.cpp.
References m_configuration.
Referenced by TransformFactory::setParametersFromPlugin().
void Transform::setConfigurationValue | ( | QString | name, |
QString | value | ||
) |
Definition at line 275 of file Transform.cpp.
References m_configuration, and SVDEBUG.
Referenced by Transform().
Transform::SummaryType Transform::getSummaryType | ( | ) | const |
Definition at line 306 of file Transform.cpp.
References m_summaryType.
Referenced by RDFTransformFactoryImpl::writeTransformToRDF().
void Transform::setSummaryType | ( | SummaryType | type | ) |
Definition at line 312 of file Transform.cpp.
References m_summaryType.
Referenced by RDFTransformFactoryImpl::getTransforms(), and setFromXmlAttributes().
QString Transform::getPluginVersion | ( | ) | const |
Definition at line 282 of file Transform.cpp.
References m_pluginVersion.
Referenced by FeatureExtractionModelTransformer::initialise(), and RDFTransformFactoryImpl::writeTransformToRDF().
void Transform::setPluginVersion | ( | QString | version | ) |
Definition at line 288 of file Transform.cpp.
References m_pluginVersion.
Referenced by TransformFactory::getDefaultTransformFor(), RDFTransformFactoryImpl::getTransforms(), and setFromXmlAttributes().
QString Transform::getProgram | ( | ) | const |
Definition at line 294 of file Transform.cpp.
References m_program.
Referenced by TransformFactory::setPluginParameters(), and RDFTransformFactoryImpl::writeTransformToRDF().
void Transform::setProgram | ( | QString | program | ) |
Definition at line 300 of file Transform.cpp.
References m_program.
Referenced by RDFTransformFactoryImpl::getTransforms(), setFromXmlAttributes(), and TransformFactory::setParametersFromPlugin().
int Transform::getStepSize | ( | ) | const |
Definition at line 318 of file Transform.cpp.
References m_stepSize.
Referenced by FeatureExtractionModelTransformer::initialise(), TransformFactory::makeContextConsistentWithPlugin(), FeatureExtractionModelTransformer::run(), RDFFeatureWriter::writeDenseRDF(), and RDFTransformFactoryImpl::writeTransformToRDF().
void Transform::setStepSize | ( | int | s | ) |
Definition at line 324 of file Transform.cpp.
References m_stepSize.
Referenced by RDFTransformFactoryImpl::getTransforms(), TransformFactory::makeContextConsistentWithPlugin(), and setFromXmlAttributes().
int Transform::getBlockSize | ( | ) | const |
Definition at line 330 of file Transform.cpp.
References m_blockSize.
Referenced by FeatureExtractionModelTransformer::initialise(), TransformFactory::makeContextConsistentWithPlugin(), RealTimeEffectModelTransformer::RealTimeEffectModelTransformer(), FeatureExtractionModelTransformer::run(), RDFFeatureWriter::writeDenseRDF(), and RDFTransformFactoryImpl::writeTransformToRDF().
void Transform::setBlockSize | ( | int | s | ) |
Definition at line 336 of file Transform.cpp.
References m_blockSize.
Referenced by RDFTransformFactoryImpl::getTransforms(), TransformFactory::makeContextConsistentWithPlugin(), RealTimeEffectModelTransformer::RealTimeEffectModelTransformer(), and setFromXmlAttributes().
WindowType Transform::getWindowType | ( | ) | const |
Definition at line 342 of file Transform.cpp.
References m_windowType.
Referenced by FeatureExtractionModelTransformer::run(), and RDFTransformFactoryImpl::writeTransformToRDF().
void Transform::setWindowType | ( | WindowType | type | ) |
Definition at line 348 of file Transform.cpp.
References m_windowType.
Referenced by RDFTransformFactoryImpl::getTransforms(), and setFromXmlAttributes().
RealTime Transform::getStartTime | ( | ) | const |
Definition at line 354 of file Transform.cpp.
