svcore
1.9
|
WritableWaveFileModel.cpp
Go to the documentation of this file.
122 SVCERR << "WritableWaveFileModel: Error in creating WAV file writer: " << m_targetWriter->getError() << endl;
139 SVCERR << "WritableWaveFileModel: Error in creating temporary WAV file writer: " << m_temporaryWriter->getError() << endl;
148 SVCERR << "WritableWaveFileModel: Error in creating wave file reader: " << m_reader->getError() << endl;
217 SVCERR << "ERROR: WritableWaveFileModel::addSamples: writer failed: " << writer->getError() << endl;
288 SVCERR << "WritableWaveFileModel: Error in creating normalising reader: " << normalisingReader.getError() << endl;
299 SVCERR << "ERROR: WritableWaveFileModel::normaliseToTarget: writer failed: " << m_targetWriter->getError() << endl;
void toXml(QTextStream &stream, QString indent="", QString extraAttributes="") const override
Stream this exportable object out to XML on a text stream.
Definition: Model.cpp:204
int getSummaryBlockSize(int desired) const override
Definition: WritableWaveFileModel.cpp:335
static PlayParameterRepository * getInstance()
Definition: PlayParameterRepository.cpp:26
Definition: WavFileWriter.h:51
ReadOnlyWaveFileModel * m_model
Definition: WritableWaveFileModel.h:207
void writeCompleted(ModelId)
std::vector< floatvec_t > getMultiChannelData(int fromchannel, int tochannel, sv_frame_t start, sv_frame_t count) const override
Get the specified set of samples from given contiguous range of channels of the model in single-preci...
Definition: WritableWaveFileModel.cpp:327
int getWriteProportion() const
Get the proportion of the file which has been written so far, as a percentage.
Definition: WritableWaveFileModel.cpp:253
Range getSummary(int channel, sv_frame_t start, sv_frame_t count) const override
Return the range from the given start frame, corresponding to the given number of underlying sample f...
Definition: WritableWaveFileModel.cpp:352
std::vector< Range > RangeBlock
Definition: RangeSummarisableTimeValueModel.h:76
void componentModelChanged(ModelId)
Definition: WritableWaveFileModel.cpp:182
void setWriteProportion(int proportion)
Set the proportion of the file which has been written so far, as a percentage.
Definition: WritableWaveFileModel.cpp:247
bool isOK() const override
Return true if the model was constructed successfully.
Definition: ReadOnlyWaveFileModel.cpp:140
sv_samplerate_t m_sampleRate
Definition: WritableWaveFileModel.h:228
Normalisation m_normalisation
Definition: WritableWaveFileModel.h:227
QString m_temporaryPath
Definition: WritableWaveFileModel.h:214
std::vector< float, breakfastquay::StlAllocator< float > > floatvec_t
Definition: BaseTypes.h:53
void setStartFrame(sv_frame_t startFrame) override
Definition: WritableWaveFileModel.cpp:194
void componentModelChangedWithin(ModelId, sv_frame_t, sv_frame_t)
Definition: WritableWaveFileModel.cpp:188
int getSummaryBlockSize(int desired) const override
Definition: ReadOnlyWaveFileModel.cpp:377
WavFileWriter * m_temporaryWriter
When normalising, this writer is used to write verbatim samples to the temporary file prior to normal...
Definition: WritableWaveFileModel.h:213
static const int PROPORTION_UNKNOWN
Definition: WritableWaveFileModel.h:133
floatvec_t getData(int channel, sv_frame_t start, sv_frame_t count) const override
Get the specified set of samples from the given channel of the model in single-precision floating-poi...
Definition: WritableWaveFileModel.cpp:320
WavFileWriter * m_targetWriter
When not normalising, this writer is used to write verbatim samples direct to the target file...
Definition: WritableWaveFileModel.h:223
WritableWaveFileModel(QString path, sv_samplerate_t sampleRate, int channels, Normalisation normalisation)
Create a WritableWaveFileModel of the given sample rate and channel count, storing data in a new floa...
