svcore
1.9
|
ReadOnlyWaveFileModel.h
Go to the documentation of this file.
88 std::vector<floatvec_t> getMultiChannelData(int fromchannel, int tochannel, sv_frame_t start, sv_frame_t count) const override;
Definition: WaveFileModel.h:23
sv_frame_t m_frameCount
Definition: ReadOnlyWaveFileModel.h:124
std::atomic< bool > m_exiting
Definition: ReadOnlyWaveFileModel.h:142
sv_frame_t m_lastDirectReadStart
Definition: ReadOnlyWaveFileModel.h:146
sv_frame_t m_fillExtent
Definition: ReadOnlyWaveFileModel.h:123
void fillTimerTimedOut()
Definition: ReadOnlyWaveFileModel.cpp:597
bool isReady(int *) const override
Return true if the model has finished loading or calculating all its data, for a model that is capabl...
Definition: ReadOnlyWaveFileModel.cpp:146
const ZoomConstraint * getZoomConstraint() const override
If this model imposes a zoom constraint, i.e.
Definition: ReadOnlyWaveFileModel.h:65
QMutex m_directReadMutex
Definition: ReadOnlyWaveFileModel.h:148
sv_frame_t getFrameCount() const override
Definition: ReadOnlyWaveFileModel.cpp:184
RangeCacheFillThread * m_fillThread
Definition: ReadOnlyWaveFileModel.h:138
QString getLocalFilename() const
Definition: ReadOnlyWaveFileModel.cpp:237
std::vector< Range > RangeBlock
Definition: RangeSummarisableTimeValueModel.h:76
Definition: AudioFileReader.h:27
ReadOnlyWaveFileModel & m_model
Definition: ReadOnlyWaveFileModel.h:122
int getCompletion() const override
Return an estimated percentage value showing how far through any background operation used to calcula...
Definition: ReadOnlyWaveFileModel.h:59
sv_frame_t getTrueEndFrame() const override
Return the audio frame at the end of the model.
Definition: ReadOnlyWaveFileModel.h:82
sv_samplerate_t getNativeRate() const override
Return the frame rate of the underlying material, if the model itself has already been resampled...
Definition: ReadOnlyWaveFileModel.cpp:205
QString getMaker() const override
Return the "artist" or "maker" of the model, if known.
Definition: ReadOnlyWaveFileModel.cpp:223
void initialize()
bool isOK() const override
Return true if the model was constructed successfully.
Definition: ReadOnlyWaveFileModel.cpp:140
~ReadOnlyWaveFileModel()
Definition: ReadOnlyWaveFileModel.cpp:122
std::vector< float, breakfastquay::StlAllocator< float > > floatvec_t
Definition: BaseTypes.h:53
int getSummaryBlockSize(int desired) const override
Definition: ReadOnlyWaveFileModel.cpp:377
RangeCacheFillThread(ReadOnlyWaveFileModel &model)
Definition: ReadOnlyWaveFileModel.h:114
static PowerOfSqrtTwoZoomConstraint m_zoomConstraint
Definition: ReadOnlyWaveFileModel.h:143
float getValueMaximum() const override
Return the minimum possible value found in this model type.
Definition: ReadOnlyWaveFileModel.h:79
QString getTitle() const override
Return the "work title" of the model, if known.
Definition: ReadOnlyWaveFileModel.cpp:214
FileSource is a class used to refer to the contents of a file that may be either local or at a remote...
Definition: FileSource.h:59
float getValueMinimum() const override
Return the minimum possible value found in this model type.
Definition: ReadOnlyWaveFileModel.h:78
QString getTypeName() const override
Return the type of the model.
Definition: ReadOnlyWaveFileModel.h:98
QString getLocation() const override
Return the location of the data in this model (e.g.
Definition: ReadOnlyWaveFileModel.cpp:230
Range getSummary(int channel, sv_frame_t start, sv_frame_t count) const override
Return the range from the given start frame, corresponding to the given number of underlying sample f...
Definition: ReadOnlyWaveFileModel.cpp:525
void toXml(QTextStream &out, QString indent="", QString extraAttributes="") const override
Stream this exportable object out to XML on a text stream.
Definition: ReadOnlyWaveFileModel.cpp:789
Definition: Thread.h:24
sv_frame_t m_lastFillExtent
Definition: ReadOnlyWaveFileModel.h:140
sv_frame_t getStartFrame() const override
Return the first audio frame spanned by the model.
Definition: ReadOnlyWaveFileModel.h:81
void run() override
Definition: ReadOnlyWaveFileModel.cpp:638
ZoomConstraint is a simple interface that describes a limitation on the available zoom sizes for a vi...
Definition: ZoomConstraint.h:32
ReadOnlyWaveFileModel(FileSource source, sv_samplerate_t targetRate=0)
Construct a WaveFileModel from a source path and optional resampling target rate. ...
Definition: ReadOnlyWaveFileModel.cpp:43
sv_frame_t getFillExtent() const
Definition: ReadOnlyWaveFileModel.h:118
sv_frame_t m_lastDirectReadCount
Definition: ReadOnlyWaveFileModel.h:147
sv_samplerate_t getSampleRate() const override
Return the frame rate in frames per second.
Definition: ReadOnlyWaveFileModel.cpp:198
Definition: ReadOnlyWaveFileModel.h:36
void setStartFrame(sv_frame_t startFrame) override
Definition: ReadOnlyWaveFileModel.h:84
int getChannelCount() const override
Return the number of distinct channels for this model.
Definition: ReadOnlyWaveFileModel.cpp:191
floatvec_t getData(int channel, sv_frame_t start, sv_frame_t count) const override
Get the specified set of samples from the given channel of the model in single-precision floating-poi...
Definition: ReadOnlyWaveFileModel.cpp:249
void getSummaries(int channel, sv_frame_t start, sv_frame_t count, RangeBlock &ranges, int &blockSize) const override
Return ranges from the given start frame, corresponding to the given number of underlying sample fram...
Definition: ReadOnlyWaveFileModel.cpp:394
std::vector< floatvec_t > getMultiChannelData(int fromchannel, int tochannel, sv_frame_t start, sv_frame_t count) const override
Get the specified set of samples from given contiguous range of channels of the model in single-preci...
Definition: ReadOnlyWaveFileModel.cpp:313
Generated by
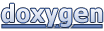