svcore
1.9
|
AudioFileReaderFactory.h
Go to the documentation of this file.
static bool isSupported(FileSource source)
Return true if the given source has a file extension that indicates a supported file type...
Definition: AudioFileReaderFactory.cpp:54
Definition: ProgressReporter.h:22
Definition: AudioFileReader.h:27
static QString getKnownExtensions()
Return the file extensions that we have audio file readers for, in a format suitable for use with QFi...
Definition: AudioFileReaderFactory.cpp:33
static AudioFileReader * createReader(FileSource source, Parameters parameters, ProgressReporter *reporter=0)
Return an audio file reader initialised to the file at the given path, or NULL if no suitable reader ...
Definition: AudioFileReaderFactory.cpp:71
Normalise file data to abs(max) == 1.0.
FileSource is a class used to refer to the contents of a file that may be either local or at a remote...
Definition: FileSource.h:59
Do not normalise file data.
Generated by
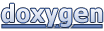