svcore
1.9
|
Reader for audio files using libsndfile. More...
#include <WavFileReader.h>
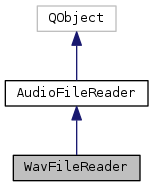
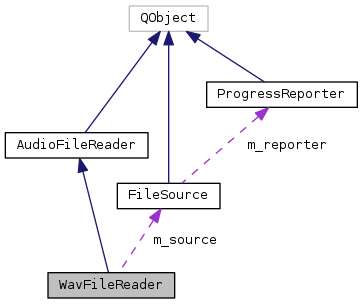
Public Types | |
enum | Normalisation { Normalisation::None, Normalisation::Peak } |
typedef std::map< QString, QString > | TagMap |
Return any tag pairs picked up from the audio file. More... | |
Signals | |
void | frameCountChanged () |
Public Member Functions | |
WavFileReader (FileSource source, bool fileUpdating=false, Normalisation normalise=Normalisation::None) | |
virtual | ~WavFileReader () |
QString | getLocation () const override |
Return the location of the audio data in the reader (as passed in to the FileSource constructor, for example). More... | |
QString | getError () const override |
If isOK() is false, return an error string. More... | |
QString | getTitle () const override |
Return the title of the work in the audio file, if known. More... | |
QString | getMaker () const override |
Return the "maker" of the work in the audio file, if known. More... | |
QString | getLocalFilename () const override |
Return the local file path of the audio data. More... | |
bool | isQuicklySeekable () const override |
Return true if this file supports fast seek and random access. More... | |
floatvec_t | getInterleavedFrames (sv_frame_t start, sv_frame_t count) const override |
Must be safe to call from multiple threads with different arguments on the same object at the same time. More... | |
int | getDecodeCompletion () const override |
Return a percentage value indicating how far through decoding the audio file we are. More... | |
bool | isUpdating () const override |
Return true if decoding is still in progress and the frame count may change. More... | |
void | updateFrameCount () |
void | updateDone () |
bool | isOK () const |
Return true if the file was opened successfully and no error has subsequently occurred. More... | |
sv_frame_t | getFrameCount () const |
Return the number of audio sample frames (i.e. More... | |
int | getChannelCount () const |
Return the number of channels in the file. More... | |
sv_samplerate_t | getSampleRate () const |
Return the samplerate at which the file is being read. More... | |
virtual sv_samplerate_t | getNativeRate () const |
Return the native samplerate of the file. More... | |
virtual TagMap | getTags () const |
virtual std::vector< floatvec_t > | getDeInterleavedFrames (sv_frame_t start, sv_frame_t count) const |
Return de-interleaved samples for count frames from index start. More... | |
Static Public Member Functions | |
static void | getSupportedExtensions (std::set< QString > &extensions) |
static bool | supportsExtension (QString ext) |
static bool | supportsContentType (QString type) |
static bool | supports (FileSource &source) |
Protected Member Functions | |
floatvec_t | getInterleavedFramesUnnormalised (sv_frame_t start, sv_frame_t count) const |
float | getMax () const |
Protected Attributes | |
SF_INFO | m_fileInfo |
SNDFILE * | m_file |
FileSource | m_source |
QString | m_path |
QString | m_error |
QString | m_title |
QString | m_maker |
bool | m_seekable |
QMutex | m_mutex |
floatvec_t | m_buffer |
sv_frame_t | m_lastStart |
sv_frame_t | m_lastCount |
Normalisation | m_normalisation |
float | m_max |
bool | m_updating |
sv_frame_t | m_frameCount |
int | m_channelCount |
sv_samplerate_t | m_sampleRate |
Detailed Description
Reader for audio files using libsndfile.
This is typically intended for seekable file types that can be read directly (e.g. WAV, AIFF etc).
Compressed files supported by libsndfile (e.g. Ogg, FLAC) should normally be read using DecodingWavFileReader instead (which decodes to an intermediate cached file).
Definition at line 41 of file WavFileReader.h.
Member Typedef Documentation
|
inherited |
Return any tag pairs picked up from the audio file.
See also getTitle and getMaker, and note that a reader which does not implement getTags may still return values from those.
Definition at line 117 of file AudioFileReader.h.
Member Enumeration Documentation
|
strong |
Enumerator | |
---|---|
None | |
Peak |
Definition at line 44 of file WavFileReader.h.
Constructor & Destructor Documentation
WavFileReader::WavFileReader | ( | FileSource | source, |
bool | fileUpdating = false , |
||
Normalisation | normalise = Normalisation::None |
||
) |
Definition at line 28 of file WavFileReader.cpp.
