svcore
1.9
|
FileSource is a class used to refer to the contents of a file that may be either local or at a remote location such as a HTTP URL. More...
#include <FileSource.h>
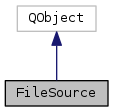
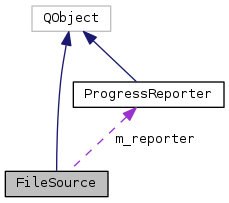
Signals | |
void | progress (int percent) |
Emitted during URL retrieval, when the retrieval progress notches up to a new percentage. More... | |
void | statusAvailable () |
Emitted when the file's existence has been tested and/or response header received. More... | |
void | ready () |
Emitted when the entire file data has been retrieved and the local file is complete (if no error has occurred). More... | |
Public Member Functions | |
FileSource (QString fileOrUrl, ProgressReporter *reporter=0, QString preferredContentType="") | |
Construct a FileSource using the given local file path or URL. More... | |
FileSource (QUrl url, ProgressReporter *reporter=0) | |
Construct a FileSource using the given remote URL. More... | |
FileSource (const FileSource &) | |
virtual | ~FileSource () |
void | waitForStatus () |
Block on a sub-event-loop until the availability of the file or remote URL is known. More... | |
void | waitForData () |
Block on a sub-event-loop until the whole of the data has been retrieved (if it is remote). More... | |
bool | isOK () const |
Return true if the FileSource object is valid and neither error nor cancellation occurred while retrieving the file or remote URL. More... | |
bool | isAvailable () |
Return true if the file or remote URL exists. More... | |
bool | isDone () const |
Return true if the entire file has been retrieved and is available. More... | |
bool | wasCancelled () const |
Return true if the operation was cancelled by the user through the ProgressReporter interface. More... | |
bool | isResource () const |
Return true if this FileSource is referring to a QRC resource. More... | |
bool | isRemote () const |
Return true if this FileSource is referring to a remote URL. More... | |
QString | getLocation () const |
Return the location filename or URL as passed to the constructor. More... | |
QString | getLocalFilename () const |
Return the name of the local file this FileSource refers to. More... | |
QString | getBasename () const |
Return the base name, i.e. More... | |
QString | getContentType () const |
Return the MIME content type of this file, if known. More... | |
QString | getExtension () const |
Return the file extension for this file, if any. More... | |
QString | getErrorString () const |
Return an error message, if isOK() is false. More... | |
void | setLeaveLocalFile (bool leave) |
Specify whether any local, cached file should remain on disc after this FileSource has been destroyed. More... | |
Static Public Member Functions | |
static bool | isRemote (QString fileOrUrl) |
Return true if the given filename or URL refers to a remote URL. More... | |
static bool | canHandleScheme (QUrl url) |
Return true if FileSource can handle the retrieval scheme for the given URL (or if the URL is for a local file). More... | |
static void | debugReport () |
Print some stats, if FileSource was compiled with debugging. More... | |
Protected Types | |
typedef std::map< QUrl, int > | RemoteRefCountMap |
typedef std::map< QUrl, QString > | RemoteLocalMap |
Protected Slots | |
void | metaDataChanged () |
void | readyRead () |
void | replyFailed (QNetworkReply::NetworkError) |
void | replyFinished () |
void | downloadProgress (qint64 done, qint64 total) |
void | cancelled () |
Protected Member Functions | |
FileSource & | operator= (const FileSource &) |
void | init () |
void | initRemote () |
void | cleanup () |
bool | createCacheFile () |
void | deleteCacheFile () |
Protected Attributes | |
QString | m_rawFileOrUrl |
QUrl | m_url |
QFile * | m_localFile |
QNetworkReply * | m_reply |
QString | m_localFilename |
QString | m_errorString |
QString | m_contentType |
QString | m_preferredContentType |
bool | m_ok |
bool | m_cancelled |
int | m_lastStatus |
bool | m_resource |
bool | m_remote |
bool | m_done |
bool | m_leaveLocalFile |
ProgressReporter * | m_reporter |
bool | m_refCounted |
Static Protected Attributes | |
static RemoteRefCountMap | m_refCountMap |
static RemoteLocalMap | m_remoteLocalMap |
static QMutex | m_mapMutex |
static QMutex | m_fileCreationMutex |
static int | m_count = 0 |
Detailed Description
FileSource is a class used to refer to the contents of a file that may be either local or at a remote location such as a HTTP URL.
