svcore
1.9
|
Exceptions.h
Go to the documentation of this file.
virtual ~DirectoryCreationFailed()
Definition: Exceptions.h:51
Definition: Exceptions.h:81
Definition: Exceptions.h:36
virtual ~InsufficientDiscSpace()
Definition: Exceptions.h:87
Definition: Exceptions.h:69
Definition: Exceptions.h:100
Definition: Exceptions.h:58
Definition: Exceptions.h:47
Definition: Exceptions.h:25
Generated by
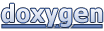