svcore
1.9
|
EditableDenseThreeDimensionalModel.cpp
Go to the documentation of this file.
33 EditableDenseThreeDimensionalModel::EditableDenseThreeDimensionalModel(sv_samplerate_t sampleRate,
405 QString("type=\"dense\" dimensions=\"3\" windowSize=\"%1\" yBinCount=\"%2\" minimum=\"%3\" maximum=\"%4\" dataset=\"%5\" startFrame=\"%6\" %7")
float getMinimumLevel() const override
Return the minimum value of the value in each bin.
Definition: EditableDenseThreeDimensionalModel.cpp:119
sv_frame_t m_startFrame
Definition: EditableDenseThreeDimensionalModel.h:195
virtual void setMinimumLevel(float sz)
Set the minimum value of the value in a bin.
Definition: EditableDenseThreeDimensionalModel.cpp:125
void toXml(QTextStream &stream, QString indent="", QString extraAttributes="") const override
Stream this exportable object out to XML on a text stream.
Definition: Model.cpp:204
bool m_haveExtents
Definition: EditableDenseThreeDimensionalModel.h:201
std::vector< float > m_binValues
Definition: EditableDenseThreeDimensionalModel.h:192
int m_resolution
Definition: EditableDenseThreeDimensionalModel.h:197
virtual void setBinName(int n, QString)
Set the name of bin n.
Definition: EditableDenseThreeDimensionalModel.cpp:243
QString getBinName(int n) const override
Return the name of bin n.
Definition: EditableDenseThreeDimensionalModel.cpp:236
QMutex m_mutex
Definition: EditableDenseThreeDimensionalModel.h:207
sv_frame_t getStartFrame() const override
Return the first audio frame spanned by the model.
Definition: EditableDenseThreeDimensionalModel.cpp:71
int m_completion
Definition: EditableDenseThreeDimensionalModel.h:205
void setCompletion(int completion, bool update=true)
Definition: EditableDenseThreeDimensionalModel.cpp:320
int m_yBinCount
Definition: EditableDenseThreeDimensionalModel.h:198
sv_frame_t m_sinceLastNotifyMin
Definition: EditableDenseThreeDimensionalModel.h:203
int getWidth() const override
Return the number of columns.
Definition: EditableDenseThreeDimensionalModel.cpp:101
float getBinValue(int n) const override
Return the value of bin n, if any.
Definition: EditableDenseThreeDimensionalModel.cpp:264
sv_frame_t m_sinceLastNotifyMax
Definition: EditableDenseThreeDimensionalModel.h:204
EditableDenseThreeDimensionalModel(sv_samplerate_t sampleRate, int resolution, int height, bool notifyOnAdd=true)
Definition: EditableDenseThreeDimensionalModel.cpp:33
int getCompletion() const override
Return an estimated percentage value showing how far through any background operation used to calcula...
Definition: EditableDenseThreeDimensionalModel.cpp:349
virtual void setBinValueUnit(QString unit)
Set the name of the unit of the values return from getBinValue() if any.
Definition: EditableDenseThreeDimensionalModel.cpp:283
QString getBinValueUnit() const override
Obtain the name of the unit of the values returned from getBinValue(), if any.
Definition: EditableDenseThreeDimensionalModel.cpp:277
sv_samplerate_t m_sampleRate
Definition: EditableDenseThreeDimensionalModel.h:196
float m_maximum
Definition: EditableDenseThreeDimensionalModel.h:200
float m_minimum
Definition: EditableDenseThreeDimensionalModel.h:199
float getValueAt(int x, int n) const override
Get a single value, from the n'th bin of the given column.
Definition: EditableDenseThreeDimensionalModel.cpp:160
bool isReady(int *completion=0) const override
Return true if the model has finished loading or calculating all its data, for a model that is capabl...
Definition: EditableDenseThreeDimensionalModel.cpp:58
virtual void setBinNames(std::vector< QString > names)
Set the names of all bins.
Definition: EditableDenseThreeDimensionalModel.cpp:251
virtual void setMaximumLevel(float sz)
Set the maximum value of the value in a bin.
