svcore
1.9
|
TextTest.cpp
Go to the documentation of this file.
31 SVDEBUG << "NOTE: TextTest::isApparentTextDocument: Failed to retrieve document from " << source.getLocation() << endl;
37 SVDEBUG << "NOTE: TextTest::isApparentTextDocument: Failed to open local file from " << source.getLocalFilename() << endl;
56 SVDEBUG << "NOTE: TextTest::isApparentTextDocument: Document is not UTF-8 and is not XML, rejecting" << endl;
static bool isValidUtf8(const std::string &bytes, bool isTruncated)
Return true if the given byte array contains a valid UTF-8 sequence, false if not.
Definition: StringBits.cpp:189
QString getLocation() const
Return the location filename or URL as passed to the constructor.
Definition: FileSource.cpp:616
QString getLocalFilename() const
Return the name of the local file this FileSource refers to.
Definition: FileSource.cpp:622
static bool isApparentTextDocument(FileSource)
Return true if the source appears to point to a text format of some kind (could be CSV...
Definition: TextTest.cpp:24
FileSource is a class used to refer to the contents of a file that may be either local or at a remote...
Definition: FileSource.h:59
Generated by
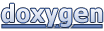