svcore
1.9
|
TabularModel is an abstract base class for models that support direct access to data in a tabular form. More...
#include <TabularModel.h>
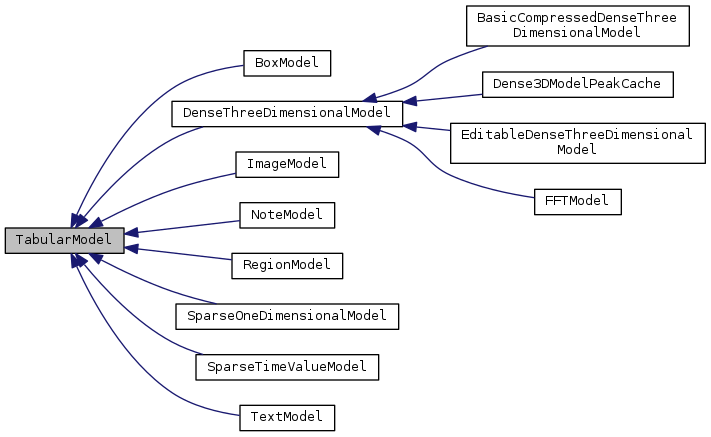
Public Types | |
enum | { SortRole = Qt::UserRole } |
enum | SortType { SortNumeric, SortAlphabetical } |
Public Member Functions | |
virtual | ~TabularModel () |
virtual int | getRowCount () const =0 |
Return the number of rows (items) in the model. More... | |
virtual int | getColumnCount () const =0 |
Return the number of columns (values/labels/etc per item). More... | |
virtual QString | getHeading (int column) const =0 |
Return the heading for a given column, e.g. More... | |
virtual QVariant | getData (int row, int column, int role) const =0 |
Get the value in the given cell, for the given role. More... | |
virtual bool | isColumnTimeValue (int col) const =0 |
Return true if the column is the frame time of the item, or an alternative representation of it (i.e. More... | |
virtual SortType | getSortType (int col) const =0 |
Return the sort type (numeric or alphabetical) for the column. More... | |
virtual sv_frame_t | getFrameForRow (int row) const =0 |
Return the frame time for the given row. More... | |
virtual int | getRowForFrame (sv_frame_t frame) const =0 |
Return the number of the first row whose frame time is not less than the given one. More... | |
virtual bool | isEditable () const =0 |
Return true if the model is user-editable, false otherwise. More... | |
virtual Command * | getSetDataCommand (int row, int column, const QVariant &, int role)=0 |
Return a command to set the value in the given cell, for the given role, to the contents of the supplied variant. More... | |
virtual Command * | getInsertRowCommand (int beforeRow)=0 |
Return a command to insert a new row before the row with the given index. More... | |
virtual Command * | getRemoveRowCommand (int row)=0 |
Return a command to delete the row with the given index. More... | |
Static Protected Member Functions | |
static QVariant | adaptFrameForRole (sv_frame_t frame, sv_samplerate_t rate, int role) |
static QVariant | adaptValueForRole (float value, QString unit, int role) |
Detailed Description
TabularModel is an abstract base class for models that support direct access to data in a tabular form.
A model that implements TabularModel may be displayed and, perhaps, edited in a data spreadsheet window.
This is very like a cut-down QAbstractItemModel. It assumes a relationship between row number and frame time.
Definition at line 35 of file TabularModel.h.
Member Enumeration Documentation
anonymous enum |
Enumerator | |
---|---|
SortRole |
Definition at line 57 of file TabularModel.h.
Enumerator | |
---|---|
SortNumeric | |
SortAlphabetical |
Definition at line 58 of file TabularModel.h.
Constructor & Destructor Documentation
|
inlinevirtual |
Definition at line 38 of file TabularModel.h.
References getColumnCount(), getHeading(), and getRowCount().
Member Function Documentation
|
pure virtual |
Return the number of rows (items) in the model.
Implemented in NoteModel, SparseTimeValueModel, BoxModel, RegionModel, SparseOneDimensionalModel, TextModel, ImageModel, and DenseThreeDimensionalModel.