References m_startTime.
Referenced by RealTimeEffectModelTransformer::run(), FeatureExtractionModelTransformer::run(), RDFFeatureWriter::writeDenseRDF(), and RDFTransformFactoryImpl::writeTransformToRDF().
void Transform::setStartTime | ( | RealTime | t | ) |
Definition at line 360 of file Transform.cpp.
References m_startTime.
Referenced by RDFTransformFactoryImpl::getTransforms(), and setFromXmlAttributes().
RealTime Transform::getDuration | ( | ) | const |
Definition at line 366 of file Transform.cpp.
References m_duration.
Referenced by RealTimeEffectModelTransformer::run(), FeatureExtractionModelTransformer::run(), RDFFeatureWriter::writeDenseRDF(), and RDFTransformFactoryImpl::writeTransformToRDF().
void Transform::setDuration | ( | RealTime | d | ) |
Definition at line 372 of file Transform.cpp.
References m_duration.
Referenced by RDFTransformFactoryImpl::getTransforms(), and setFromXmlAttributes().
sv_samplerate_t Transform::getSampleRate | ( | ) | const |
Definition at line 378 of file Transform.cpp.
References m_sampleRate.
Referenced by TransformFactory::instantiatePluginFor(), RDFFeatureWriter::writeDenseRDF(), CSVFeatureWriter::writeFeature(), and RDFTransformFactoryImpl::writeTransformToRDF().
void Transform::setSampleRate | ( | sv_samplerate_t | rate | ) |
Definition at line 384 of file Transform.cpp.
References m_sampleRate.
Referenced by TransformFactory::getDefaultTransformFor(), RDFTransformFactoryImpl::getTransforms(), and setFromXmlAttributes().
|
overridevirtual |
Stream this exportable object out to XML on a text stream.
Implements XmlExportable.
Definition at line 390 of file Transform.cpp.
References XmlExportable::encodeEntities(), m_blockSize, m_configuration, m_duration, m_id, m_parameters, m_pluginVersion, m_program, m_sampleRate, m_startTime, m_stepSize, m_summaryType, m_windowType, NoSummary, summaryTypeToString(), and RealTime::toString().
void Transform::setFromXmlAttributes | ( | const QXmlAttributes & | attrs | ) |
Set the main transform data from the given XML attributes.
This does not set the parameters or configuration, which are exported to separate XML elements rather than attributes of the transform element.
Note that this only sets those attributes which are actually present in the argument. Any attributes not defined in the attribute will remain unchanged in the Transform. If your aim is to create a transform exactly matching the given attributes, ensure you start from an empty transform rather than one that has already been configured.
Definition at line 486 of file Transform.cpp.
References RealTime::fromString(), setBlockSize(), setDuration(), setIdentifier(), setPluginVersion(), setProgram(), setSampleRate(), setStartTime(), setStepSize(), setSummaryType(), setWindowType(), and stringToSummaryType().
Referenced by Transform().
|
inline |
Definition at line 160 of file Transform.h.
References m_errorString, stringToSummaryType(), and summaryTypeToString().
|
static |
Definition at line 445 of file Transform.cpp.
References Count, Maximum, Mean, Median, Minimum, Mode, NoSummary, StandardDeviation, Sum, SVDEBUG, and Variance.
Referenced by getErrorString(), RDFTransformFactoryImpl::getTransforms(), and setFromXmlAttributes().
|
static |
Definition at line 465 of file Transform.cpp.
References Count, Maximum, Mean, Median, Minimum, Mode, NoSummary, StandardDeviation, Sum, SVDEBUG, and Variance.
Referenced by getErrorString(), toXml(), and RDFTransformFactoryImpl::writeTransformToRDF().
|
staticprotected |
Definition at line 185 of file Transform.cpp.
References PluginIdentifier::createIdentifier().
|
staticprotected |
Definition at line 193 of file Transform.cpp.