References getMax(), AudioFileReader::m_channelCount, m_error, m_file, m_fileInfo, AudioFileReader::m_frameCount, m_maker, m_max, m_normalisation, m_path, AudioFileReader::m_sampleRate, m_seekable, m_title, m_updating, None, and SVDEBUG.
|
virtual |
Definition at line 112 of file WavFileReader.cpp.
References m_file.
Member Function Documentation
|
inlineoverridevirtual |
Return the location of the audio data in the reader (as passed in to the FileSource constructor, for example).
This might be a remote URL.
See also getLocalFilename().
Implements AudioFileReader.
Definition at line 51 of file WavFileReader.h.
References FileSource::getLocation(), and m_source.
|
inlineoverridevirtual |
If isOK() is false, return an error string.
Reimplemented from AudioFileReader.
Definition at line 52 of file WavFileReader.h.
References m_error.
Referenced by DecodingWavFileReader::DecodingWavFileReader(), WritableWaveFileModel::init(), CodedAudioFileReader::initialiseDecodeCache(), and WritableWaveFileModel::normaliseToTarget().
|
inlineoverridevirtual |
Return the title of the work in the audio file, if known.
This may be implemented by subclasses that support file tagging. This is not the same thing as the file name.
Implements AudioFileReader.
Definition at line 54 of file WavFileReader.h.
References m_title.
Referenced by DecodingWavFileReader::DecodingWavFileReader().
|
inlineoverridevirtual |
Return the "maker" of the work in the audio file, if known.
This could represent almost anything (band, composer, conductor, artist etc).
Implements AudioFileReader.
Definition at line 55 of file WavFileReader.h.
References m_maker.
Referenced by DecodingWavFileReader::DecodingWavFileReader().
|
inlineoverridevirtual |
Return the local file path of the audio data.
This is the filesystem location most likely to contain readable audio data, but it may be in a different place or format from the originally specified location - for example, if the file has been retrieved and decoded, then it will be the (possibly temporary) decode target file.
This returns a non-empty value only if there is some local filename that contains exactly the audio data being provided by this reader. In some cases this may not exist, for example when a file has been resampled or normalised directly into a memory buffer. In this case, return an empty string.
See also getLocation().
Implements AudioFileReader.
Definition at line 57 of file WavFileReader.h.
References m_path.
|
inlineoverridevirtual |
Return true if this file supports fast seek and random access.
Typically this will be true for uncompressed formats and false for compressed ones.
Implements AudioFileReader.
Definition at line 59 of file WavFileReader.h.
References getInterleavedFrames(), getSupportedExtensions(), m_seekable, supports(), supportsContentType(), and supportsExtension().
|
overridevirtual |
Must be safe to call from multiple threads with different arguments on the same object at the same time.
Implements AudioFileReader.
Definition at line 164 of file WavFileReader.cpp.
References getInterleavedFramesUnnormalised(), in_range_for(), m_max, m_normalisation, and None.
Referenced by DecodingWavFileReader::DecodingWavFileReader(), CodedAudioFileReader::getInterleavedFrames(), getMax(), isQuicklySeekable(), and WritableWaveFileModel::normaliseToTarget().
|
static |
Definition at line 280 of file WavFileReader.cpp.
Referenced by AudioFileReaderFactory::getKnownExtensions(), DecodingWavFileReader::getSupportedExtensions(), isQuicklySeekable(), and supportsExtension().
|
static |
Definition at line 309 of file WavFileReader.cpp.
References getSupportedExtensions().
Referenced by isQuicklySeekable(), supports(), and DecodingWavFileReader::supportsExtension().
|
static |
Definition at line 317 of file WavFileReader.cpp.
Referenced by isQuicklySeekable(), supports(), and DecodingWavFileReader::supportsContentType().
|
static |
Definition at line 325 of file WavFileReader.cpp.
References FileSource::getContentType(), FileSource::getExtension(), supportsContentType(), and supportsExtension().
Referenced by AudioFileReaderFactory::createReader(), isQuicklySeekable(), AudioFileReaderFactory::isSupported(), and DecodingWavFileReader::supports().
|
inlineoverridevirtual |
Return a percentage value indicating how far through decoding the audio file we are.
This should be implemented by subclasses that will not know exactly how long the audio file is (in sample frames) until it has been completely decoded. A reader that initialises the frame count directly within its constructor should always return 100 from this.
