svcore
1.9
|
#include <AudioFileReader.h>
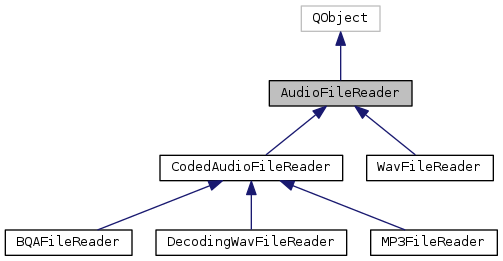
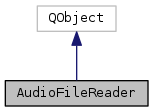
Public Types | |
typedef std::map< QString, QString > | TagMap |
Return any tag pairs picked up from the audio file. More... | |
Signals | |
void | frameCountChanged () |
Public Member Functions | |
virtual | ~AudioFileReader () |
bool | isOK () const |
Return true if the file was opened successfully and no error has subsequently occurred. More... | |
virtual QString | getError () const |
If isOK() is false, return an error string. More... | |
sv_frame_t | getFrameCount () const |
Return the number of audio sample frames (i.e. More... | |
int | getChannelCount () const |
Return the number of channels in the file. More... | |
sv_samplerate_t | getSampleRate () const |
Return the samplerate at which the file is being read. More... | |
virtual sv_samplerate_t | getNativeRate () const |
Return the native samplerate of the file. More... | |
virtual QString | getLocation () const =0 |
Return the location of the audio data in the reader (as passed in to the FileSource constructor, for example). More... | |
virtual QString | getLocalFilename () const =0 |
Return the local file path of the audio data. More... | |
virtual QString | getTitle () const =0 |
Return the title of the work in the audio file, if known. More... | |
virtual QString | getMaker () const =0 |
Return the "maker" of the work in the audio file, if known. More... | |
virtual TagMap | getTags () const |
virtual bool | isQuicklySeekable () const =0 |
Return true if this file supports fast seek and random access. More... | |
virtual int | getDecodeCompletion () const |
Return a percentage value indicating how far through decoding the audio file we are. More... | |
virtual bool | isUpdating () const |
Return true if decoding is still in progress and the frame count may change. More... | |
virtual floatvec_t | getInterleavedFrames (sv_frame_t start, sv_frame_t count) const =0 |
Return interleaved samples for count frames from index start. More... | |
virtual std::vector< floatvec_t > | getDeInterleavedFrames (sv_frame_t start, sv_frame_t count) const |
Return de-interleaved samples for count frames from index start. More... | |
Protected Attributes | |
sv_frame_t | m_frameCount |
int | m_channelCount |
sv_samplerate_t | m_sampleRate |
Detailed Description
Definition at line 27 of file AudioFileReader.h.
Member Typedef Documentation
typedef std::map<QString, QString> AudioFileReader::TagMap |
Return any tag pairs picked up from the audio file.
See also getTitle and getMaker, and note that a reader which does not implement getTags may still return values from those.
Definition at line 117 of file AudioFileReader.h.
Constructor & Destructor Documentation
|
inlinevirtual |
Definition at line 32 of file AudioFileReader.h.
Member Function Documentation
|
inline |
Return true if the file was opened successfully and no error has subsequently occurred.
Definition at line 38 of file AudioFileReader.h.
References m_channelCount.
Referenced by AudioFileReaderFactory::createReader(), DecodingWavFileReader::DecodingWavFileReader(), AudioFileSizeEstimator::estimate(), ReadOnlyWaveFileModel::getData(), CodedAudioFileReader::getInterleavedFrames(), ReadOnlyWaveFileModel::getMultiChannelData(), CodedAudioFileReader::initialiseDecodeCache(), and ReadOnlyWaveFileModel::isOK().
|
inlinevirtual |
If isOK() is false, return an error string.
Reimplemented in MP3FileReader, WavFileReader, BQAFileReader, and DecodingWavFileReader.
Definition at line 43 of file AudioFileReader.h.
|
inline |
Return the number of audio sample frames (i.e.
samples per channel) in the file.
Definition at line 49 of file AudioFileReader.h.
References m_frameCount.
Referenced by DecodingWavFileReader::addBlock(), DecodingWavFileReader::DecodingWavFileReader(), AudioFileSizeEstimator::estimate(), ReadOnlyWaveFileModel::getFrameCount(), and WritableWaveFileModel::normaliseToTarget().
|
inline |
Return the number of channels in the file.
Definition at line 54 of file AudioFileReader.h.
References m_channelCount.
Referenced by WritableWaveFileModel::addSamples(), DecodingWavFileReader::DecodingWavFileReader(), AudioFileSizeEstimator::estimate(), ReadOnlyWaveFileModel::getChannelCount(), and getDeInterleavedFrames().
|
inline |
Return the samplerate at which the file is being read.
This is the rate requested when the file was opened, which may differ from the native rate of the file (in which case the file will be resampled as it is read).
Definition at line 62 of file AudioFileReader.h.
References m_sampleRate.
Referenced by AudioFileReaderFactory::createReader(), DecodingWavFileReader::DecodingWavFileReader(), AudioFileSizeEstimator::estimate(), ReadOnlyWaveFileModel::getSampleRate(), and ReadOnlyWaveFileModel::ReadOnlyWaveFileModel().
|
inlinevirtual |
Return the native samplerate of the file.
This will differ from getSampleRate() if the file is being resampled because it was requested to open at a different rate from native.
Reimplemented in CodedAudioFileReader.
Definition at line 69 of file AudioFileReader.h.
References getLocalFilename(), getLocation(), getMaker(), getTitle(), and m_sampleRate.
Referenced by ReadOnlyWaveFileModel::getNativeRate().
|
pure virtual |
Return the location of the audio data in the reader (as passed in to the FileSource constructor, for example).
