svcore
1.9
|
Container storing a series of events, with or without durations, and supporting the ability to query which events are active at a given frame or within a span of frames. More...
#include <EventSeries.h>
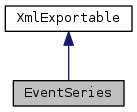
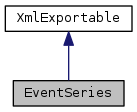
Public Types | |
enum | Direction { Forward, Backward } |
enum | { NO_ID = -1 } |
typedef int | ExportId |
Public Member Functions | |
EventSeries () | |
~EventSeries ()=default | |
EventSeries (const EventSeries &) | |
EventSeries & | operator= (const EventSeries &) |
EventSeries & | operator= (EventSeries &&) |
bool | operator== (const EventSeries &other) const |
void | clear () |
void | add (const Event &e) |
void | remove (const Event &e) |
bool | contains (const Event &e) const |
bool | isEmpty () const |
int | count () const |
sv_frame_t | getStartFrame () const |
Return the frame of the first event in the series. More... | |
sv_frame_t | getEndFrame () const |
Return the frame plus duration of the event in the series that ends last. More... | |
EventVector | getEventsSpanning (sv_frame_t frame, sv_frame_t duration) const |
Retrieve all events any part of which falls within the range in frames defined by the given frame f and duration d. More... | |
EventVector | getEventsCovering (sv_frame_t frame) const |
Retrieve all events that cover the given frame. More... | |
EventVector | getEventsWithin (sv_frame_t frame, sv_frame_t duration, int overspill=0) const |
Retrieve all events falling wholly within the range in frames defined by the given frame f and duration d. More... | |
EventVector | getEventsStartingWithin (sv_frame_t frame, sv_frame_t duration) const |
Retrieve all events starting within the range in frames defined by the given frame f and duration d. More... | |
EventVector | getEventsStartingAt (sv_frame_t frame) const |
Retrieve all events starting at exactly the given frame. More... | |
EventVector | getAllEvents () const |
Retrieve all events, in their natural order. More... | |
bool | getEventPreceding (const Event &e, Event &preceding) const |
If e is in the series and is not the first event in it, set preceding to the event immediate preceding it according to the standard event ordering and return true. More... | |
bool | getEventFollowing (const Event &e, Event &following) const |
If e is in the series and is not the final event in it, set following to the event immediate following it according to the standard event ordering and return true. More... | |
bool | getNearestEventMatching (sv_frame_t startSearchAt, std::function< bool(const Event &)> predicate, Direction direction, Event &found) const |
Return the first event for which the given predicate returns true, searching events with start frames increasingly far from the given frame in the given direction. More... | |
Event | getEventByIndex (int index) const |
Return the event at the given numerical index in the series, where 0 = the first event and count()-1 = the last. More... | |
int | getIndexForEvent (const Event &e) const |
Return the index of the first event in the series that does not compare inferior to the given event. More... | |
void | toXml (QTextStream &out, QString indent, QString extraAttributes) const override |
Emit to XML as a dataset element. More... | |
void | toXml (QTextStream &out, QString indent, QString extraAttributes, Event::ExportNameOptions) const |
Emit to XML as a dataset element. More... | |
QVector< QString > | getStringExportHeaders (DataExportOptions options, Event::ExportNameOptions) const |
Return a label for each column that would be written by toStringExportRows. More... | |
QVector< QVector< QString > > | toStringExportRows (DataExportOptions options, sv_frame_t startFrame, sv_frame_t duration, sv_samplerate_t sampleRate, sv_frame_t resolution, Event fillEvent) const |
Emit events starting within the given range as string rows ready for conversion to an e.g. More... | |
ExportId | getExportId () const |
Return the numerical export identifier for this object. More... | |
virtual QString | toXmlString (QString indent="", QString extraAttributes="") const |
Convert this exportable object to XML in a string. More... | |
Static Public Member Functions | |
static EventSeries | fromEvents (const EventVector &ee) |
static QString | encodeEntities (QString) |
static QString | encodeColour (int r, int g, int b) |
Private Types | |
typedef std::vector< Event > | Events |
This vector contains all events in the series, in the normal sort order. More... | |
typedef std::map< sv_frame_t, std::vector< Event > > | FrameEventMap |
The FrameEventMap maps from frame number to a set of events. More... | |
Private Member Functions | |
EventSeries (const EventSeries &other, const QMutexLocker &) | |
void | createSeam (sv_frame_t frame) |
Create a seam at the given frame, copying from the prior seam if there is one. More... | |
bool | seamsEqual (const std::vector< Event > &s1, const std::vector< Event > &s2) const |
Return true if the two seam map entries contain the same set of events. More... | |
Private Attributes | |
QMutex | m_mutex |
Events | m_events |
FrameEventMap | m_seams |
sv_frame_t | m_finalDurationlessEventFrame |
The frame of the last durationless event we have in the series. More... | |
Detailed Description
Container storing a series of events, with or without durations, and supporting the ability to query which events are active at a given frame or within a span of frames.
