svcore
1.9
|
NoteModel.h
Go to the documentation of this file.
EventVector getEventsCovering(sv_frame_t f) const
Definition: NoteModel.h:181
QVector< QString > getStringExportHeaders(DataExportOptions options) const override
Return a label for each column that would be written by toStringExportRows.
Definition: NoteModel.h:412
void toXml(QTextStream &stream, QString indent="", QString extraAttributes="") const override
Stream this exportable object out to XML on a text stream.
Definition: Model.cpp:204
Definition: NoteModel.h:43
Command * getSetDataCommand(int row, int column, const QVariant &value, int role) override
Return a command to set the value in the given cell, for the given role, to the contents of the suppl...
Definition: NoteModel.h:298
static PlayParameterRepository * getInstance()
Definition: PlayParameterRepository.cpp:26
Definition: NoteExportable.h:20
sv_samplerate_t getSampleRate() const override
Return the frame rate in frames per second.
Definition: NoteModel.h:116
EventVector getEventsWithin(sv_frame_t f, sv_frame_t duration) const
Definition: NoteModel.h:184
EventVector getEventsStartingAt(sv_frame_t frame) const
Retrieve all events starting at exactly the given frame.
Definition: EventSeries.h:143
void toXml(QTextStream &out, QString indent="", QString extraAttributes="") const override
XmlExportable methods.
Definition: NoteModel.h:384
static QVariant adaptFrameForRole(sv_frame_t frame, sv_samplerate_t rate, int role)
Definition: TabularModel.h:124
NoteList getNotesStartingWithin(sv_frame_t startFrame, sv_frame_t duration) const override
Get notes that start within the range in frames defined by the given start frame and duration...
Definition: NoteModel.h:368
Event getEventByIndex(int index) const
Return the event at the given numerical index in the series, where 0 = the first event and count()-1 ...
Definition: EventSeries.cpp:547
void update(sv_frame_t frame, sv_frame_t duration)
Definition: DeferredNotifier.h:43
EventVector getAllEvents() const
Retrieve all events, in their natural order.
Definition: EventSeries.cpp:464
bool getNearestEventMatching(sv_frame_t startSearchAt, std::function< bool(Event)> predicate, EventSeries::Direction direction, Event &found) const
Definition: NoteModel.h:193
QVariant getData(int row, int column, int role) const override
Get the value in the given cell, for the given role.
Definition: NoteModel.h:279
bool isColumnTimeValue(int column) const override
Return true if the column is the frame time of the item, or an alternative representation of it (i...
Definition: NoteModel.h:249
sv_frame_t getTrueEndFrame() const override
Return the audio frame at the end of the model.
Definition: NoteModel.h:108
sv_frame_t getStartFrame() const
Return the frame of the first event in the series.
Definition: EventSeries.cpp:265
int getColumnCount() const override
Return the number of columns (values/labels/etc per item).
Definition: NoteModel.h:245
QVector< QVector< QString > > toStringExportRows(DataExportOptions options, sv_frame_t startFrame, sv_frame_t duration, sv_samplerate_t sampleRate, sv_frame_t resolution, Event fillEvent) const
Emit events starting within the given range as string rows ready for conversion to an e...
Definition: EventSeries.cpp:615
Command * getRemoveRowCommand(int row) override
Return a command to delete the row with the given index.
Definition: NoteModel.h:339
Definition: DeferredNotifier.h:25
Definition: TabularModel.h:58
void makeDeferredNotifications()
Definition: DeferredNotifier.h:53
Definition: NoteModel.h:33
bool isEditable() const override
Return true if the model is user-editable, false otherwise.
Definition: NoteModel.h:328
QVector< QString > getStringExportHeaders(DataExportOptions options, Event::ExportNameOptions) const
Return a label for each column that would be written by toStringExportRows.
Definition: EventSeries.cpp:604
Definition: TabularModel.h:58
int getIndexForEvent(const Event &e) const
Return the index of the first event in the series that does not compare inferior to the given event...
Definition: EventSeries.cpp:557
sv_frame_t getEndFrame() const
Return the audio frame at the end of the model, i.e.
Definition: Model.h:87
sv_frame_t getFrameForRow(int row) const override
Return the frame time for the given row.
Definition: NoteModel.h:255
void toXml(QTextStream &out, QString indent, QString extraAttributes) const override
Emit to XML as a dataset element.
Definition: EventSeries.cpp:567
sv_frame_t getStartFrame() const override
Return the first audio frame spanned by the model.
Definition: NoteModel.h:104
EventVector getEventsCovering(sv_frame_t frame) const
Retrieve all events that cover the given frame.