Definition: WritableWaveFileModel.cpp:40
FileSource is a class used to refer to the contents of a file that may be either local or at a remote...
Definition: FileSource.h:59
QString getError() const override
If isOK() is false, return an error string.
Definition: WavFileReader.h:52
void normaliseToTarget()
Definition: WritableWaveFileModel.cpp:277
void getSummaries(int channel, sv_frame_t start, sv_frame_t count, RangeBlock &ranges, int &blockSize) const override
Return ranges from the given start frame, corresponding to the given number of underlying sample fram...
Definition: WritableWaveFileModel.cpp:342
void modelChangedWithin(ModelId myId, sv_frame_t startFrame, sv_frame_t endFrame)
Emitted when a model has been edited (or more data retrieved from cache, in the case of a cached mode...
Range getSummary(int channel, sv_frame_t start, sv_frame_t count) const override
Return the range from the given start frame, corresponding to the given number of underlying sample f...
Definition: ReadOnlyWaveFileModel.cpp:525
void toXml(QTextStream &out, QString indent="", QString extraAttributes="") const override
Stream this exportable object out to XML on a text stream.
Definition: WritableWaveFileModel.cpp:359
bool isOK() const override
Return true if the model was constructed successfully.
Definition: WritableWaveFileModel.cpp:241
void addPlayable(int id, const Playable *)
Register a playable.
Definition: PlayParameterRepository.cpp:36
void updateModel()
Tell the model to update its own (read) view of the (written) file.
Definition: WritableWaveFileModel.cpp:233
sv_frame_t getFrameCount() const override
Definition: WritableWaveFileModel.cpp:313
Definition: WavFileWriter.h:34
sv_frame_t getFrameCount() const
Return the number of audio sample frames (i.e.
Definition: AudioFileReader.h:49
Definition: Exceptions.h:47
floatvec_t getInterleavedFrames(sv_frame_t start, sv_frame_t count) const override
Must be safe to call from multiple threads with different arguments on the same object at the same ti...
Definition: WavFileReader.cpp:164
Definition: ReadOnlyWaveFileModel.h:36
void setStartFrame(sv_frame_t startFrame) override
Definition: ReadOnlyWaveFileModel.h:84
int getChannelCount() const override
Return the number of distinct channels for this model.
Definition: ReadOnlyWaveFileModel.cpp:191
Definition: ById.h:115
floatvec_t getData(int channel, sv_frame_t start, sv_frame_t count) const override
Get the specified set of samples from the given channel of the model in single-precision floating-poi...
Definition: ReadOnlyWaveFileModel.cpp:249
~WritableWaveFileModel()
Definition: WritableWaveFileModel.cpp:170
virtual bool addSamples(const float *const *samples, sv_frame_t count)
Call addSamples to append a block of samples to the end of the file.
Definition: WritableWaveFileModel.cpp:203
bool putInterleavedFrames(const floatvec_t &frames)
As writeSamples, but compatible with WavFileReader api. More expensive.
Definition: WavFileWriter.cpp:204
bool writeSamples(const float *const *samples, sv_frame_t count)
Write samples from raw arrays; count is per-channel.
Definition: WavFileWriter.cpp:176
void modelChanged(ModelId myId)
Emitted when a model has been edited (or more data retrieved from cache, in the case of a cached mode...
void getSummaries(int channel, sv_frame_t start, sv_frame_t count, RangeBlock &ranges, int &blockSize) const override
Return ranges from the given start frame, corresponding to the given number of underlying sample fram...
Definition: ReadOnlyWaveFileModel.cpp:394
int getChannelCount() const
Return the number of channels in the file.
Definition: AudioFileReader.h:54
std::vector< floatvec_t > getMultiChannelData(int fromchannel, int tochannel, sv_frame_t start, sv_frame_t count) const override
Get the specified set of samples from given contiguous range of channels of the model in single-preci...
Definition: ReadOnlyWaveFileModel.cpp:313
Generated by
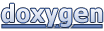