svcore
1.9
|
XmlExportable.cpp
Go to the documentation of this file.
virtual void toXml(QTextStream &stream, QString indent="", QString extraAttributes="") const =0
Stream this exportable object out to XML on a text stream.
virtual QString toXmlString(QString indent="", QString extraAttributes="") const
Convert this exportable object to XML in a string.
Definition: XmlExportable.cpp:25
static QString encodeColour(int r, int g, int b)
Definition: XmlExportable.cpp:54
ExportId getExportId() const
Return the numerical export identifier for this object.
Definition: XmlExportable.cpp:71
Generated by
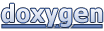