To that end, in addition to the series of events, it stores a series of "seams", which are frame positions at which the set of simultaneous events changes (i.e. an event of non-zero duration starts or ends) associated with a set of the events that are active at or from that position. These are updated when an event is added or removed.
This class is highly optimised for inserting events in increasing order of start frame. Inserting (or deleting) events in the middle does work, and should be acceptable in interactive use, but it is very slow in bulk.
EventSeries is thread-safe.
Definition at line 49 of file EventSeries.h.
Member Typedef Documentation
|
private |
This vector contains all events in the series, in the normal sort order.
For backward compatibility we must support series containing multiple instances of identical events, so consecutive events in this vector will not always be distinct. The vector is used in preference to a multiset or map<Event, int> in order to allow indexing by "row number" as well as by properties such as frame.
Because events are immutable, we do not have to worry about the order changing once an event is inserted - we only add or delete them.
Definition at line 263 of file EventSeries.h.
|
private |
The FrameEventMap maps from frame number to a set of events.
In the seam map this is used to represent the events that are active at that frame, either because they begin at that frame or because they are continuing from an earlier frame. There is an entry here for each frame at which an event starts or ends, with the event appearing in all entries from its start time onward and disappearing again at its end frame.
Only events with duration appear in this map; point events appear only in m_events. Note that unlike m_events, we only store one instance of each event here, even if we hold many - we refer back to m_events when we need to know how many identical copies of a given event we have.
Definition at line 281 of file EventSeries.h.
|
inherited |
Definition at line 33 of file XmlExportable.h.
Member Enumeration Documentation
Enumerator | |
---|---|
Forward | |
Backward |
Definition at line 180 of file EventSeries.h.
|
inherited |
Enumerator | |
---|---|
NO_ID |
Definition at line 28 of file XmlExportable.h.
Constructor & Destructor Documentation
|
inline |
Definition at line 52 of file EventSeries.h.
References add(), clear(), contains(), count(), fromEvents(), getEndFrame(), getEventsCovering(), getEventsSpanning(), getEventsStartingWithin(), getEventsWithin(), getStartFrame(), isEmpty(), operator=(), operator==(), and ~EventSeries().
|
default |
Referenced by EventSeries().
EventSeries::EventSeries | ( | const EventSeries & | other | ) |
Definition at line 22 of file EventSeries.cpp.
|
private |
Definition at line 27 of file EventSeries.cpp.
Member Function Documentation
EventSeries & EventSeries::operator= | ( | const EventSeries & | other | ) |
Definition at line 35 of file EventSeries.cpp.
References m_events, m_finalDurationlessEventFrame, m_mutex, and m_seams.
Referenced by EventSeries().
EventSeries & EventSeries::operator= | ( | EventSeries && | other | ) |
Definition at line 45 of file EventSeries.cpp.
References m_events, m_finalDurationlessEventFrame, m_mutex, and m_seams.
bool EventSeries::operator== | ( | const EventSeries & | other | ) | const |
Definition at line 55 of file EventSeries.cpp.
References m_events, and m_mutex.
Referenced by EventSeries().
|
static |
void EventSeries::clear | ( | ) |
Definition at line 256 of file EventSeries.cpp.
References m_events, m_finalDurationlessEventFrame, m_mutex, and m_seams.
