svcore
1.9
|
Command.h
Go to the documentation of this file.
virtual void unexecute()=0
BundleCommand is a MacroCommand whose name includes a note of how many commands it contains...
Definition: Command.h:112
virtual QString getName() const =0
GenericCommand(QString name, std::function< void()> execute, std::function< void()> unexecute, std::function< void()> onDelete)
Definition: Command.h:53
GenericCommand is a Command that can be constructed directly using lambdas, without having to create ...
Definition: Command.h:41
Definition: Command.h:26
Definition: Command.h:86
virtual void execute()=0
GenericCommand(QString name, std::function< void()> execute, std::function< void()> unexecute)
Definition: Command.h:44
Generated by
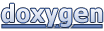