svcore
1.9
|
#include "base/Debug.h"
#include <sys/mman.h>
#include <dlfcn.h>
#include <stdio.h>
#include <cmath>
#include <unistd.h>


Go to the source code of this file.
Macros | |
#define | MLOCK(a, b) ::mlock((a),(b)) |
#define | MUNLOCK(a, b) (::munlock((a),(b)) ? (::perror("munlock failed"), 0) : 0) |
#define | MUNLOCK_SAMPLEBLOCK(a) do { if (!(a).empty()) { const float &b = *(a).begin(); MUNLOCK(&b, (a).capacity() * sizeof(float)); } } while(0); |
#define | DLOPEN(a, b) dlopen((a).toStdString().c_str(),(b)) |
#define | DLSYM(a, b) dlsym((a),(b)) |
#define | DLCLOSE(a) dlclose((a)) |
#define | DLERROR() dlerror() |
#define | ISNAN std::isnan |
#define | ISINF std::isinf |
#define | PLUGIN_GLOB "*.so" |
#define | PATH_SEPARATOR ':' |
#define | DEFAULT_LADSPA_PATH "$HOME/ladspa:$HOME/.ladspa:/usr/local/lib/ladspa:/usr/lib/ladspa" |
#define | DEFAULT_DSSI_PATH "$HOME/dssi:$HOME/.dssi:/usr/local/lib/dssi:/usr/lib/dssi" |
#define | MUNLOCKALL() ::munlockall() |
#define | MBARRIER() SystemMemoryBarrier() |
#define | M_PI 3.14159265358979323846 |
Enumerations | |
enum | ProcessStatus { ProcessRunning, ProcessNotRunning, UnknownProcessStatus } |
Functions | |
void | SystemMemoryBarrier () |
ProcessStatus | GetProcessStatus (int pid) |
void | GetRealMemoryMBAvailable (ssize_t &available, ssize_t &total) |
ssize_t | GetDiscSpaceMBAvailable (const char *path) |
bool | OSReportsDarkThemeActive () |
bool | OSQueryAccentColour (int *r, int *g, int *b) |
void | StoreStartupLocale () |
void | RestoreStartupLocale () |
double | mod (double x, double y) |
float | modf (float x, float y) |
double | princarg (double a) |
float | princargf (float a) |
bool | getEnvUtf8 (std::string variable, std::string &value) |
Return the value of the given environment variable by reference. More... | |
bool | putEnvUtf8 (std::string variable, std::string value) |
Set the value of the given environment variable. More... | |
Macro Definition Documentation
#define MUNLOCK | ( | a, | |
b | |||
) | (::munlock((a),(b)) ? (::perror("munlock failed"), 0) : 0) |
Definition at line 91 of file System.h.
Referenced by ReadOnlyWaveFileModel::RangeCacheFillThread::run().
#define MUNLOCK_SAMPLEBLOCK | ( | a | ) | do { if (!(a).empty()) { const float &b = *(a).begin(); MUNLOCK(&b, (a).capacity() * sizeof(float)); } } while(0); |
#define DLOPEN | ( | a, | |
b | |||
) | dlopen((a).toStdString().c_str(),(b)) |
Definition at line 97 of file System.h.
Referenced by DSSIPluginFactory::discoverPluginsFrom(), LADSPAPluginFactory::discoverPluginsFrom(), NativeVampPluginFactory::getPluginIdentifiers(), NativeVampPluginFactory::instantiatePlugin(), and LADSPAPluginFactory::loadLibrary().
#define DLSYM | ( | a, | |
b | |||
) | dlsym((a),(b)) |
Definition at line 98 of file System.h.
Referenced by DSSIPluginFactory::discoverPluginsFrom(), LADSPAPluginFactory::discoverPluginsFrom(), DSSIPluginFactory::getDSSIDescriptor(), LADSPAPluginFactory::getLADSPADescriptor(), NativeVampPluginFactory::getPluginIdentifiers(), and NativeVampPluginFactory::instantiatePlugin().
#define DLCLOSE | ( | a | ) | dlclose((a)) |
Definition at line 99 of file System.h.
Referenced by DSSIPluginFactory::discoverPluginsFrom(), LADSPAPluginFactory::discoverPluginsFrom(), NativeVampPluginFactory::getPluginIdentifiers(), NativeVampPluginFactory::instantiatePlugin(), NativeVampPluginFactory::pluginDeleted(), and LADSPAPluginFactory::unloadLibrary().
#define DLERROR | ( | ) | dlerror() |
Definition at line 100 of file System.h.
Referenced by DSSIPluginFactory::discoverPluginsFrom(), LADSPAPluginFactory::discoverPluginsFrom(), NativeVampPluginFactory::getPluginIdentifiers(), NativeVampPluginFactory::instantiatePlugin(), and LADSPAPluginFactory::loadLibrary().
