svcore
1.9
|
ZoomConstraint.h
Go to the documentation of this file.
virtual ZoomLevel getMaxZoomLevel() const
Return the maximum zoom level within range for this constraint.
Definition: ZoomConstraint.h:81
Definition: ZoomLevel.h:30
virtual ZoomLevel getMinZoomLevel() const
Return the minimum zoom level within range for this constraint.
Definition: ZoomConstraint.h:71
Definition: ZoomConstraint.h:40
virtual ZoomLevel getNearestZoomLevel(ZoomLevel requestedZoomLevel, RoundingDirection=RoundNearest) const
Given an "ideal" zoom level (frames per pixel or pixels per frame) for a given zoom level...
Definition: ZoomConstraint.h:53
Definition: ZoomConstraint.h:38
Definition: ZoomConstraint.h:39
ZoomConstraint is a simple interface that describes a limitation on the available zoom sizes for a vi...
Definition: ZoomConstraint.h:32
Definition: ZoomLevel.h:29
Generated by
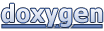