Referenced by EventSeries().
void EventSeries::add | ( | const Event & | e | ) |
Definition at line 89 of file EventSeries.cpp.
References createSeam(), Event::getDuration(), Event::getFrame(), Event::hasDuration(), m_events, m_finalDurationlessEventFrame, m_mutex, m_seams, and SVCERR.
Referenced by TextModel::add(), ImageModel::add(), SparseOneDimensionalModel::add(), BoxModel::add(), RegionModel::add(), SparseTimeValueModel::add(), NoteModel::add(), EventSeries(), and fromEvents().
void EventSeries::remove | ( | const Event & | e | ) |
Definition at line 136 of file EventSeries.cpp.
References Event::getDuration(), Event::getFrame(), Event::hasDuration(), m_events, m_finalDurationlessEventFrame, m_mutex, m_seams, seamsEqual(), SVCERR, and Event::toXmlString().
Referenced by ImageModel::remove(), TextModel::remove(), SparseOneDimensionalModel::remove(), BoxModel::remove(), RegionModel::remove(), SparseTimeValueModel::remove(), and NoteModel::remove().
bool EventSeries::contains | ( | const Event & | e | ) | const |
Definition at line 249 of file EventSeries.cpp.
References m_events, and m_mutex.
Referenced by TextModel::containsEvent(), ImageModel::containsEvent(), SparseOneDimensionalModel::containsEvent(), BoxModel::containsEvent(), RegionModel::containsEvent(), SparseTimeValueModel::containsEvent(), NoteModel::containsEvent(), and EventSeries().
bool EventSeries::isEmpty | ( | ) | const |
Definition at line 72 of file EventSeries.cpp.
References m_events, and m_mutex.
Referenced by EventSeries(), TextModel::getTrueEndFrame(), ImageModel::getTrueEndFrame(), SparseOneDimensionalModel::getTrueEndFrame(), RegionModel::getTrueEndFrame(), BoxModel::getTrueEndFrame(), SparseTimeValueModel::getTrueEndFrame(), NoteModel::getTrueEndFrame(), TextModel::isEmpty(), ImageModel::isEmpty(), SparseOneDimensionalModel::isEmpty(), BoxModel::isEmpty(), RegionModel::isEmpty(), SparseTimeValueModel::isEmpty(), and NoteModel::isEmpty().
int EventSeries::count | ( | ) | const |
Definition at line 79 of file EventSeries.cpp.
References m_events, and m_mutex.
Referenced by EventSeries(), ImageModel::getData(), TextModel::getData(), SparseOneDimensionalModel::getData(), RegionModel::getData(), BoxModel::getData(), SparseTimeValueModel::getData(), NoteModel::getData(), TextModel::getEventCount(), ImageModel::getEventCount(), SparseOneDimensionalModel::getEventCount(), BoxModel::getEventCount(), RegionModel::getEventCount(), SparseTimeValueModel::getEventCount(), NoteModel::getEventCount(), ImageModel::getFrameForRow(), TextModel::getFrameForRow(), SparseOneDimensionalModel::getFrameForRow(), RegionModel::getFrameForRow(), BoxModel::getFrameForRow(), SparseTimeValueModel::getFrameForRow(), NoteModel::getFrameForRow(), ImageModel::getInsertRowCommand(), TextModel::getInsertRowCommand(), SparseOneDimensionalModel::getInsertRowCommand(), RegionModel::getInsertRowCommand(), BoxModel::getInsertRowCommand(), SparseTimeValueModel::getInsertRowCommand(), NoteModel::getInsertRowCommand(), ImageModel::getRemoveRowCommand(), TextModel::getRemoveRowCommand(), SparseOneDimensionalModel::getRemoveRowCommand(), RegionModel::getRemoveRowCommand(), BoxModel::getRemoveRowCommand(), SparseTimeValueModel::getRemoveRowCommand(), NoteModel::getRemoveRowCommand(), ImageModel::getRowCount(), TextModel::getRowCount(), SparseOneDimensionalModel::getRowCount(), RegionModel::getRowCount(), BoxModel::getRowCount(), SparseTimeValueModel::getRowCount(), NoteModel::getRowCount(), ImageModel::getSetDataCommand(), TextModel::getSetDataCommand(), SparseOneDimensionalModel::getSetDataCommand(), RegionModel::getSetDataCommand(), BoxModel::getSetDataCommand(), SparseTimeValueModel::getSetDataCommand(), and NoteModel::getSetDataCommand().
sv_frame_t EventSeries::getStartFrame | ( | ) | const |
Return the frame of the first event in the series.
