svcore
1.9
|
AudioFileReaderFactory.cpp
Go to the documentation of this file.
77 SVDEBUG << "AudioFileReaderFactory: url \"" << source.getLocation() << "\": requested rate: " << params.targetRate << (params.targetRate == 0 ? " (use source rate)" : "") << endl;
78 SVDEBUG << "AudioFileReaderFactory: local filename \"" << source.getLocalFilename() << "\", content type \"" << source.getContentType() << "\"" << endl;
81 SVDEBUG << "AudioFileReaderFactory::createReader(\"" << source.getLocation() << "\": Failed to retrieve source (transmission error?): " << source.getErrorString() << endl;
86 SVDEBUG << "AudioFileReaderFactory::createReader(\"" << source.getLocation() << "\": Source not found" << endl;
135 SVDEBUG << "AudioFileReaderFactory: Checking whether any reader officially handles this source" << endl;
137 SVDEBUG << "AudioFileReaderFactory: Source not officially handled by any reader, trying again with each reader in turn"
182 SVDEBUG << "AudioFileReaderFactory: WAV file reader rate: " << reader->getSampleRate() << ", normalised " << normalised << ", seekable " << reader->isQuicklySeekable() << ", in memory " << (cacheMode == CodedAudioFileReader::CacheInMemory) << ", creating decoding reader" << endl;
Definition: MP3FileReader.h:31
static bool isSupported(FileSource source)
Return true if the given source has a file extension that indicates a supported file type...
Definition: AudioFileReaderFactory.cpp:54
Definition: StorageAdviser.h:36
static void getSupportedExtensions(std::set< QString > &extensions)
Definition: MP3FileReader.cpp:614
QString getLocation() const
Return the location filename or URL as passed to the constructor.
Definition: FileSource.cpp:616
static void getSupportedExtensions(std::set< QString > &extensions)
Definition: BQAFileReader.cpp:179
Definition: ProgressReporter.h:22
Definition: StorageAdviser.h:45
Definition: AudioFileReader.h:27
Definition: DecodingWavFileReader.h:29
GaplessMode
How the MP3FileReader should handle leading and trailing gaps.
Definition: MP3FileReader.h:41
QString getLocalFilename() const
Return the name of the local file this FileSource refers to.
Definition: FileSource.cpp:622
static Recommendation recommend(Criteria criteria, size_t minimumSize, size_t maximumSize)
Recommend where to store some data, given certain storage and recall criteria.
Definition: StorageAdviser.cpp:67
Do not trim any samples.
static QString getKnownExtensions()
Return the file extensions that we have audio file readers for, in a format suitable for use with QFi...
Definition: AudioFileReaderFactory.cpp:33
QString getErrorString() const
Return an error message, if isOK() is false.
Definition: FileSource.cpp:650
static AudioFileReader * createReader(FileSource source, Parameters parameters, ProgressReporter *reporter=0)
Return an audio file reader initialised to the file at the given path, or NULL if no suitable reader ...
Definition: AudioFileReaderFactory.cpp:71
Trim unwanted samples from the start and end of the decoded audio.
FileSource is a class used to refer to the contents of a file that may be either local or at a remote...
Definition: FileSource.h:59
static void getSupportedExtensions(std::set< QString > &extensions)
Definition: WavFileReader.cpp:280
QString getContentType() const
Return the MIME content type of this file, if known.
Definition: FileSource.cpp:634
Definition: StorageAdviser.h:46
bool isOK() const
Return true if the file was opened successfully and no error has subsequently occurred.
Definition: AudioFileReader.h:38
Audio file reader using bqaudiostream library AudioReadStream classes.
Definition: BQAFileReader.h:32
static sv_frame_t estimate(FileSource source, sv_samplerate_t targetRate=0)
Return an estimate of the number of samples (across all channels) in the given audio file...
Definition: AudioFileSizeEstimator.cpp:24
virtual bool isQuicklySeekable() const =0
Return true if this file supports fast seek and random access.
sv_samplerate_t getSampleRate() const
Return the samplerate at which the file is being read.
Definition: AudioFileReader.h:62
bool isOK() const
Return true if the FileSource object is valid and neither error nor cancellation occurred while retri...
Definition: FileSource.cpp:586
Generated by
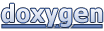