Definition: EditableDenseThreeDimensionalModel.cpp:137
void completionChanged(ModelId myId)
Emitted when some internal processing has advanced a stage, but the model has not changed externally...
static bool shouldUseLogScale(std::vector< double > values)
Estimate whether a set of values would be more properly shown using a logarithmic than a linear scale...
Definition: LogRange.cpp:93
QString m_binValueUnit
Definition: EditableDenseThreeDimensionalModel.h:193
ExportId getExportId() const
Return the numerical export identifier for this object.
Definition: XmlExportable.cpp:71
ValueMatrix m_data
Definition: EditableDenseThreeDimensionalModel.h:189
sv_frame_t getTrueEndFrame() const override
Return the audio frame at the end of the model.
Definition: EditableDenseThreeDimensionalModel.cpp:83
bool in_range_for(const C &container, T i)
Check whether an integer index is in range for a container, avoiding overflows and signed/unsigned co...
Definition: BaseTypes.h:37
void modelChangedWithin(ModelId myId, sv_frame_t startFrame, sv_frame_t endFrame)
Emitted when a model has been edited (or more data retrieved from cache, in the case of a cached mode...
bool shouldUseLogValueScale() const override
Return true if the distribution of values in the bins is such as to suggest a log scale (mapping to c...
Definition: EditableDenseThreeDimensionalModel.cpp:289
sv_samplerate_t getSampleRate() const override
Return the frame rate in frames per second.
Definition: EditableDenseThreeDimensionalModel.cpp:65
QVector< QString > getStringExportHeaders(DataExportOptions options) const override
Return a label for each column that would be written by toStringExportRows.
Definition: EditableDenseThreeDimensionalModel.cpp:355
virtual void setStartFrame(sv_frame_t)
Set the frame offset of the first column.
Definition: EditableDenseThreeDimensionalModel.cpp:77
QVector< QVector< QString > > toStringExportRows(DataExportOptions options, sv_frame_t startFrame, sv_frame_t duration) const override
Emit events starting within the given range as string rows ready for conversion to an e...
Definition: EditableDenseThreeDimensionalModel.cpp:366
bool m_notifyOnAdd
Definition: EditableDenseThreeDimensionalModel.h:202
virtual void setResolution(int sz)
Set the number of sample frames covered by each set of bins.
Definition: EditableDenseThreeDimensionalModel.cpp:95
ColumnOp::Column Column
Definition: DenseThreeDimensionalModel.h:59
virtual void setBinValues(std::vector< float > values)
Set the values of all bins (separate from their labels).
Definition: EditableDenseThreeDimensionalModel.cpp:271
virtual void setHeight(int sz)
Set the number of bins in each column.
Definition: EditableDenseThreeDimensionalModel.cpp:113
void toXml(QTextStream &out, QString indent="", QString extraAttributes="") const override
Stream this exportable object out to XML on a text stream.
Definition: EditableDenseThreeDimensionalModel.cpp:389
bool isOK() const override
Return true if the model was constructed successfully.
Definition: EditableDenseThreeDimensionalModel.cpp:52
float getMaximumLevel() const override
Return the maximum value of the value in each bin.
Definition: EditableDenseThreeDimensionalModel.cpp:131
virtual void setColumn(int x, const Column &values)
Set the entire set of bin values at the given column.
Definition: EditableDenseThreeDimensionalModel.cpp:174
void modelChanged(ModelId myId)
Emitted when a model has been edited (or more data retrieved from cache, in the case of a cached mode...
bool hasBinValues() const override
Return true if the bins have values as well as names.
Definition: EditableDenseThreeDimensionalModel.cpp:258
int getHeight() const override
Return the number of bins in each column.
Definition: EditableDenseThreeDimensionalModel.cpp:107
std::vector< QString > m_binNames
Definition: EditableDenseThreeDimensionalModel.h:191
Column getColumn(int x) const override
Get the set of bin values at the given column.
Definition: EditableDenseThreeDimensionalModel.cpp:143
int getResolution() const override
Return the number of sample frames covered by each set of bins.
Definition: EditableDenseThreeDimensionalModel.cpp:89
Generated by
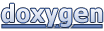