Definition: EventSeries.cpp:420
static QVariant adaptValueForRole(float value, QString unit, int role)
Definition: TabularModel.h:133
EventVector getEventsSpanning(sv_frame_t frame, sv_frame_t duration) const
Retrieve all events any part of which falls within the range in frames defined by the given frame f a...
Definition: EventSeries.cpp:294
Model is the base class for all data models that represent any sort of data on a time scale based on ...
Definition: Model.h:51
TabularModel is an abstract base class for models that support direct access to data in a tabular for...
Definition: TabularModel.h:35
void completionChanged(ModelId myId)
Emitted when some internal processing has advanced a stage, but the model has not changed externally...
int getRowForFrame(sv_frame_t frame) const override
Return the number of the first row whose frame time is not less than the given one.
Definition: NoteModel.h:263
QVector< QVector< QString > > toStringExportRows(DataExportOptions options, sv_frame_t startFrame, sv_frame_t duration) const override
Emit events starting within the given range as string rows ready for conversion to an e...
Definition: NoteModel.h:417
sv_frame_t getEndFrame() const
Return the frame plus duration of the event in the series that ends last.
Definition: EventSeries.cpp:273
EventVector getEventsSpanning(sv_frame_t f, sv_frame_t duration) const
Definition: NoteModel.h:178
QString getHeading(int column) const override
Return the heading for a given column, e.g.
Definition: NoteModel.h:267
EventVector getEventsStartingWithin(sv_frame_t f, sv_frame_t duration) const
Definition: NoteModel.h:187
SortType getSortType(int column) const override
Return the sort type (numeric or alphabetical) for the column.
Definition: NoteModel.h:322
ExportId getExportId() const
Return the numerical export identifier for this object.
Definition: XmlExportable.cpp:71
Command * getInsertRowCommand(int row) override
Return a command to insert a new row before the row with the given index.
Definition: NoteModel.h:330
void setCompletion(int completion, bool update=true)
Definition: NoteModel.h:142
bool getNearestEventMatching(sv_frame_t startSearchAt, std::function< bool(const Event &)> predicate, Direction direction, Event &found) const
Return the first event for which the given predicate returns true, searching events with start frames...
Definition: EventSeries.cpp:508
bool isOK() const override
Return true if the model was constructed successfully.
Definition: NoteModel.h:102
NoteModel(sv_samplerate_t sampleRate, int resolution, float valueMinimum, float valueMaximum, bool notifyOnAdd=true, Subtype subtype=NORMAL_NOTE)
Definition: NoteModel.h:72
void modelChangedWithin(ModelId myId, sv_frame_t startFrame, sv_frame_t endFrame)
Emitted when a model has been edited (or more data retrieved from cache, in the case of a cached mode...
int getCompletion() const override
Return an estimated percentage value showing how far through any background operation used to calcula...
Definition: NoteModel.h:140
EventVector getEventsWithin(sv_frame_t frame, sv_frame_t duration, int overspill=0) const
Retrieve all events falling wholly within the range in frames defined by the given frame f and durati...
Definition: EventSeries.cpp:342
NoteModel(sv_samplerate_t sampleRate, int resolution, bool notifyOnAdd=true, Subtype subtype=NORMAL_NOTE)
Definition: NoteModel.h:46
An immutable(-ish) type used for point and event representation in sparse models, as well as for inte...
Definition: Event.h:55
void addPlayable(int id, const Playable *)
Register a playable.
Definition: PlayParameterRepository.cpp:36
Definition: Command.h:26
Definition: NoteModel.h:42
EventVector getEventsStartingAt(sv_frame_t f) const
Definition: NoteModel.h:190
Container storing a series of events, with or without durations, and supporting the ability to query ...
Definition: EventSeries.h:49
NoteList getNotesActiveAt(sv_frame_t frame) const override
Get notes that are active at the given frame, i.e.
Definition: NoteModel.h:357
Interface for classes that can be modified through these commands.
Definition: EventCommands.h:26
EventVector getEventsStartingWithin(sv_frame_t frame, sv_frame_t duration) const
Retrieve all events starting within the range in frames defined by the given frame f and duration d...
Definition: EventSeries.cpp:395
QString getDefaultPlayClipId() const override
Definition: NoteModel.h:120
void modelChanged(ModelId myId)
Emitted when a model has been edited (or more data retrieved from cache, in the case of a cached mode...
Command to add or remove a series of events to or from an editable, with undo.
Definition: EventCommands.h:115
Generated by
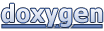