If there are no events, return 0.
Definition at line 265 of file EventSeries.cpp.
References m_events, and m_mutex.
Referenced by EventSeries(), TextModel::getStartFrame(), ImageModel::getStartFrame(), SparseOneDimensionalModel::getStartFrame(), RegionModel::getStartFrame(), BoxModel::getStartFrame(), SparseTimeValueModel::getStartFrame(), and NoteModel::getStartFrame().
sv_frame_t EventSeries::getEndFrame | ( | ) | const |
Return the frame plus duration of the event in the series that ends last.
If there are no events, return 0.
Definition at line 273 of file EventSeries.cpp.
References m_events, m_finalDurationlessEventFrame, m_mutex, and m_seams.
Referenced by EventSeries(), TextModel::getTrueEndFrame(), ImageModel::getTrueEndFrame(), SparseOneDimensionalModel::getTrueEndFrame(), RegionModel::getTrueEndFrame(), BoxModel::getTrueEndFrame(), SparseTimeValueModel::getTrueEndFrame(), and NoteModel::getTrueEndFrame().
EventVector EventSeries::getEventsSpanning | ( | sv_frame_t | frame, |
sv_frame_t | duration | ||
) | const |
Retrieve all events any part of which falls within the range in frames defined by the given frame f and duration d.
- An event without duration is spanned by the range if its own frame is greater than or equal to f and less than f + d.
- An event with duration is spanned by the range if its start frame is less than f + d and its start frame plus its duration is greater than f.
Note: Passing a duration of zero is seldom useful here; you probably want getEventsCovering instead. getEventsSpanning(f, 0) is not equivalent to getEventsCovering(f). The latter includes durationless events at f and events starting at f, both of which are excluded from the former.
Definition at line 294 of file EventSeries.cpp.
References m_events, m_mutex, and m_seams.
Referenced by EventSeries(), TextModel::getEventsSpanning(), ImageModel::getEventsSpanning(), SparseOneDimensionalModel::getEventsSpanning(), BoxModel::getEventsSpanning(), RegionModel::getEventsSpanning(), SparseTimeValueModel::getEventsSpanning(), NoteModel::getEventsSpanning(), and BoxModel::toStringExportRows().
EventVector EventSeries::getEventsCovering | ( | sv_frame_t | frame | ) | const |
Retrieve all events that cover the given frame.
An event without duration covers a frame if its own frame is equal to it. An event with duration covers a frame if its start frame is less than or equal to it and its end frame (start + duration) is greater than it.
Definition at line 420 of file EventSeries.cpp.
References m_events, m_mutex, and m_seams.
Referenced by EventSeries(), TextModel::getEventsCovering(), ImageModel::getEventsCovering(), SparseOneDimensionalModel::getEventsCovering(), BoxModel::getEventsCovering(), RegionModel::getEventsCovering(), SparseTimeValueModel::getEventsCovering(), NoteModel::getEventsCovering(), and NoteModel::getNotesActiveAt().
EventVector EventSeries::getEventsWithin | ( | sv_frame_t | frame, |
sv_frame_t | duration, | ||
int | overspill = 0 |
||
) | const |
Retrieve all events falling wholly within the range in frames defined by the given frame f and duration d.
- An event without duration is within the range if its own frame is greater than or equal to f and less than f + d.
- An event with duration is within the range if its start frame is greater than or equal to f and its start frame plus its duration is less than or equal to f + d.
If overspill is greater than zero, also include that number of additional events (where they exist) both before and after the edges of the range.
Definition at line 342 of file EventSeries.cpp.
References m_events, and m_mutex.
