svcore
1.9
|
AudioFileReader.h
Go to the documentation of this file.
virtual sv_samplerate_t getNativeRate() const
Return the native samplerate of the file.
Definition: AudioFileReader.h:69
virtual QString getMaker() const =0
Return the "maker" of the work in the audio file, if known.
virtual floatvec_t getInterleavedFrames(sv_frame_t start, sv_frame_t count) const =0
Return interleaved samples for count frames from index start.
Definition: AudioFileReader.h:27
virtual QString getTitle() const =0
Return the title of the work in the audio file, if known.
virtual bool isUpdating() const
Return true if decoding is still in progress and the frame count may change.
Definition: AudioFileReader.h:141
std::vector< float, breakfastquay::StlAllocator< float > > floatvec_t
Definition: BaseTypes.h:53
std::map< QString, QString > TagMap
Return any tag pairs picked up from the audio file.
Definition: AudioFileReader.h:117
virtual QString getError() const
If isOK() is false, return an error string.
Definition: AudioFileReader.h:43
void frameCountChanged()
virtual QString getLocation() const =0
Return the location of the audio data in the reader (as passed in to the FileSource constructor...
virtual QString getLocalFilename() const =0
Return the local file path of the audio data.
virtual std::vector< floatvec_t > getDeInterleavedFrames(sv_frame_t start, sv_frame_t count) const
Return de-interleaved samples for count frames from index start.
Definition: AudioFileReader.cpp:21
bool isOK() const
Return true if the file was opened successfully and no error has subsequently occurred.
Definition: AudioFileReader.h:38
virtual int getDecodeCompletion() const
Return a percentage value indicating how far through decoding the audio file we are.
Definition: AudioFileReader.h:135
virtual bool isQuicklySeekable() const =0
Return true if this file supports fast seek and random access.
sv_frame_t getFrameCount() const
Return the number of audio sample frames (i.e.
Definition: AudioFileReader.h:49
sv_samplerate_t getSampleRate() const
Return the samplerate at which the file is being read.
Definition: AudioFileReader.h:62
int getChannelCount() const
Return the number of channels in the file.
Definition: AudioFileReader.h:54
Generated by
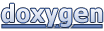