svcore
1.9
|
RangeSummarisableTimeValueModel.h
Go to the documentation of this file.
void setMax(float max)
Definition: RangeSummarisableTimeValueModel.h:55
virtual int getSummaryBlockSize(int desired) const =0
float m_min
Definition: RangeSummarisableTimeValueModel.h:71
RangeSummarisableTimeValueModel()
Definition: RangeSummarisableTimeValueModel.h:37
std::vector< Range > RangeBlock
Definition: RangeSummarisableTimeValueModel.h:76
float absmean() const
Definition: RangeSummarisableTimeValueModel.h:52
Range(float min, float max, float absmean)
Definition: RangeSummarisableTimeValueModel.h:44
bool m_new
Definition: RangeSummarisableTimeValueModel.h:70
float m_absmean
Definition: RangeSummarisableTimeValueModel.h:73
QString getTypeName() const override
Return the type of the model.
Definition: RangeSummarisableTimeValueModel.h:102
virtual void getSummaries(int channel, sv_frame_t start, sv_frame_t count, RangeBlock &ranges, int &blockSize) const =0
Return ranges from the given start frame, corresponding to the given number of underlying sample fram...
float max() const
Definition: RangeSummarisableTimeValueModel.h:51
void sample(float s)
Definition: RangeSummarisableTimeValueModel.h:58
float m_max
Definition: RangeSummarisableTimeValueModel.h:72
Range()
Definition: RangeSummarisableTimeValueModel.h:42
void setMin(float min)
Definition: RangeSummarisableTimeValueModel.h:54
float min() const
Definition: RangeSummarisableTimeValueModel.h:50
void setAbsmean(float absmean)
Definition: RangeSummarisableTimeValueModel.h:56
Range & operator=(const Range &)=default
Base class for models containing dense two-dimensional data (value against time). ...
Definition: DenseTimeValueModel.h:30
Base class for models containing dense two-dimensional data (value against time) that may be meaningf...
Definition: RangeSummarisableTimeValueModel.h:32
virtual Range getSummary(int channel, sv_frame_t start, sv_frame_t count) const =0
Return the range from the given start frame, corresponding to the given number of underlying sample f...
Generated by
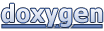