References PluginIdentifier::parseIdentifier().
|
inlineprotected |
Definition at line 176 of file Transform.h.
|
inherited |
Return the numerical export identifier for this object.
It's allocated the first time this is called, so objects on which this is never called do not get allocated one.
Definition at line 71 of file XmlExportable.cpp.
References XmlExportable::m_exportId, and mutex.
Referenced by EditableDenseThreeDimensionalModel::toXml(), BasicCompressedDenseThreeDimensionalModel::toXml(), EventSeries::toXml(), ImageModel::toXml(), TextModel::toXml(), Model::toXml(), SparseOneDimensionalModel::toXml(), RegionModel::toXml(), BoxModel::toXml(), SparseTimeValueModel::toXml(), NoteModel::toXml(), and XmlExportable::~XmlExportable().
|
virtualinherited |
Convert this exportable object to XML in a string.
The default implementation calls toXml and returns the result as a string. Do not override this unless you really know what you're doing.
Definition at line 25 of file XmlExportable.cpp.
References XmlExportable::toXml().
Referenced by ModelTransformerFactory::getConfigurationForTransform(), RDFTransformFactoryImpl::getTransforms(), and XmlExportable::~XmlExportable().
|
staticinherited |
Definition at line 41 of file XmlExportable.cpp.
Referenced by TextMatcher::test(), PluginXml::toXml(), ReadOnlyWaveFileModel::toXml(), toXml(), WritableWaveFileModel::toXml(), Model::toXml(), Event::toXml(), RegionModel::toXml(), BoxModel::toXml(), SparseTimeValueModel::toXml(), NoteModel::toXml(), and XmlExportable::~XmlExportable().
|
staticinherited |
Definition at line 54 of file XmlExportable.cpp.
Referenced by XmlExportable::~XmlExportable().
Member Data Documentation
|
protected |
Definition at line 166 of file Transform.h.
Referenced by getIdentifier(), getOutput(), getPluginIdentifier(), operator<(), operator==(), setIdentifier(), setOutput(), setPluginIdentifier(), and toXml().
|
protected |
Definition at line 190 of file Transform.h.
Referenced by getParameters(), operator<(), operator==(), setParameter(), setParameters(), and toXml().
|
protected |
Definition at line 191 of file Transform.h.
Referenced by getConfiguration(), operator<(), operator==(), setConfiguration(), setConfigurationValue(), and toXml().
|
protected |
Definition at line 192 of file Transform.h.
Referenced by getSummaryType(), operator<(), operator==(), setSummaryType(), and toXml().
|
protected |
Definition at line 193 of file Transform.h.
Referenced by getPluginVersion(), setPluginVersion(), and toXml().
|
protected |
Definition at line 194 of file Transform.h.
Referenced by getProgram(), operator<(), operator==(), setProgram(), and toXml().
|
protected |
Definition at line 195 of file Transform.h.
Referenced by getStepSize(), operator<(), operator==(), setStepSize(), and toXml().
|
protected |
Definition at line 196 of file Transform.h.
Referenced by getBlockSize(), operator<(), operator==(), setBlockSize(), and toXml().
|
protected |
Definition at line 197 of file Transform.h.
Referenced by getWindowType(), operator<(), operator==(), setWindowType(), and toXml().
|
protected |
Definition at line 198 of file Transform.h.
Referenced by getStartTime(), operator<(), operator==(), setStartTime(), and toXml().
|
protected |
Definition at line 199 of file Transform.h.
Referenced by getDuration(), operator<(), operator==(), setDuration(), and toXml().
|
protected |
Definition at line 200 of file Transform.h.
Referenced by getSampleRate(), operator<(), operator==(), setSampleRate(), and toXml().
|
protected |
Definition at line 201 of file Transform.h.
Referenced by getErrorString(), and Transform().
The documentation for this class was generated from the following files:
Generated by
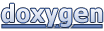