When a FileSource object is constructed, the file or URL passed to its constructor is tested for validity, and if it refers to a remote HTTP or FTP location it is retrieved asynchronously (the Qt event loop must be running) and cached locally. You can then query a local path for the file and refer to that as a normal filename.
Use isAvailable() to test whether the file or remote URL exists, and isOK() to test for internal validity or transmission errors. Call waitForStatus() to block until the availability and validity of the URL have been established so that isAvailable may be called, and waitForData() to block until the entire file has been cached.
FileSource handles reference counting for cache files. You can construct many FileSource objects with the same URL and you will receive the same cached file for each; it is also reasonable to pass FileSource objects by value. FileSource only makes sense for stateless URLs that result in the same data on each request.
Cached files share a lifetime with their "owning" FileSource objects; when the last FileSource referring to a given URL is deleted or goes out of scope, its cached file (if any) is also removed.
Definition at line 59 of file FileSource.h.
Member Typedef Documentation
|
protected |
Definition at line 254 of file FileSource.h.
|
protected |
Definition at line 255 of file FileSource.h.
Constructor & Destructor Documentation
FileSource::FileSource | ( | QString | fileOrUrl, |
ProgressReporter * | reporter = 0 , |
||
QString | preferredContentType = "" |
||
) |
Construct a FileSource using the given local file path or URL.
The URL may be raw or encoded.
If a ProgressReporter is provided, it will be updated with progress status. Note that the progress() signal will also be emitted regularly during retrieval, even if no reporter is supplied here. Caller retains ownership of the reporter object.
Definition at line 90 of file FileSource.cpp.
References canHandleScheme(), deleteCacheFile(), init(), isAvailable(), isRemote(), m_done, m_errorString, m_lastStatus, m_ok, m_resource, m_url, ready(), statusAvailable(), and waitForStatus().
FileSource::FileSource | ( | QUrl | url, |
ProgressReporter * | reporter = 0 |
||
) |
Construct a FileSource using the given remote URL.
If a ProgressReporter is provided, it will be updated with progress status. Note that the progress() signal will also be emitted regularly during retrieval, even if no reporter is supplied here. Caller retains ownership of the reporter object.
Definition at line 183 of file FileSource.cpp.
References canHandleScheme(), init(), m_errorString, and m_url.
FileSource::FileSource | ( | const FileSource & | rf | ) |
Definition at line 215 of file FileSource.cpp.
References canHandleScheme(), isRemote(), m_done, m_errorString, m_lastStatus, m_localFilename, m_mapMutex, m_ok, m_refCounted, m_refCountMap, m_remoteLocalMap, and m_url.
|
virtual |
Definition at line 273 of file FileSource.cpp.
References cleanup(), deleteCacheFile(), isRemote(), m_leaveLocalFile, and m_url.
Member Function Documentation
void FileSource::waitForStatus | ( | ) |
Block on a sub-event-loop until the availability of the file or remote URL is known.
Definition at line 561 of file FileSource.cpp.
References m_done, m_lastStatus, and m_ok.
Referenced by FileSource(), and isAvailable().
void FileSource::waitForData | ( | ) |
Block on a sub-event-loop until the whole of the data has been retrieved (if it is remote).
Definition at line 570 of file FileSource.cpp.
Referenced by AudioFileSizeEstimator::estimate(), RDFImporterImpl::getDataModelsAudio(), PlaylistFileReader::init(), ReadOnlyWaveFileModel::ReadOnlyWaveFileModel(), and CachedFile::retrieve().
bool FileSource::isOK | ( | ) | const |
Return true if the FileSource object is valid and neither error nor cancellation occurred while retrieving the file or remote URL.
