svcore
1.9
|
WavFileReader.cpp
Go to the documentation of this file.
109 SVDEBUG << "WavFileReader: Filename " << m_path << ", frame count " << m_frameCount << ", channel count " << m_channelCount << ", sample rate " << m_sampleRate << ", format " << m_fileInfo.format << ", seekable " << m_fileInfo.seekable << " adjusted to " << m_seekable << ", normalisation " << int(m_normalisation) << endl;
static bool supportsExtension(QString ext)
Definition: WavFileReader.cpp:309
floatvec_t getInterleavedFramesUnnormalised(sv_frame_t start, sv_frame_t count) const
Definition: WavFileReader.cpp:180
static bool supportsContentType(QString type)
Definition: WavFileReader.cpp:317
WavFileReader(FileSource source, bool fileUpdating=false, Normalisation normalise=Normalisation::None)
Definition: WavFileReader.cpp:28
std::vector< float, breakfastquay::StlAllocator< float > > floatvec_t
Definition: BaseTypes.h:53
void frameCountChanged()
FileSource is a class used to refer to the contents of a file that may be either local or at a remote...
Definition: FileSource.h:59
static void getSupportedExtensions(std::set< QString > &extensions)
Definition: WavFileReader.cpp:280
QString getContentType() const
Return the MIME content type of this file, if known.
Definition: FileSource.cpp:634
bool in_range_for(const C &container, T i)
Check whether an integer index is in range for a container, avoiding overflows and signed/unsigned co...
Definition: BaseTypes.h:37
QString getExtension() const
Return the file extension for this file, if any.
Definition: FileSource.cpp:640
floatvec_t getInterleavedFrames(sv_frame_t start, sv_frame_t count) const override
Must be safe to call from multiple threads with different arguments on the same object at the same ti...
Definition: WavFileReader.cpp:164
Generated by
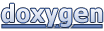