svcore
1.9
|
ReadOnlyWaveFileModel.cpp
Go to the documentation of this file.
175 SVCERR << "ReadOnlyWaveFileModel(" << objectName() << ")::isReady(): ready = " << ready << ", m_fillThread = " << m_fillThread << ", m_lastFillExtent = " << m_lastFillExtent << ", end frame = " << getEndFrame() << ", start frame = " << getStartFrame() << ", c = " << c << ", completion = " << *completion << endl;
177 SVCERR << "ReadOnlyWaveFileModel(" << objectName() << ")::isReady(): ready = " << ready << ", m_fillThread = " << m_fillThread << ", m_lastFillExtent = " << m_lastFillExtent << ", end frame = " << getEndFrame() << ", start frame = " << getStartFrame() << ", c = " << c << ", completion not requested" << endl;
261 cout << "ReadOnlyWaveFileModel::getData[" << this << "]: " << channel << ", " << start << ", " << count << endl;
322 cout << "ReadOnlyWaveFileModel::getData[" << this << "]: " << fromchannel << "," << tochannel << ", " << start << ", " << count << endl;
490 cerr << "blockSize is " << blockSize << ", cacheBlock " << cacheBlock << ", start " << start << ", count " << count << " (frame count " << getFrameCount() << "), power is " << power << ", div is " << div << ", startIndex " << startIndex << ", endIndex " << endIndex << endl;
592 SVCERR << "ReadOnlyWaveFileModel(" << objectName() << ")::fillCache: started fill thread" << endl;
602 SVCERR << "ReadOnlyWaveFileModel(" << objectName() << ")::fillTimerTimedOut: extent = " << fillExtent << endl;
610 SVCERR << "ReadOnlyWaveFileModel(" << objectName() << ")::fillTimerTimedOut: no thread" << endl;
628 SVCERR << "ReadOnlyWaveFileModel(" << objectName() << ")::cacheFilled, about to emit things" << endl;
657 SVCERR << "ReadOnlyWaveFileModel(" << objectName() << ")::fill: Waiting for channels..." << endl;
689 cout << "ReadOnlyWaveFileModel(" << m_model.objectName() << ")::fill inner loop: frame = " << frame << ", count = " << m_frameCount << ", blocksize " << readBlockSize << endl;
783 SVCERR << "ReadOnlyWaveFileModel(" << m_model.objectName() << "): Cache type " << cacheType << " now contains " << m_model.m_cache[cacheType].size() << " ranges" << endl;
void setMax(float max)
Definition: RangeSummarisableTimeValueModel.h:55
virtual sv_samplerate_t getNativeRate() const
Return the native samplerate of the file.
Definition: AudioFileReader.h:69
void toXml(QTextStream &stream, QString indent="", QString extraAttributes="") const override
Stream this exportable object out to XML on a text stream.
Definition: Model.cpp:204
static PlayParameterRepository * getInstance()
Definition: PlayParameterRepository.cpp:26
std::atomic< bool > m_exiting
Definition: ReadOnlyWaveFileModel.h:142
sv_frame_t m_lastDirectReadStart
Definition: ReadOnlyWaveFileModel.h:146
void fillTimerTimedOut()
Definition: ReadOnlyWaveFileModel.cpp:597
bool isReady(int *) const override
Return true if the model has finished loading or calculating all its data, for a model that is capabl...
Definition: ReadOnlyWaveFileModel.cpp:146
virtual QString getMaker() const =0
Return the "maker" of the work in the audio file, if known.
QMutex m_directReadMutex
Definition: ReadOnlyWaveFileModel.h:148
sv_frame_t getFrameCount() const override
Definition: ReadOnlyWaveFileModel.cpp:184
RangeCacheFillThread * m_fillThread
Definition: ReadOnlyWaveFileModel.h:138
QString getLocalFilename() const
Definition: ReadOnlyWaveFileModel.cpp:237
virtual floatvec_t getInterleavedFrames(sv_frame_t start, sv_frame_t count) const =0
Return interleaved samples for count frames from index start.
std::vector< Range > RangeBlock
Definition: RangeSummarisableTimeValueModel.h:76
Definition: AudioFileReader.h:27
virtual int getNearestBlockSize(int requestedBlockSize, int &type, int &power, RoundingDirection dir=RoundNearest) const
Definition: PowerOfSqrtTwoZoomConstraint.cpp:51
float absmean() const
Definition: RangeSummarisableTimeValueModel.h:52
sv_samplerate_t getNativeRate() const override
Return the frame rate of the underlying material, if the model itself has already been resampled...
Definition: ReadOnlyWaveFileModel.cpp:205
virtual QString getTitle() const =0
Return the title of the work in the audio file, if known.
If the reader supports threaded decoding, it will be used and the file will be decoded in a backgroun...
QString getMaker() const override
Return the "artist" or "maker" of the model, if known.
Definition: ReadOnlyWaveFileModel.cpp:223
virtual int getMinCachePower() const
Definition: PowerOfSqrtTwoZoomConstraint.h:28
virtual bool isUpdating() const
Return true if decoding is still in progress and the frame count may change.
Definition: AudioFileReader.h:141
void waitForData()
Block on a sub-event-loop until the whole of the data has been retrieved (if it is remote)...
Definition: FileSource.cpp:570
bool isOK() const override
Return true if the model was constructed successfully.
Definition: ReadOnlyWaveFileModel.cpp:140
~ReadOnlyWaveFileModel()
Definition: ReadOnlyWaveFileModel.cpp:122
sv_frame_t getEndFrame() const
Return the audio frame at the end of the model, i.e.