Non-existence of the file or URL is not an error – call isAvailable() to test for that.
Definition at line 586 of file FileSource.cpp.
References m_ok.
Referenced by AudioFileReaderFactory::createReader(), AudioFileSizeEstimator::estimate(), ReadOnlyWaveFileModel::ReadOnlyWaveFileModel(), and CachedFile::retrieve().
bool FileSource::isAvailable | ( | ) |
Return true if the file or remote URL exists.
This may block on a sub-event-loop (calling waitForStatus) if the status is not yet available.
Definition at line 544 of file FileSource.cpp.
References m_lastStatus, m_ok, and waitForStatus().
Referenced by AudioFileReaderFactory::createReader(), FileSource(), RDFImporterImpl::getDataModelsAudio(), PlaylistFileReader::init(), TextTest::isApparentTextDocument(), PlaylistFileReader::PlaylistFileReader(), and CachedFile::retrieve().
bool FileSource::isDone | ( | ) | const |
Return true if the entire file has been retrieved and is available.
Definition at line 592 of file FileSource.cpp.
References m_done.
bool FileSource::wasCancelled | ( | ) | const |
Return true if the operation was cancelled by the user through the ProgressReporter interface.
Note that the cancelled() signal will have been emitted, and isOK() will also return false in this case.
Definition at line 598 of file FileSource.cpp.
References m_cancelled.
bool FileSource::isResource | ( | ) | const |
Return true if this FileSource is referring to a QRC resource.
Definition at line 604 of file FileSource.cpp.
References m_resource.
Referenced by init().
bool FileSource::isRemote | ( | ) | const |
Return true if this FileSource is referring to a remote URL.
Definition at line 610 of file FileSource.cpp.
References m_remote.
Referenced by deleteCacheFile(), FileSource(), PlaylistFileReader::init(), init(), PlaylistFileReader::load(), PluginRDFIndexer::pullURL(), and ~FileSource().
QString FileSource::getLocation | ( | ) | const |
Return the location filename or URL as passed to the constructor.
Definition at line 616 of file FileSource.cpp.
References m_url.
Referenced by AudioFileReaderFactory::createReader(), RDFImporterImpl::getDataModelsAudio(), DecodingWavFileReader::getLocation(), BQAFileReader::getLocation(), WavFileReader::getLocation(), MP3FileReader::getLocation(), PlaylistFileReader::init(), TextTest::isApparentTextDocument(), and PlaylistFileReader::PlaylistFileReader().
QString FileSource::getLocalFilename | ( | ) | const |
Return the name of the local file this FileSource refers to.
This is the filename that should be used when reading normally from the FileSource. If the filename passed to the constructor was a local file, this will return the same filename; otherwise this will be the name of the temporary cache file owned by the FileSource.
Definition at line 622 of file FileSource.cpp.
References m_localFilename.
Referenced by AudioFileReaderFactory::createReader(), AudioFileSizeEstimator::estimate(), RDFImporterImpl::getDataModelsAudio(), PlaylistFileReader::init(), TextTest::isApparentTextDocument(), and CachedFile::retrieve().
QString FileSource::getBasename | ( | ) | const |
Return the base name, i.e.
the final path element (including extension, if any) of the location.
Definition at line 628 of file FileSource.cpp.
References m_localFilename.
QString FileSource::getContentType | ( | ) | const |
Return the MIME content type of this file, if known.
Definition at line 634 of file FileSource.cpp.
References m_contentType.
Referenced by AudioFileReaderFactory::createReader(), BQAFileReader::supports(), WavFileReader::supports(), and MP3FileReader::supports().
QString FileSource::getExtension | ( | ) | const |
Return the file extension for this file, if any.
The returned extension is always lower-case.
Definition at line 640 of file FileSource.cpp.
References m_localFilename, and m_url.
