svcore
1.9
|
Model.cpp
Go to the documentation of this file.
208 stream << QString("<model id=\"%1\" name=\"%2\" sampleRate=\"%3\" start=\"%4\" end=\"%5\" %6/>\n")
void alignmentCompletionChanged(ModelId myId)
Emitted when the completion percentage changes for the calculation of this model's alignment model...
void toXml(QTextStream &stream, QString indent="", QString extraAttributes="") const override
Stream this exportable object out to XML on a text stream.
Definition: Model.cpp:204
virtual sv_frame_t alignFromReference(sv_frame_t referenceFrame) const
Return the frame number in this model that corresponds to the given frame number of the reference mod...
Definition: Model.cpp:116
virtual void setAlignment(ModelId alignmentModel)
Specify an alignment between this model's timeline and that of a reference model. ...
Definition: Model.cpp:45
virtual int getAlignmentCompletion() const
Return the completion percentage for the alignment model: 100 if there is no alignment model or it ha...
Definition: Model.cpp:141
void alignmentModelCompletionChanged(ModelId)
Definition: Model.cpp:66
virtual QString getLocation() const
Return the location of the data in this model (e.g.
Definition: Model.cpp:195
virtual QString getMaker() const
Return the "artist" or "maker" of the model, if known.
Definition: Model.cpp:186
sv_frame_t getEndFrame() const
Return the audio frame at the end of the model, i.e.
Definition: Model.h:87
virtual const ModelId getAlignmentReference() const
Return the reference model for the current alignment timeline, if any.
Definition: Model.cpp:79
virtual sv_samplerate_t getSampleRate() const =0
Return the frame rate in frames per second.
void completionChanged(ModelId myId)
Emitted when some internal processing has advanced a stage, but the model has not changed externally...
ExportId getExportId() const
Return the numerical export identifier for this object.
Definition: XmlExportable.cpp:71
virtual void setSourceModel(ModelId model)
Set the source model for this model.
Definition: Model.cpp:31
virtual sv_frame_t getStartFrame() const =0
Return the first audio frame spanned by the model.
virtual QString getTitle() const
Return the "work title" of the model, if known.
Definition: Model.cpp:177
virtual const ModelId getAlignment() const
Retrieve the alignment model for this model.
Definition: Model.cpp:72
virtual sv_frame_t alignToReference(sv_frame_t frame) const
Return the frame number of the reference model that corresponds to the given frame number in this mod...
Definition: Model.cpp:88
Definition: ById.h:115
Generated by
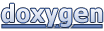