svcore
1.9
|
DenseTimeValueModel.h
Go to the documentation of this file.
virtual int getChannelCount() const =0
Return the number of distinct channels for this model.
virtual std::vector< floatvec_t > getMultiChannelData(int fromchannel, int tochannel, sv_frame_t start, sv_frame_t count) const =0
Get the specified set of samples from given contiguous range of channels of the model in single-preci...
virtual floatvec_t getData(int channel, sv_frame_t start, sv_frame_t count) const =0
Get the specified set of samples from the given channel of the model in single-precision floating-poi...
virtual float getValueMinimum() const =0
Return the minimum possible value found in this model type.
QVector< QVector< QString > > toStringExportRows(DataExportOptions options, sv_frame_t startFrame, sv_frame_t duration) const override
Emit events starting within the given range as string rows ready for conversion to an e...
Definition: DenseTimeValueModel.cpp:34
std::vector< float, breakfastquay::StlAllocator< float > > floatvec_t
Definition: BaseTypes.h:53
QString getTypeName() const override
Return the type of the model.
Definition: DenseTimeValueModel.h:93
Model is the base class for all data models that represent any sort of data on a time scale based on ...
Definition: Model.h:51
virtual ~DenseTimeValueModel()
Definition: DenseTimeValueModel.h:37
virtual float getValueMaximum() const =0
Return the minimum possible value found in this model type.
Base class for models containing dense two-dimensional data (value against time). ...
Definition: DenseTimeValueModel.h:30
QVector< QString > getStringExportHeaders(DataExportOptions options) const override
Return a label for each column that would be written by toStringExportRows.
Definition: DenseTimeValueModel.cpp:23
QString getDefaultPlayClipId() const override
Definition: DenseTimeValueModel.h:83
Generated by
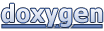