Referenced by AudioFileSizeEstimator::estimate(), PlaylistFileReader::isSupported(), BQAFileReader::supports(), WavFileReader::supports(), and MP3FileReader::supports().
QString FileSource::getErrorString | ( | ) | const |
Return an error message, if isOK() is false.
Definition at line 650 of file FileSource.cpp.
References m_errorString.
Referenced by AudioFileReaderFactory::createReader().
void FileSource::setLeaveLocalFile | ( | bool | leave | ) |
Specify whether any local, cached file should remain on disc after this FileSource has been destroyed.
The default is false (cached files share their FileSource owners' lifespans).
Definition at line 580 of file FileSource.cpp.
References m_leaveLocalFile.
|
static |
Return true if the given filename or URL refers to a remote URL.
Definition at line 525 of file FileSource.cpp.
|
static |
Return true if FileSource can handle the retrieval scheme for the given URL (or if the URL is for a local file).
Definition at line 534 of file FileSource.cpp.
Referenced by FileSource(), and PluginRDFIndexer::pullURL().
|
static |
Print some stats, if FileSource was compiled with debugging.
It's safe to leave a call to this function in release code, as long as FileSource itself is compiled with release flags.
Definition at line 85 of file FileSource.cpp.
|
signal |
Emitted during URL retrieval, when the retrieval progress notches up to a new percentage.
Referenced by downloadProgress(), init(), replyFailed(), and replyFinished().
|
signal |
Emitted when the file's existence has been tested and/or response header received.
Calls to isAvailable() after this has been emitted will not block.
Referenced by FileSource(), and metaDataChanged().
|
signal |
Emitted when the entire file data has been retrieved and the local file is complete (if no error has occurred).
Referenced by FileSource(), replyFailed(), and replyFinished().
|
protectedslot |
Definition at line 662 of file FileSource.cpp.
References cleanup(), deleteCacheFile(), init(), m_contentType, m_done, m_errorString, m_lastStatus, m_localFile, m_refCounted, m_reply, m_url, and statusAvailable().
Referenced by initRemote().
|
protectedslot |
Definition at line 656 of file FileSource.cpp.
References m_localFile, and m_reply.
Referenced by initRemote().
|
protectedslot |
Definition at line 794 of file FileSource.cpp.
References cleanup(), m_done, m_errorString, m_ok, m_reply, progress(), and ready().
Referenced by initRemote().
|
protectedslot |
Definition at line 748 of file FileSource.cpp.
References cleanup(), deleteCacheFile(), m_done, m_errorString, m_lastStatus, m_localFile, m_localFilename, m_ok, m_url, progress(), and ready().
Referenced by initRemote().
|
protectedslot |
|
protectedslot |
Definition at line 737 of file FileSource.cpp.
References cleanup(), m_cancelled, m_done, m_errorString, and m_ok.
Referenced by init().
|
protected |
|
protected |
Definition at line 286 of file FileSource.cpp.
References cancelled(), cleanup(), createCacheFile(), initRemote(), isRemote(), isResource(), m_done, m_lastStatus, m_localFile, m_localFilename, m_mapMutex, m_ok, m_rawFileOrUrl, m_refCounted, m_refCountMap, m_remote, m_remoteLocalMap, m_reporter, m_resource, m_url, progress(), and ProgressReporter::setMessage().
Referenced by FileSource(), and metaDataChanged().
|
protected |
Definition at line 459 of file FileSource.cpp.
References downloadProgress(), m_mapMutex, m_ok, m_preferredContentType, m_reply, m_url, metaDataChanged(), readyRead(), replyFailed(), and replyFinished().
Referenced by init().
|
protected |
Definition at line 501 of file FileSource.cpp.
References m_done, m_localFile, and m_reply.
Referenced by cancelled(), deleteCacheFile(), init(), metaDataChanged(), replyFailed(), replyFinished(), and ~FileSource().
|
protected |
Definition at line 864 of file FileSource.cpp.