Referenced by EventSeries(), TextModel::getEventsWithin(), ImageModel::getEventsWithin(), SparseOneDimensionalModel::getEventsWithin(), BoxModel::getEventsWithin(), RegionModel::getEventsWithin(), SparseTimeValueModel::getEventsWithin(), and NoteModel::getEventsWithin().
EventVector EventSeries::getEventsStartingWithin | ( | sv_frame_t | frame, |
sv_frame_t | duration | ||
) | const |
Retrieve all events starting within the range in frames defined by the given frame f and duration d.
An event (regardless of whether it has duration or not) starts within the range if its start frame is greater than or equal to f and less than f + d.
Definition at line 395 of file EventSeries.cpp.
References m_events, and m_mutex.
Referenced by EventSeries(), getEventsStartingAt(), TextModel::getEventsStartingWithin(), ImageModel::getEventsStartingWithin(), SparseOneDimensionalModel::getEventsStartingWithin(), BoxModel::getEventsStartingWithin(), RegionModel::getEventsStartingWithin(), SparseTimeValueModel::getEventsStartingWithin(), NoteModel::getEventsStartingWithin(), SparseOneDimensionalModel::getNotesStartingWithin(), and NoteModel::getNotesStartingWithin().
|
inline |
Retrieve all events starting at exactly the given frame.
Definition at line 143 of file EventSeries.h.
References getAllEvents(), getEventFollowing(), getEventPreceding(), and getEventsStartingWithin().
Referenced by TextModel::getEventsStartingAt(), ImageModel::getEventsStartingAt(), SparseOneDimensionalModel::getEventsStartingAt(), BoxModel::getEventsStartingAt(), RegionModel::getEventsStartingAt(), SparseTimeValueModel::getEventsStartingAt(), and NoteModel::getEventsStartingAt().
EventVector EventSeries::getAllEvents | ( | ) | const |
Retrieve all events, in their natural order.
Definition at line 464 of file EventSeries.cpp.
References m_events, and m_mutex.
Referenced by TextModel::getAllEvents(), ImageModel::getAllEvents(), SparseOneDimensionalModel::getAllEvents(), BoxModel::getAllEvents(), RegionModel::getAllEvents(), SparseTimeValueModel::getAllEvents(), NoteModel::getAllEvents(), and getEventsStartingAt().
If e is in the series and is not the first event in it, set preceding to the event immediate preceding it according to the standard event ordering and return true.
Otherwise leave preceding unchanged and return false.
If there are multiple events identical to e in the series, assume that the event passed in is the first one (i.e. never set preceding equal to e).
It is acceptable for preceding to alias e when this is called.
Definition at line 472 of file EventSeries.cpp.
References m_events, and m_mutex.
Referenced by getEventsStartingAt().
If e is in the series and is not the final event in it, set following to the event immediate following it according to the standard event ordering and return true.
Otherwise leave following unchanged and return false.
If there are multiple events identical to e in the series, assume that the event passed in is the last one (i.e. never set following equal to e).
It is acceptable for following to alias e when this is called.
Definition at line 489 of file EventSeries.cpp.
References m_events, and m_mutex.
Referenced by getEventsStartingAt().
bool EventSeries::getNearestEventMatching | ( | sv_frame_t | startSearchAt, |
std::function< bool(const Event &)> | predicate, | ||
Direction | direction, | ||
Event & | found | ||
) | const |
Return the first event for which the given predicate returns true, searching events with start frames increasingly far from the given frame in the given direction.
If the direction is Forward then the search includes events starting at the given frame, otherwise it does not.
Definition at line 508 of file EventSeries.cpp.
References Backward, Forward, m_events, and m_mutex.
Referenced by TextModel::getNearestEventMatching(), ImageModel::getNearestEventMatching(), SparseOneDimensionalModel::getNearestEventMatching(), BoxModel::getNearestEventMatching(), RegionModel::getNearestEventMatching(), SparseTimeValueModel::getNearestEventMatching(), and NoteModel::getNearestEventMatching().
Event EventSeries::getEventByIndex | ( | int | index | ) | const |
Return the event at the given numerical index in the series, where 0 = the first event and count()-1 = the last.
Definition at line 547 of file EventSeries.cpp.
