svcore
1.9
|
PowerOfSqrtTwoZoomConstraint.cpp
Go to the documentation of this file.
102 // SVCERR << "Testing base " << base << " (i = " << i << ", power = " << power << ", type = " << type << ")" << endl;
virtual ZoomLevel getMaxZoomLevel() const
Return the maximum zoom level within range for this constraint.
Definition: ZoomConstraint.h:81
virtual int getNearestBlockSize(int requestedBlockSize, int &type, int &power, RoundingDirection dir=RoundNearest) const
Definition: PowerOfSqrtTwoZoomConstraint.cpp:51
virtual ZoomLevel getMinZoomLevel() const
Return the minimum zoom level within range for this constraint.
Definition: ZoomConstraint.h:71
virtual int getMinCachePower() const
Definition: PowerOfSqrtTwoZoomConstraint.h:28
Definition: ZoomConstraint.h:40
Definition: ZoomConstraint.h:38
Definition: ZoomConstraint.h:39
Definition: ZoomLevel.h:29
virtual ZoomLevel getNearestZoomLevel(ZoomLevel requested, RoundingDirection dir=RoundNearest) const override
Given an "ideal" zoom level (frames per pixel or pixels per frame) for a given zoom level...
Definition: PowerOfSqrtTwoZoomConstraint.cpp:25
Generated by
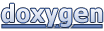