svcore
1.9
|
ModelTransformerFactory.cpp
Go to the documentation of this file.
113 SVDEBUG << "ModelTransformer: last configuration for identifier " << transform.getIdentifier() << ": " << configurationXml << endl;
119 SVDEBUG << "ModelTransformerFactory::getConfigurationForTransform: instantiating real-time plugin" << endl;
137 SVDEBUG << "ModelTransformerFactory::getConfigurationForTransform: instantiating Vamp plugin" << endl;
212 SVDEBUG << "ModelTransformerFactory::transform: Constructing transformer with input model " << input.getModel() << endl;
227 SVDEBUG << "ModelTransformerFactory::transformMultiple: Constructing transformer with input model " << input.getModel() << endl;
284 cerr << "WARNING: ModelTransformerFactory::transformerFinished: sender is not a transformer" << endl;
virtual void setParametersFromXml(QString xml)
Set the parameters and program of a plugin from an XML plugin element as returned by toXml...
Definition: PluginXml.cpp:195
ModelId transform(const Transform &transform, const ModelTransformer::Input &input, QString &message, AdditionalModelHandler *handler=0)
Return the output model resulting from applying the named transform to the given input model...
Definition: ModelTransformerFactory.cpp:207
ModelTransformer * createTransformer(const Transforms &transforms, const ModelTransformer::Input &input)
Definition: ModelTransformerFactory.cpp:184
virtual int getTargetChannelCount() const =0
Get the number of channels of audio that will be provided to the play target.
Definition: RealTimePluginFactory.h:50
static ModelTransformerFactory * getInstance()
Definition: ModelTransformerFactory.cpp:50
virtual bool configure(ModelTransformer::Input &input, Transform &transform, std::shared_ptr< Vamp::PluginBase > plugin, ModelId &inputModel, AudioPlaySource *source, sv_frame_t startFrame, sv_frame_t duration, const QMap< QString, ModelId > &modelMap, QStringList candidateModelNames, QString defaultModelName)=0
Definition: ModelTransformer.h:44
static RealTimePluginFactory * instanceFor(QString identifier)
Definition: RealTimePluginFactory.cpp:65
static ModelTransformerFactory * m_instance
Definition: ModelTransformerFactory.h:173
ModelTransformer::Input getConfigurationForTransform(Transform &transform, std::vector< ModelId > candidateInputModels, ModelId defaultInputModel, AudioPlaySource *source=0, sv_frame_t startFrame=0, sv_frame_t duration=0, UserConfigurator *configurator=0)
Fill out the configuration for the given transform (may include asking the user by calling back on th...
Definition: ModelTransformerFactory.cpp:60
std::vector< ModelId > transformMultiple(const Transforms &transform, const ModelTransformer::Input &input, QString &message, AdditionalModelHandler *handler=0)
Return the multiple output models resulting from applying the named transforms to the given input mod...
Definition: ModelTransformerFactory.cpp:222
virtual QString toXmlString(QString indent="", QString extraAttributes="") const
Convert this exportable object to XML in a string.
Definition: XmlExportable.cpp:25
QString getTransformFriendlyName(TransformId identifier)
Brief but friendly name of a transform, suitable for use as the name of the output layer...
Definition: TransformFactory.cpp:863
static TransformFactory * getInstance()
Definition: TransformFactory.cpp:48
virtual std::shared_ptr< RealTimePluginInstance > instantiatePlugin(QString identifier, int clientId, int position, sv_samplerate_t sampleRate, int blockSize, int channels)=0
Instantiate a plugin.
virtual sv_samplerate_t getSourceSampleRate() const =0
Return the sample rate of the source material – any material that wants to play at a different rate ...
virtual ~ModelTransformerFactory()
Definition: ModelTransformerFactory.cpp:55
void transformFailed(QString transformName, QString message)
TransformerConfigurationMap m_lastConfigurations
Definition: ModelTransformerFactory.h:165
TransformerSet m_runningTransformers
Definition: ModelTransformerFactory.h:168
virtual Models getAdditionalOutputModels()
Return any additional models that were created during processing.
Definition: ModelTransformer.h:103
void transformerFinished()
Definition: ModelTransformerFactory.cpp:276
virtual std::shared_ptr< Vamp::Plugin > instantiatePlugin(QString identifier, sv_samplerate_t inputSampleRate)=0
Instantiate (load) and return pointer to the plugin with the given identifier, at the given sample ra...
virtual bool willHaveAdditionalOutputModels()
Return true if the current transform is one that may produce additional models (to be retrieved throu...
Definition: ModelTransformer.h:110
Definition: Transform.h:34
static FeatureExtractionPluginFactory * instance()
Definition: FeatureExtractionPluginFactory.cpp:26
bool in_range_for(const C &container, T i)
Check whether an integer index is in range for a container, avoiding overflows and signed/unsigned co...
Definition: BaseTypes.h:37
Models getOutputModels()
Return the set of output model IDs created by the transform or transforms.
Definition: ModelTransformer.h:91
A ModelTransformer turns one data model into another.
Definition: ModelTransformer.h:37
bool haveRunningTransformers() const
Definition: ModelTransformerFactory.cpp:336
virtual int getTargetBlockSize() const =0
Get the block size of the target audio device.
bool isAbandoned() const
Return true if the processing thread is being or has been abandoned, i.e.
Definition: ModelTransformer.h:72
Definition: ById.h:115
Definition: PluginXml.h:26
Generated by
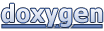