Referenced by ~TabularModel().
|
pure virtual |
Return the number of columns (values/labels/etc per item).
Implemented in NoteModel, SparseTimeValueModel, BoxModel, RegionModel, SparseOneDimensionalModel, TextModel, ImageModel, and DenseThreeDimensionalModel.
Referenced by ~TabularModel().
|
pure virtual |
Return the heading for a given column, e.g.
"Time" or "Value". These are shown directly to the user, so must be translated already.
Implemented in NoteModel, SparseTimeValueModel, BoxModel, RegionModel, SparseOneDimensionalModel, TextModel, ImageModel, and DenseThreeDimensionalModel.
Referenced by ~TabularModel().
|
pure virtual |
Get the value in the given cell, for the given role.
The role is actually a Qt::ItemDataRole.
Implemented in NoteModel, SparseTimeValueModel, BoxModel, RegionModel, SparseOneDimensionalModel, TextModel, ImageModel, and DenseThreeDimensionalModel.
|
pure virtual |
Return true if the column is the frame time of the item, or an alternative representation of it (i.e.
anything that has the same sort order). Duration is not a time value by this meaning.
Implemented in NoteModel, SparseTimeValueModel, BoxModel, RegionModel, SparseOneDimensionalModel, DenseThreeDimensionalModel, TextModel, and ImageModel.
|
pure virtual |
Return the sort type (numeric or alphabetical) for the column.
Implemented in NoteModel, SparseTimeValueModel, BoxModel, RegionModel, SparseOneDimensionalModel, TextModel, ImageModel, and DenseThreeDimensionalModel.
|
pure virtual |
Return the frame time for the given row.
Implemented in NoteModel, SparseTimeValueModel, BoxModel, RegionModel, SparseOneDimensionalModel, DenseThreeDimensionalModel, TextModel, and ImageModel.
|
pure virtual |
Return the number of the first row whose frame time is not less than the given one.
If there is none, return getRowCount().
Implemented in NoteModel, SparseTimeValueModel, BoxModel, RegionModel, SparseOneDimensionalModel, DenseThreeDimensionalModel, TextModel, and ImageModel.
|
pure virtual |
Return true if the model is user-editable, false otherwise.
Implemented in NoteModel, SparseTimeValueModel, BoxModel, RegionModel, SparseOneDimensionalModel, TextModel, ImageModel, and DenseThreeDimensionalModel.
|
pure virtual |
Return a command to set the value in the given cell, for the given role, to the contents of the supplied variant.
If the model is not editable or the cell or value is out of range, return nullptr.
Implemented in NoteModel, SparseTimeValueModel, BoxModel, RegionModel, SparseOneDimensionalModel, TextModel, ImageModel, and DenseThreeDimensionalModel.
|
pure virtual |
Return a command to insert a new row before the row with the given index.
If the model is not editable or the index is out of range, return nullptr.
Implemented in NoteModel, SparseTimeValueModel, BoxModel, RegionModel, SparseOneDimensionalModel, TextModel, ImageModel, and DenseThreeDimensionalModel.
|
pure virtual |
Return a command to delete the row with the given index.
If the model is not editable or the index is out of range, return nullptr.
Implemented in NoteModel, SparseTimeValueModel, BoxModel, RegionModel, SparseOneDimensionalModel, TextModel, ImageModel, and DenseThreeDimensionalModel.
|
inlinestaticprotected |
Definition at line 124 of file TabularModel.h.
References RealTime::frame2RealTime(), SortRole, RealTime::toString(), and RealTime::toText().
Referenced by ImageModel::getData(), TextModel::getData(), SparseOneDimensionalModel::getData(), RegionModel::getData(), BoxModel::getData(), SparseTimeValueModel::getData(), and NoteModel::getData().
|
inlinestaticprotected |
Definition at line 133 of file TabularModel.h.
References SortRole.
Referenced by RegionModel::getData(), BoxModel::getData(), SparseTimeValueModel::getData(), and NoteModel::getData().
The documentation for this class was generated from the following file:
Generated by
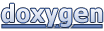