svcore
1.9
|
CachedFile.cpp
Go to the documentation of this file.
135 SVDEBUG << "CachedFile::check: Local file does not exist, making a note that it hasn't been retrieved" << endl;
160 SVCERR << "CachedFile::check: Retrieval failed, will try again later (using existing file for now)" << endl;
208 SVDEBUG << "CachedFile::retrieve: ERROR: FileSource reported success, but local temporary file \"" << tempName << "\" does not exist" << endl;
215 SVCERR << "CachedFile::retrieve: ERROR: Failed to remove previous copy of cached file at \"" << m_localFilename << "\"" << endl;
225 SVCERR << "CachedFile::retrieve: ERROR: Failed to copy newly retrieved file from \"" << tempName << "\" to \"" << m_localFilename << "\"" << endl;
229 SVDEBUG << "CachedFile::retrieve: Successfully copied newly retrieved file \"" << tempName << "\" to its home at \"" << m_localFilename << "\"" << endl;
CachedFile(QString fileOrUrl, ProgressReporter *reporter=0, QString preferredContentType="")
Definition: CachedFile.cpp:71
Definition: ProgressReporter.h:22
QString getLocalFilename() const
Return the name of the local file this FileSource refers to.
Definition: FileSource.cpp:622
static OriginLocalFilenameMap m_knownGoodCaches
Definition: CachedFile.h:59
void waitForData()
Block on a sub-event-loop until the whole of the data has been retrieved (if it is remote)...
Definition: FileSource.cpp:570
std::map< QString, QString > OriginLocalFilenameMap
Definition: CachedFile.h:58
static QString getLocalFilenameFor(QUrl url)
Definition: CachedFile.cpp:39
FileSource is a class used to refer to the contents of a file that may be either local or at a remote...
Definition: FileSource.h:59
QString getContainingPath()
Return the path of the directory in which the temporary directory has been or will be created...
Definition: TempDirectory.cpp:59
Definition: Exceptions.h:47
void updateLastRetrieval(bool successful)
Definition: CachedFile.cpp:250
bool isOK() const
Return true if the FileSource object is valid and neither error nor cancellation occurred while retri...
Definition: FileSource.cpp:586
Generated by
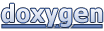