svcore
1.9
|
DeferredNotifier.h
Go to the documentation of this file.
void update(sv_frame_t frame, sv_frame_t duration)
Definition: DeferredNotifier.h:43
Definition: DeferredNotifier.h:25
void makeDeferredNotifications()
Definition: DeferredNotifier.h:53
DeferredNotifier(Model *m, ModelId id, Mode mode)
Definition: DeferredNotifier.h:33
Model is the base class for all data models that represent any sort of data on a time scale based on ...
Definition: Model.h:51
void modelChangedWithin(ModelId myId, sv_frame_t startFrame, sv_frame_t endFrame)
Emitted when a model has been edited (or more data retrieved from cache, in the case of a cached mode...
Definition: ById.h:115
Generated by
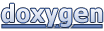