This might be a remote URL.
See also getLocalFilename().
Implemented in MP3FileReader, WavFileReader, BQAFileReader, and DecodingWavFileReader.
Referenced by ReadOnlyWaveFileModel::getLocation(), and getNativeRate().
|
pure virtual |
Return the local file path of the audio data.
This is the filesystem location most likely to contain readable audio data, but it may be in a different place or format from the originally specified location - for example, if the file has been retrieved and decoded, then it will be the (possibly temporary) decode target file.
This returns a non-empty value only if there is some local filename that contains exactly the audio data being provided by this reader. In some cases this may not exist, for example when a file has been resampled or normalised directly into a memory buffer. In this case, return an empty string.
See also getLocation().
Implemented in CodedAudioFileReader, and WavFileReader.
Referenced by ReadOnlyWaveFileModel::getLocalFilename(), and getNativeRate().
|
pure virtual |
Return the title of the work in the audio file, if known.
This may be implemented by subclasses that support file tagging. This is not the same thing as the file name.
Implemented in MP3FileReader, WavFileReader, BQAFileReader, and DecodingWavFileReader.
Referenced by getNativeRate(), ReadOnlyWaveFileModel::getTitle(), and ReadOnlyWaveFileModel::ReadOnlyWaveFileModel().
|
pure virtual |
Return the "maker" of the work in the audio file, if known.
This could represent almost anything (band, composer, conductor, artist etc).
Implemented in MP3FileReader, WavFileReader, BQAFileReader, and DecodingWavFileReader.
Referenced by ReadOnlyWaveFileModel::getMaker(), and getNativeRate().
|
inlinevirtual |
Reimplemented in MP3FileReader.
Definition at line 118 of file AudioFileReader.h.
References isQuicklySeekable().
|
pure virtual |
Return true if this file supports fast seek and random access.
Typically this will be true for uncompressed formats and false for compressed ones.
Implemented in CodedAudioFileReader, and WavFileReader.
Referenced by AudioFileReaderFactory::createReader(), and getTags().
|
inlinevirtual |
Return a percentage value indicating how far through decoding the audio file we are.
This should be implemented by subclasses that will not know exactly how long the audio file is (in sample frames) until it has been completely decoded. A reader that initialises the frame count directly within its constructor should always return 100 from this.
Reimplemented in MP3FileReader, WavFileReader, BQAFileReader, and DecodingWavFileReader.
Definition at line 135 of file AudioFileReader.h.
Referenced by ReadOnlyWaveFileModel::isReady().
|
inlinevirtual |
Return true if decoding is still in progress and the frame count may change.
Reimplemented in MP3FileReader, WavFileReader, BQAFileReader, and DecodingWavFileReader.
Definition at line 141 of file AudioFileReader.h.
References frameCountChanged(), getDeInterleavedFrames(), and getInterleavedFrames().
Referenced by ReadOnlyWaveFileModel::isReady().
|
pure virtual |
Return interleaved samples for count frames from index start.
The resulting vector will contain count * getChannelCount() samples (or fewer if end of file is reached).
The subclass implementations of this function must be thread-safe – that is, safe to call from multiple threads with different arguments on the same object at the same time.
Implemented in WavFileReader, and CodedAudioFileReader.
Referenced by ReadOnlyWaveFileModel::getData(), getDeInterleavedFrames(), ReadOnlyWaveFileModel::getMultiChannelData(), ReadOnlyWaveFileModel::getSummaries(), and isUpdating().
|
virtual |
Return de-interleaved samples for count frames from index start.
Implemented in this class (it calls getInterleavedFrames and de-interleaves). The resulting vector will contain getChannelCount() sample blocks of count samples each (or fewer if end of file is reached).
Definition at line 21 of file AudioFileReader.cpp.
References getChannelCount(), and getInterleavedFrames().
Referenced by isUpdating().
|
signal |
Referenced by isUpdating(), and WavFileReader::updateFrameCount().
Member Data Documentation
|
protected |
Definition at line 169 of file AudioFileReader.h.
Referenced by MP3FileReader::accept(), CodedAudioFileReader::CodedAudioFileReader(), CodedAudioFileReader::finishDecodeCache(), getFrameCount(), WavFileReader::getMax(), CodedAudioFileReader::pushBufferNonResampling(), CodedAudioFileReader::pushBufferResampling(), WavFileReader::updateFrameCount(), and WavFileReader::WavFileReader().
|
protected |
Definition at line 170 of file AudioFileReader.h.
Referenced by MP3FileReader::accept(), CodedAudioFileReader::addSamplesToDecodeCache(), BQAFileReader::BQAFileReader(), DecodingWavFileReader::DecodingWavFileReader(), getChannelCount(), CodedAudioFileReader::getInterleavedFrames(), WavFileReader::getInterleavedFramesUnnormalised(), WavFileReader::getMax(), CodedAudioFileReader::initialiseDecodeCache(), isOK(), MP3FileReader::MP3FileReader(), CodedAudioFileReader::pushBufferNonResampling(), CodedAudioFileReader::pushBufferResampling(), CodedAudioFileReader::pushCacheWriteBufferMaybe(), WavFileReader::updateFrameCount(), and WavFileReader::WavFileReader().
|
protected |
Definition at line 171 of file AudioFileReader.h.
Referenced by MP3FileReader::accept(), DecodingWavFileReader::addBlock(), CodedAudioFileReader::CodedAudioFileReader(), getNativeRate(), getSampleRate(), CodedAudioFileReader::initialiseDecodeCache(), MP3FileReader::MP3FileReader(), CodedAudioFileReader::pushBuffer(), WavFileReader::updateFrameCount(), and WavFileReader::WavFileReader().
The documentation for this class was generated from the following files:
Generated by
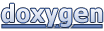