Reimplemented from AudioFileReader.
Definition at line 72 of file WavFileReader.h.
|
inlineoverridevirtual |
Return true if decoding is still in progress and the frame count may change.
Reimplemented from AudioFileReader.
Definition at line 74 of file WavFileReader.h.
References m_updating, updateDone(), and updateFrameCount().
void WavFileReader::updateFrameCount | ( | ) |
Definition at line 120 of file WavFileReader.cpp.
References AudioFileReader::frameCountChanged(), AudioFileReader::m_channelCount, m_file, m_fileInfo, AudioFileReader::m_frameCount, m_mutex, m_path, AudioFileReader::m_sampleRate, and SVDEBUG.
Referenced by WritableWaveFileModel::addSamples(), CodedAudioFileReader::finishDecodeCache(), isUpdating(), CodedAudioFileReader::pushCacheWriteBufferMaybe(), updateDone(), and WritableWaveFileModel::updateModel().
void WavFileReader::updateDone | ( | ) |
Definition at line 154 of file WavFileReader.cpp.
References getMax(), m_max, m_normalisation, m_updating, None, and updateFrameCount().
Referenced by isUpdating(), and WritableWaveFileModel::writeComplete().
|
protected |
Definition at line 180 of file WavFileReader.cpp.
References HitCount::hit(), m_buffer, AudioFileReader::m_channelCount, m_file, m_fileInfo, m_lastCount, m_lastStart, m_mutex, HitCount::miss(), and HitCount::partial().
Referenced by getInterleavedFrames().
|
protected |
Definition at line 243 of file WavFileReader.cpp.
References getInterleavedFrames(), AudioFileReader::m_channelCount, m_file, AudioFileReader::m_frameCount, and SVDEBUG.
Referenced by updateDone(), and WavFileReader().
|
inlineinherited |
Return true if the file was opened successfully and no error has subsequently occurred.
Definition at line 38 of file AudioFileReader.h.
References AudioFileReader::m_channelCount.
Referenced by AudioFileReaderFactory::createReader(), DecodingWavFileReader::DecodingWavFileReader(), AudioFileSizeEstimator::estimate(), ReadOnlyWaveFileModel::getData(), CodedAudioFileReader::getInterleavedFrames(), ReadOnlyWaveFileModel::getMultiChannelData(), CodedAudioFileReader::initialiseDecodeCache(), and ReadOnlyWaveFileModel::isOK().
|
inlineinherited |
Return the number of audio sample frames (i.e.
samples per channel) in the file.
Definition at line 49 of file AudioFileReader.h.
References AudioFileReader::m_frameCount.
Referenced by DecodingWavFileReader::addBlock(), DecodingWavFileReader::DecodingWavFileReader(), AudioFileSizeEstimator::estimate(), ReadOnlyWaveFileModel::getFrameCount(), and WritableWaveFileModel::normaliseToTarget().
|
inlineinherited |
Return the number of channels in the file.
Definition at line 54 of file AudioFileReader.h.
References AudioFileReader::m_channelCount.
Referenced by WritableWaveFileModel::addSamples(), DecodingWavFileReader::DecodingWavFileReader(), AudioFileSizeEstimator::estimate(), ReadOnlyWaveFileModel::getChannelCount(), and AudioFileReader::getDeInterleavedFrames().
|
inlineinherited |
Return the samplerate at which the file is being read.
This is the rate requested when the file was opened, which may differ from the native rate of the file (in which case the file will be resampled as it is read).
Definition at line 62 of file AudioFileReader.h.
References AudioFileReader::m_sampleRate.
Referenced by AudioFileReaderFactory::createReader(), DecodingWavFileReader::DecodingWavFileReader(), AudioFileSizeEstimator::estimate(), ReadOnlyWaveFileModel::getSampleRate(), and ReadOnlyWaveFileModel::ReadOnlyWaveFileModel().
|
inlinevirtualinherited |
Return the native samplerate of the file.
This will differ from getSampleRate() if the file is being resampled because it was requested to open at a different rate from native.
Reimplemented in CodedAudioFileReader.
Definition at line 69 of file AudioFileReader.h.
References AudioFileReader::getLocalFilename(), AudioFileReader::getLocation(), AudioFileReader::getMaker(), AudioFileReader::getTitle(), and AudioFileReader::m_sampleRate.
Referenced by ReadOnlyWaveFileModel::getNativeRate().
|
inlinevirtualinherited |
Reimplemented in MP3FileReader.
Definition at line 118 of file AudioFileReader.h.
