svcore
1.9
|
WavFileReader.h
Go to the documentation of this file.
bool isUpdating() const override
Return true if decoding is still in progress and the frame count may change.
Definition: WavFileReader.h:74
QString getLocation() const
Return the location filename or URL as passed to the constructor.
Definition: FileSource.cpp:616
int getDecodeCompletion() const override
Return a percentage value indicating how far through decoding the audio file we are.
Definition: WavFileReader.h:72
Definition: AudioFileReader.h:27
static bool supportsExtension(QString ext)
Definition: WavFileReader.cpp:309
floatvec_t getInterleavedFramesUnnormalised(sv_frame_t start, sv_frame_t count) const
Definition: WavFileReader.cpp:180
static bool supportsContentType(QString type)
Definition: WavFileReader.cpp:317
WavFileReader(FileSource source, bool fileUpdating=false, Normalisation normalise=Normalisation::None)
Definition: WavFileReader.cpp:28
bool isQuicklySeekable() const override
Return true if this file supports fast seek and random access.
Definition: WavFileReader.h:59
std::vector< float, breakfastquay::StlAllocator< float > > floatvec_t
Definition: BaseTypes.h:53
FileSource is a class used to refer to the contents of a file that may be either local or at a remote...
Definition: FileSource.h:59
static void getSupportedExtensions(std::set< QString > &extensions)
Definition: WavFileReader.cpp:280
QString getError() const override
If isOK() is false, return an error string.
Definition: WavFileReader.h:52
QString getLocation() const override
Return the location of the audio data in the reader (as passed in to the FileSource constructor...
Definition: WavFileReader.h:51
QString getTitle() const override
Return the title of the work in the audio file, if known.
Definition: WavFileReader.h:54
floatvec_t getInterleavedFrames(sv_frame_t start, sv_frame_t count) const override
Must be safe to call from multiple threads with different arguments on the same object at the same ti...
Definition: WavFileReader.cpp:164
QString getMaker() const override
Return the "maker" of the work in the audio file, if known.
Definition: WavFileReader.h:55
QString getLocalFilename() const override
Return the local file path of the audio data.
Definition: WavFileReader.h:57
Generated by
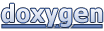