References TempDirectory::getInstance(), m_count, m_fileCreationMutex, m_localFilename, m_mapMutex, m_refCounted, m_refCountMap, m_remoteLocalMap, m_url, and DirectoryCreationFailed::what().
Referenced by init().
|
protected |
Definition at line 809 of file FileSource.cpp.
References cleanup(), isRemote(), m_done, m_fileCreationMutex, m_localFilename, m_mapMutex, m_refCounted, m_refCountMap, and m_url.
Referenced by FileSource(), metaDataChanged(), replyFinished(), and ~FileSource().
Member Data Documentation
|
protected |
Definition at line 237 of file FileSource.h.
Referenced by init().
|
protected |
Definition at line 238 of file FileSource.h.
Referenced by createCacheFile(), deleteCacheFile(), FileSource(), getExtension(), getLocation(), init(), initRemote(), metaDataChanged(), replyFinished(), and ~FileSource().
|
protected |
Definition at line 239 of file FileSource.h.
Referenced by cleanup(), init(), metaDataChanged(), readyRead(), and replyFinished().
|
protected |
Definition at line 240 of file FileSource.h.
Referenced by cleanup(), initRemote(), metaDataChanged(), readyRead(), and replyFailed().
|
protected |
Definition at line 241 of file FileSource.h.
Referenced by createCacheFile(), deleteCacheFile(), FileSource(), getBasename(), getExtension(), getLocalFilename(), init(), and replyFinished().
|
protected |
Definition at line 242 of file FileSource.h.
Referenced by cancelled(), FileSource(), getErrorString(), metaDataChanged(), replyFailed(), and replyFinished().
|
protected |
Definition at line 243 of file FileSource.h.
Referenced by getContentType(), and metaDataChanged().
|
protected |
Definition at line 244 of file FileSource.h.
Referenced by initRemote().
|
protected |
Definition at line 245 of file FileSource.h.
Referenced by cancelled(), FileSource(), init(), initRemote(), isAvailable(), isOK(), replyFailed(), replyFinished(), waitForData(), and waitForStatus().
|
protected |
Definition at line 246 of file FileSource.h.
Referenced by cancelled(), and wasCancelled().
|
protected |
Definition at line 247 of file FileSource.h.
Referenced by FileSource(), init(), isAvailable(), metaDataChanged(), replyFinished(), and waitForStatus().
|
protected |
Definition at line 248 of file FileSource.h.
Referenced by FileSource(), init(), and isResource().
|
protected |
Definition at line 249 of file FileSource.h.
Referenced by init(), and isRemote().
|
protected |
Definition at line 250 of file FileSource.h.
Referenced by cancelled(), cleanup(), deleteCacheFile(), FileSource(), init(), isDone(), metaDataChanged(), replyFailed(), replyFinished(), waitForData(), and waitForStatus().
|
protected |
Definition at line 251 of file FileSource.h.
Referenced by setLeaveLocalFile(), and ~FileSource().
|
protected |
Definition at line 252 of file FileSource.h.
Referenced by init().
|
staticprotected |
Definition at line 256 of file FileSource.h.
Referenced by createCacheFile(), deleteCacheFile(), FileSource(), and init().
|
staticprotected |
Definition at line 257 of file FileSource.h.
Referenced by createCacheFile(), FileSource(), and init().
|
staticprotected |
Definition at line 258 of file FileSource.h.
Referenced by createCacheFile(), deleteCacheFile(), FileSource(), init(), and initRemote().
|
protected |
Definition at line 259 of file FileSource.h.
Referenced by createCacheFile(), deleteCacheFile(), FileSource(), init(), and metaDataChanged().
|
staticprotected |
Definition at line 271 of file FileSource.h.
Referenced by createCacheFile(), and deleteCacheFile().
|
staticprotected |
Definition at line 272 of file FileSource.h.
Referenced by createCacheFile().
The documentation for this class was generated from the following files:
Generated by
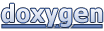