References AudioFileReader::isQuicklySeekable().
|
virtualinherited |
Return de-interleaved samples for count frames from index start.
Implemented in this class (it calls getInterleavedFrames and de-interleaves). The resulting vector will contain getChannelCount() sample blocks of count samples each (or fewer if end of file is reached).
Definition at line 21 of file AudioFileReader.cpp.
References AudioFileReader::getChannelCount(), and AudioFileReader::getInterleavedFrames().
Referenced by AudioFileReader::isUpdating().
|
signalinherited |
Referenced by AudioFileReader::isUpdating(), and updateFrameCount().
Member Data Documentation
|
protected |
Definition at line 80 of file WavFileReader.h.
Referenced by getInterleavedFramesUnnormalised(), updateFrameCount(), and WavFileReader().
|
protected |
Definition at line 81 of file WavFileReader.h.
Referenced by getInterleavedFramesUnnormalised(), getMax(), updateFrameCount(), WavFileReader(), and ~WavFileReader().
|
protected |
Definition at line 83 of file WavFileReader.h.
Referenced by getLocation().
|
protected |
Definition at line 84 of file WavFileReader.h.
Referenced by getLocalFilename(), updateFrameCount(), and WavFileReader().
|
protected |
Definition at line 85 of file WavFileReader.h.
Referenced by getError(), and WavFileReader().
|
protected |
Definition at line 86 of file WavFileReader.h.
Referenced by getTitle(), and WavFileReader().
|
protected |
Definition at line 87 of file WavFileReader.h.
Referenced by getMaker(), and WavFileReader().
|
protected |
Definition at line 89 of file WavFileReader.h.
Referenced by isQuicklySeekable(), and WavFileReader().
|
mutableprotected |
Definition at line 91 of file WavFileReader.h.
Referenced by getInterleavedFramesUnnormalised(), and updateFrameCount().
|
mutableprotected |
Definition at line 92 of file WavFileReader.h.
Referenced by getInterleavedFramesUnnormalised().
|
mutableprotected |
Definition at line 93 of file WavFileReader.h.
Referenced by getInterleavedFramesUnnormalised().
|
mutableprotected |
Definition at line 94 of file WavFileReader.h.
Referenced by getInterleavedFramesUnnormalised().
|
protected |
Definition at line 96 of file WavFileReader.h.
Referenced by getInterleavedFrames(), updateDone(), and WavFileReader().
|
protected |
Definition at line 97 of file WavFileReader.h.
Referenced by getInterleavedFrames(), updateDone(), and WavFileReader().
|
protected |
Definition at line 99 of file WavFileReader.h.
Referenced by isUpdating(), updateDone(), and WavFileReader().
|
protectedinherited |
Definition at line 169 of file AudioFileReader.h.
Referenced by MP3FileReader::accept(), CodedAudioFileReader::CodedAudioFileReader(), CodedAudioFileReader::finishDecodeCache(), AudioFileReader::getFrameCount(), getMax(), CodedAudioFileReader::pushBufferNonResampling(), CodedAudioFileReader::pushBufferResampling(), updateFrameCount(), and WavFileReader().
|
protectedinherited |
Definition at line 170 of file AudioFileReader.h.
Referenced by MP3FileReader::accept(), CodedAudioFileReader::addSamplesToDecodeCache(), BQAFileReader::BQAFileReader(), DecodingWavFileReader::DecodingWavFileReader(), AudioFileReader::getChannelCount(), CodedAudioFileReader::getInterleavedFrames(), getInterleavedFramesUnnormalised(), getMax(), CodedAudioFileReader::initialiseDecodeCache(), AudioFileReader::isOK(), MP3FileReader::MP3FileReader(), CodedAudioFileReader::pushBufferNonResampling(), CodedAudioFileReader::pushBufferResampling(), CodedAudioFileReader::pushCacheWriteBufferMaybe(), updateFrameCount(), and WavFileReader().
|
protectedinherited |
Definition at line 171 of file AudioFileReader.h.
Referenced by MP3FileReader::accept(), DecodingWavFileReader::addBlock(), CodedAudioFileReader::CodedAudioFileReader(), AudioFileReader::getNativeRate(), AudioFileReader::getSampleRate(), CodedAudioFileReader::initialiseDecodeCache(), MP3FileReader::MP3FileReader(), CodedAudioFileReader::pushBuffer(), updateFrameCount(), and WavFileReader().
The documentation for this class was generated from the following files:
Generated by
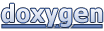