svcore
1.9
|
DenseThreeDimensionalModel.h
Go to the documentation of this file.
virtual int getWidth() const =0
Return the number of columns of bins in the model.
int getColumnCount() const override
Return the number of columns (values/labels/etc per item).
Definition: DenseThreeDimensionalModel.h:133
QString getTypeName() const override
Return the type of the model.
Definition: DenseThreeDimensionalModel.h:121
virtual float getValueAt(int column, int n) const =0
Get the single data point from the n'th bin of the given column.
static RealTime frame2RealTime(sv_frame_t frame, sv_samplerate_t sampleRate)
Convert a sample frame at the given sample rate into a RealTime.
Definition: RealTimeSV.cpp:498
virtual bool shouldUseLogValueScale() const =0
Estimate whether a logarithmic scale might be appropriate for the value scale.
virtual QString getBinValueUnit() const
Obtain the name of the unit of the values returned from getBinValue(), if any.
Definition: DenseThreeDimensionalModel.h:94
bool isEditable() const override
Return true if the model is user-editable, false otherwise.
Definition: DenseThreeDimensionalModel.h:135
Command * getSetDataCommand(int, int, const QVariant &, int) override
Return a command to set the value in the given cell, for the given role, to the contents of the suppl...
Definition: DenseThreeDimensionalModel.h:136
bool isLocalPeak(int x, int y)
Utility function to query whether a given bin is greater than its (vertical) neighbours.
Definition: DenseThreeDimensionalModel.h:106
int getRowCount() const override
Return the number of rows (items) in the model.
Definition: DenseThreeDimensionalModel.h:132
virtual float getMinimumLevel() const =0
Return the minimum permissible value in each bin.
Definition: TabularModel.h:58
sv_frame_t getFrameForRow(int row) const override
Return the frame time for the given row.
Definition: DenseThreeDimensionalModel.h:176
SortType getSortType(int) const override
Return the sort type (numeric or alphabetical) for the column.
Definition: DenseThreeDimensionalModel.h:172
int getRowForFrame(sv_frame_t frame) const override
Return the number of the first row whose frame time is not less than the given one.
Definition: DenseThreeDimensionalModel.h:179
DenseThreeDimensionalModel()
Definition: DenseThreeDimensionalModel.h:184
bool isOverThreshold(int x, int y, float threshold)
Utility function to query whether a given bin is greater than a certain threshold.
Definition: DenseThreeDimensionalModel.h:117
Command * getRemoveRowCommand(int) override
Return a command to delete the row with the given index.
Definition: DenseThreeDimensionalModel.h:138
virtual int getResolution() const =0
Return the number of sample frames covered by each column of bins.
QString getHeading(int column) const override
Return the heading for a given column, e.g.
Definition: DenseThreeDimensionalModel.h:140
virtual float getBinValue(int n) const
Return the value of bin n, if any.
Definition: DenseThreeDimensionalModel.h:88
virtual bool hasBinValues() const
Return true if the bins have values as well as names.
Definition: DenseThreeDimensionalModel.h:81
virtual sv_samplerate_t getSampleRate() const =0
Return the frame rate in frames per second.
Model is the base class for all data models that represent any sort of data on a time scale based on ...
Definition: Model.h:51
TabularModel is an abstract base class for models that support direct access to data in a tabular for...
Definition: TabularModel.h:35
virtual Column getColumn(int column) const =0
Get data from the given column of bin values.
QVariant getData(int row, int column, int) const override
Get the value in the given cell, for the given role.
Definition: DenseThreeDimensionalModel.h:154
virtual QString getBinName(int n) const =0
Get the name of a given bin (i.e.
virtual sv_frame_t getStartFrame() const =0
Return the first audio frame spanned by the model.
Command * getInsertRowCommand(int) override
Return a command to insert a new row before the row with the given index.
Definition: DenseThreeDimensionalModel.h:137
Definition: Command.h:26
virtual int getCompletion() const override=0
Return an estimated percentage value showing how far through any background operation used to calcula...
virtual float getMaximumLevel() const =0
Return the maximum permissible value in each bin.
std::string toText(bool fixedDp=false) const
Return a user-readable string to the nearest millisecond, typically in a form like HH:MM:SS...
Definition: RealTimeSV.cpp:274
bool isColumnTimeValue(int col) const override
Return true if the column is the frame time of the item, or an alternative representation of it (i...
Definition: DenseThreeDimensionalModel.h:169
virtual int getHeight() const =0
Return the number of bins in each column.
ColumnOp::Column Column
Definition: DenseThreeDimensionalModel.h:59
RealTime represents time values to nanosecond precision with accurate arithmetic and frame-rate conve...
Definition: RealTime.h:42
Generated by
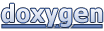