References in_range_for(), m_events, and m_mutex.
Referenced by ImageModel::getData(), TextModel::getData(), SparseOneDimensionalModel::getData(), RegionModel::getData(), BoxModel::getData(), SparseTimeValueModel::getData(), NoteModel::getData(), ImageModel::getFrameForRow(), TextModel::getFrameForRow(), SparseOneDimensionalModel::getFrameForRow(), RegionModel::getFrameForRow(), BoxModel::getFrameForRow(), SparseTimeValueModel::getFrameForRow(), NoteModel::getFrameForRow(), ImageModel::getInsertRowCommand(), TextModel::getInsertRowCommand(), SparseOneDimensionalModel::getInsertRowCommand(), RegionModel::getInsertRowCommand(), BoxModel::getInsertRowCommand(), SparseTimeValueModel::getInsertRowCommand(), NoteModel::getInsertRowCommand(), ImageModel::getRemoveRowCommand(), TextModel::getRemoveRowCommand(), SparseOneDimensionalModel::getRemoveRowCommand(), RegionModel::getRemoveRowCommand(), BoxModel::getRemoveRowCommand(), SparseTimeValueModel::getRemoveRowCommand(), NoteModel::getRemoveRowCommand(), ImageModel::getSetDataCommand(), TextModel::getSetDataCommand(), SparseOneDimensionalModel::getSetDataCommand(), RegionModel::getSetDataCommand(), BoxModel::getSetDataCommand(), SparseTimeValueModel::getSetDataCommand(), and NoteModel::getSetDataCommand().
int EventSeries::getIndexForEvent | ( | const Event & | e | ) | const |
Return the index of the first event in the series that does not compare inferior to the given event.
If there is no such event, return count().
Definition at line 557 of file EventSeries.cpp.
References m_events, and m_mutex.
Referenced by NoteModel::getIndexForEvent(), ImageModel::getRowForFrame(), TextModel::getRowForFrame(), SparseOneDimensionalModel::getRowForFrame(), RegionModel::getRowForFrame(), BoxModel::getRowForFrame(), SparseTimeValueModel::getRowForFrame(), and NoteModel::getRowForFrame().
|
overridevirtual |
Emit to XML as a dataset element.
Implements XmlExportable.
Definition at line 567 of file EventSeries.cpp.
References XmlExportable::getExportId(), m_events, and m_mutex.
Referenced by ImageModel::toXml(), TextModel::toXml(), SparseOneDimensionalModel::toXml(), RegionModel::toXml(), BoxModel::toXml(), SparseTimeValueModel::toXml(), and NoteModel::toXml().
void EventSeries::toXml | ( | QTextStream & | out, |
QString | indent, | ||
QString | extraAttributes, | ||
Event::ExportNameOptions | options | ||
) | const |
Emit to XML as a dataset element.
Definition at line 585 of file EventSeries.cpp.
References XmlExportable::getExportId(), m_events, and m_mutex.
QVector< QString > EventSeries::getStringExportHeaders | ( | DataExportOptions | options, |
Event::ExportNameOptions | nopts | ||
) | const |
Return a label for each column that would be written by toStringExportRows.
Definition at line 604 of file EventSeries.cpp.
References m_events.
Referenced by ImageModel::getStringExportHeaders(), TextModel::getStringExportHeaders(), SparseOneDimensionalModel::getStringExportHeaders(), RegionModel::getStringExportHeaders(), SparseTimeValueModel::getStringExportHeaders(), and NoteModel::getStringExportHeaders().
QVector< QVector< QString > > EventSeries::toStringExportRows | ( | DataExportOptions | options, |
sv_frame_t | startFrame, | ||
sv_frame_t | duration, | ||
sv_samplerate_t | sampleRate, | ||
sv_frame_t | resolution, | ||
Event | fillEvent | ||
) | const |
Emit events starting within the given range as string rows ready for conversion to an e.g.
comma-separated data format.
Definition at line 615 of file EventSeries.cpp.
References DataExportFillGaps, m_events, m_mutex, Event::toStringExportRow(), and Event::withFrame().
