svcore
1.9
|
TabularModel.h
Go to the documentation of this file.
virtual bool isEditable() const =0
Return true if the model is user-editable, false otherwise.
Definition: TabularModel.h:57
static RealTime frame2RealTime(sv_frame_t frame, sv_samplerate_t sampleRate)
Convert a sample frame at the given sample rate into a RealTime.
Definition: RealTimeSV.cpp:498
virtual int getRowCount() const =0
Return the number of rows (items) in the model.
virtual sv_frame_t getFrameForRow(int row) const =0
Return the frame time for the given row.
static QVariant adaptFrameForRole(sv_frame_t frame, sv_samplerate_t rate, int role)
Definition: TabularModel.h:124
virtual Command * getInsertRowCommand(int beforeRow)=0
Return a command to insert a new row before the row with the given index.
std::string toString(bool align=false) const
Return a human-readable debug-type string to full precision (probably not a format to show to a user ...
Definition: RealTimeSV.cpp:213
Definition: TabularModel.h:58
Definition: TabularModel.h:58
virtual Command * getRemoveRowCommand(int row)=0
Return a command to delete the row with the given index.
static QVariant adaptValueForRole(float value, QString unit, int role)
Definition: TabularModel.h:133
virtual SortType getSortType(int col) const =0
Return the sort type (numeric or alphabetical) for the column.
virtual int getRowForFrame(sv_frame_t frame) const =0
Return the number of the first row whose frame time is not less than the given one.
virtual Command * getSetDataCommand(int row, int column, const QVariant &, int role)=0
Return a command to set the value in the given cell, for the given role, to the contents of the suppl...
TabularModel is an abstract base class for models that support direct access to data in a tabular for...
Definition: TabularModel.h:35
virtual QVariant getData(int row, int column, int role) const =0
Get the value in the given cell, for the given role.
virtual QString getHeading(int column) const =0
Return the heading for a given column, e.g.
Definition: Command.h:26
virtual int getColumnCount() const =0
Return the number of columns (values/labels/etc per item).
std::string toText(bool fixedDp=false) const
Return a user-readable string to the nearest millisecond, typically in a form like HH:MM:SS...
Definition: RealTimeSV.cpp:274
virtual bool isColumnTimeValue(int col) const =0
Return true if the column is the frame time of the item, or an alternative representation of it (i...
RealTime represents time values to nanosecond precision with accurate arithmetic and frame-rate conve...
Definition: RealTime.h:42
Generated by
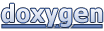