#define ISNAN std::isnan |
Definition at line 103 of file System.h.
Referenced by RegionModel::add(), SparseTimeValueModel::add(), NoteModel::add(), EditableDenseThreeDimensionalModel::setColumn(), and BasicCompressedDenseThreeDimensionalModel::setColumn().
#define ISINF std::isinf |
Definition at line 104 of file System.h.
Referenced by RegionModel::add(), SparseTimeValueModel::add(), NoteModel::add(), EditableDenseThreeDimensionalModel::setColumn(), and BasicCompressedDenseThreeDimensionalModel::setColumn().
#define PATH_SEPARATOR ':' |
Definition at line 135 of file System.h.
Referenced by DSSIPluginFactory::getPluginPath(), and LADSPAPluginFactory::getPluginPath().
#define DEFAULT_LADSPA_PATH "$HOME/ladspa:$HOME/.ladspa:/usr/local/lib/ladspa:/usr/lib/ladspa" |
Definition at line 137 of file System.h.
Referenced by LADSPAPluginFactory::getPluginPath().
#define DEFAULT_DSSI_PATH "$HOME/dssi:$HOME/.dssi:/usr/local/lib/dssi:/usr/lib/dssi" |
Definition at line 138 of file System.h.
Referenced by DSSIPluginFactory::getPluginPath().
#define MBARRIER | ( | ) | SystemMemoryBarrier() |
#define M_PI 3.14159265358979323846 |
Definition at line 187 of file System.h.
Referenced by Window< T >::cosinewin(), FFTModel::estimateStableFrequency(), FFTModel::getPeakFrequencies(), and princarg().
Enumeration Type Documentation
enum ProcessStatus |
Function Documentation
void SystemMemoryBarrier | ( | ) |
Referenced by GetDiscSpaceMBAvailable().
ProcessStatus GetProcessStatus | ( | int | pid | ) |
Definition at line 84 of file System.cpp.
References ProcessNotRunning, ProcessRunning, and UnknownProcessStatus.
Referenced by TempDirectory::cleanupAbandonedDirectories().
void GetRealMemoryMBAvailable | ( | ssize_t & | available, |
ssize_t & | total | ||
) |
Definition at line 126 of file System.cpp.
Referenced by StorageAdviser::recommend().
ssize_t GetDiscSpaceMBAvailable | ( | const char * | path | ) |
Definition at line 262 of file System.cpp.
References SystemMemoryBarrier().
Referenced by StorageAdviser::recommend().
bool OSReportsDarkThemeActive | ( | ) |
Definition at line 5 of file os-other.cpp.
Referenced by OSQueryAccentColour().
bool OSQueryAccentColour | ( | int * | r, |
int * | g, | ||
int * | b | ||
) |
Definition at line 27 of file os-win10.cpp.
References OSReportsDarkThemeActive().
void StoreStartupLocale | ( | ) |
Definition at line 319 of file System.cpp.
void RestoreStartupLocale | ( | ) |
Definition at line 328 of file System.cpp.
Referenced by RealTimePluginFactory::enumerateAllPlugins(), RealTimePluginFactory::getAllPluginIdentifiers(), and NativeVampPluginFactory::getPluginIdentifiers().
double mod | ( | double | x, |
double | y | ||
) |
Definition at line 337 of file System.cpp.
Referenced by princarg().
float modf | ( | float | x, |
float | y | ||
) |
Definition at line 338 of file System.cpp.
double princarg | ( | double | a | ) |
Definition at line 340 of file System.cpp.
Referenced by FFTModel::estimateStableFrequency(), FFTModel::getPeakFrequencies(), and princargf().
float princargf | ( | float | a | ) |
Definition at line 341 of file System.cpp.
References princarg().
bool getEnvUtf8 | ( | std::string | variable, |
std::string & | value | ||
) |
Return the value of the given environment variable by reference.
Return true if successfully retrieved, false if unset or on error. Both the variable name and the returned value are UTF-8 encoded.
Definition at line 344 of file System.cpp.
References SVCERR.
Referenced by PluginPathSetter::getPaths(), DSSIPluginFactory::getPluginPath(), LADSPAPluginFactory::getPluginPath(), ResourceFinder::getSystemResourcePrefixList(), and PluginPathSetter::initialiseEnvironmentVariables().
bool putEnvUtf8 | ( | std::string | variable, |
std::string | value | ||
) |
Set the value of the given environment variable.
Return true if successfully set, false on error. Both the variable name and the value must be UTF-8 encoded.
Definition at line 407 of file System.cpp.
References SVCERR.
Referenced by PluginPathSetter::initialiseEnvironmentVariables().
Generated by
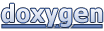