svcore
1.9
|
AudioFileSizeEstimator.cpp
Go to the documentation of this file.
QString getLocalFilename() const
Return the name of the local file this FileSource refers to.
Definition: FileSource.cpp:622
void waitForData()
Block on a sub-event-loop until the whole of the data has been retrieved (if it is remote)...
Definition: FileSource.cpp:570
FileSource is a class used to refer to the contents of a file that may be either local or at a remote...
Definition: FileSource.h:59
bool isOK() const
Return true if the file was opened successfully and no error has subsequently occurred.
Definition: AudioFileReader.h:38
static sv_frame_t estimate(FileSource source, sv_samplerate_t targetRate=0)
Return an estimate of the number of samples (across all channels) in the given audio file...
Definition: AudioFileSizeEstimator.cpp:24
QString getExtension() const
Return the file extension for this file, if any.
Definition: FileSource.cpp:640
sv_frame_t getFrameCount() const
Return the number of audio sample frames (i.e.
Definition: AudioFileReader.h:49
sv_samplerate_t getSampleRate() const
Return the samplerate at which the file is being read.
Definition: AudioFileReader.h:62
int getChannelCount() const
Return the number of channels in the file.
Definition: AudioFileReader.h:54
bool isOK() const
Return true if the FileSource object is valid and neither error nor cancellation occurred while retri...
Definition: FileSource.cpp:586
Generated by
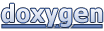