Referenced by ImageModel::toStringExportRows(), TextModel::toStringExportRows(), SparseOneDimensionalModel::toStringExportRows(), RegionModel::toStringExportRows(), SparseTimeValueModel::toStringExportRows(), and NoteModel::toStringExportRows().
|
inlineprivate |
Create a seam at the given frame, copying from the prior seam if there is one.
If a seam already exists at the given frame, leave it untouched.
Call with m_mutex locked.
Definition at line 302 of file EventSeries.h.
Referenced by add().
|
inlineprivate |
Return true if the two seam map entries contain the same set of events.
Precondition: no duplicates, i.e. no event appears more than once in s1 or more than once in s2.
Call with m_mutex locked.
Definition at line 327 of file EventSeries.h.
References in_range_for().
Referenced by remove().
|
inherited |
Return the numerical export identifier for this object.
It's allocated the first time this is called, so objects on which this is never called do not get allocated one.
Definition at line 71 of file XmlExportable.cpp.
References XmlExportable::m_exportId, and mutex.
Referenced by EditableDenseThreeDimensionalModel::toXml(), BasicCompressedDenseThreeDimensionalModel::toXml(), toXml(), ImageModel::toXml(), TextModel::toXml(), Model::toXml(), SparseOneDimensionalModel::toXml(), RegionModel::toXml(), BoxModel::toXml(), SparseTimeValueModel::toXml(), NoteModel::toXml(), and XmlExportable::~XmlExportable().
|
virtualinherited |
Convert this exportable object to XML in a string.
The default implementation calls toXml and returns the result as a string. Do not override this unless you really know what you're doing.
Definition at line 25 of file XmlExportable.cpp.
References XmlExportable::toXml().
Referenced by ModelTransformerFactory::getConfigurationForTransform(), RDFTransformFactoryImpl::getTransforms(), and XmlExportable::~XmlExportable().
|
staticinherited |
Definition at line 41 of file XmlExportable.cpp.
Referenced by TextMatcher::test(), PluginXml::toXml(), ReadOnlyWaveFileModel::toXml(), Transform::toXml(), WritableWaveFileModel::toXml(), Model::toXml(), Event::toXml(), RegionModel::toXml(), BoxModel::toXml(), SparseTimeValueModel::toXml(), NoteModel::toXml(), and XmlExportable::~XmlExportable().
|
staticinherited |
Definition at line 54 of file XmlExportable.cpp.
Referenced by XmlExportable::~XmlExportable().
Member Data Documentation
|
mutableprivate |
Definition at line 246 of file EventSeries.h.
Referenced by add(), clear(), contains(), count(), getAllEvents(), getEndFrame(), getEventByIndex(), getEventFollowing(), getEventPreceding(), getEventsCovering(), getEventsSpanning(), getEventsStartingWithin(), getEventsWithin(), getIndexForEvent(), getNearestEventMatching(), getStartFrame(), isEmpty(), operator=(), operator==(), remove(), toStringExportRows(), and toXml().
|
private |
Definition at line 264 of file EventSeries.h.
Referenced by add(), clear(), contains(), count(), getAllEvents(), getEndFrame(), getEventByIndex(), getEventFollowing(), getEventPreceding(), getEventsCovering(), getEventsSpanning(), getEventsStartingWithin(), getEventsWithin(), getIndexForEvent(), getNearestEventMatching(), getStartFrame(), getStringExportHeaders(), isEmpty(), operator=(), operator==(), remove(), toStringExportRows(), and toXml().
|
private |
Definition at line 282 of file EventSeries.h.
Referenced by add(), clear(), getEndFrame(), getEventsCovering(), getEventsSpanning(), operator=(), and remove().
|
private |
The frame of the last durationless event we have in the series.
This is to support a fast-ish getEndFrame(): we can easily keep this up-to-date when events are added or removed, and we can easily find the end frame of the last with-duration event from the seam map, but it's not so easy to continuously update an overall end frame or to find the last frame of all events without this.
Definition at line 293 of file EventSeries.h.
Referenced by add(), clear(), getEndFrame(), operator=(), and remove().
The documentation for this class was generated from the following files:
Generated by
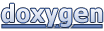