Definition: Model.h:87
std::vector< float, breakfastquay::StlAllocator< float > > floatvec_t
Definition: BaseTypes.h:53
float max() const
Definition: RangeSummarisableTimeValueModel.h:51
void sample(float s)
Definition: RangeSummarisableTimeValueModel.h:58
int getSummaryBlockSize(int desired) const override
Definition: ReadOnlyWaveFileModel.cpp:377
bool getNormaliseAudio() const
True if audio files should be loaded with normalisation (max == 1)
Definition: Preferences.h:82
static AudioFileReader * createReader(FileSource source, Parameters parameters, ProgressReporter *reporter=0)
Return an audio file reader initialised to the file at the given path, or NULL if no suitable reader ...
Definition: AudioFileReaderFactory.cpp:71
static PowerOfSqrtTwoZoomConstraint m_zoomConstraint
Definition: ReadOnlyWaveFileModel.h:143
Any encoder delay and padding found in file metadata will be compensated for, giving gapless decoding...
Definition: ZoomConstraint.h:38
virtual QString getLocation() const =0
Return the location of the audio data in the reader (as passed in to the FileSource constructor...
No delay compensation will happen and the results will be equivalent to the behaviour of audio reader...
QString getTitle() const override
Return the "work title" of the model, if known.
Definition: ReadOnlyWaveFileModel.cpp:214
Normalise file data to abs(max) == 1.0.
void setMin(float min)
Definition: RangeSummarisableTimeValueModel.h:54
FileSource is a class used to refer to the contents of a file that may be either local or at a remote...
Definition: FileSource.h:59
virtual QString getLocalFilename() const =0
Return the local file path of the audio data.
float min() const
Definition: RangeSummarisableTimeValueModel.h:50
void setAbsmean(float absmean)
Definition: RangeSummarisableTimeValueModel.h:56
QString getLocation() const override
Return the location of the data in this model (e.g.
Definition: ReadOnlyWaveFileModel.cpp:230
bool in_range_for(const C &container, T i)
Check whether an integer index is in range for a container, avoiding overflows and signed/unsigned co...
Definition: BaseTypes.h:37
void modelChangedWithin(ModelId myId, sv_frame_t startFrame, sv_frame_t endFrame)
Emitted when a model has been edited (or more data retrieved from cache, in the case of a cached mode...
bool isOK() const
Return true if the file was opened successfully and no error has subsequently occurred.
Definition: AudioFileReader.h:38
Range getSummary(int channel, sv_frame_t start, sv_frame_t count) const override
Return the range from the given start frame, corresponding to the given number of underlying sample f...
Definition: ReadOnlyWaveFileModel.cpp:525
void toXml(QTextStream &out, QString indent="", QString extraAttributes="") const override
Stream this exportable object out to XML on a text stream.
Definition: ReadOnlyWaveFileModel.cpp:789
sv_frame_t m_lastFillExtent
Definition: ReadOnlyWaveFileModel.h:140
void addPlayable(int id, const Playable *)
Register a playable.
Definition: PlayParameterRepository.cpp:36
sv_frame_t getStartFrame() const override
Return the first audio frame spanned by the model.
Definition: ReadOnlyWaveFileModel.h:81
void run() override
Definition: ReadOnlyWaveFileModel.cpp:638
ReadOnlyWaveFileModel(FileSource source, sv_samplerate_t targetRate=0)
Construct a WaveFileModel from a source path and optional resampling target rate. ...
Definition: ReadOnlyWaveFileModel.cpp:43
virtual int getDecodeCompletion() const
Return a percentage value indicating how far through decoding the audio file we are.
Definition: AudioFileReader.h:135
sv_frame_t getFrameCount() const
Return the number of audio sample frames (i.e.
Definition: AudioFileReader.h:49
Definition: Preferences.h:23
sv_frame_t getFillExtent() const
Definition: ReadOnlyWaveFileModel.h:118
sv_frame_t m_lastDirectReadCount
Definition: ReadOnlyWaveFileModel.h:147
sv_samplerate_t getSampleRate() const override
Return the frame rate in frames per second.
Definition: ReadOnlyWaveFileModel.cpp:198
Do not normalise file data.
int getChannelCount() const override
Return the number of distinct channels for this model.
Definition: ReadOnlyWaveFileModel.cpp:191
floatvec_t getData(int channel, sv_frame_t start, sv_frame_t count) const override
Get the specified set of samples from the given channel of the model in single-precision floating-poi...
Definition: ReadOnlyWaveFileModel.cpp:249
void modelChanged(ModelId myId)
Emitted when a model has been edited (or more data retrieved from cache, in the case of a cached mode...
void getSummaries(int channel, sv_frame_t start, sv_frame_t count, RangeBlock &ranges, int &blockSize) const override
Return ranges from the given start frame, corresponding to the given number of underlying sample fram...
Definition: ReadOnlyWaveFileModel.cpp:394
sv_samplerate_t getSampleRate() const
Return the samplerate at which the file is being read.
Definition: AudioFileReader.h:62
bool getUseGaplessMode() const
True if mp3 files should be loaded "gaplessly", i.e. compensating for encoder/decoder delay and paddi...
Definition: Preferences.h:79
int getChannelCount() const
Return the number of channels in the file.
Definition: AudioFileReader.h:54
std::vector< floatvec_t > getMultiChannelData(int fromchannel, int tochannel, sv_frame_t start, sv_frame_t count) const override
Get the specified set of samples from given contiguous range of channels of the model in single-preci...
Definition: ReadOnlyWaveFileModel.cpp:313
bool isOK() const
Return true if the FileSource object is valid and neither error nor cancellation occurred while retri...
Definition: FileSource.cpp:586
Generated by
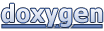