svcore
1.9
|
#include <FeatureExtractionModelTransformer.h>
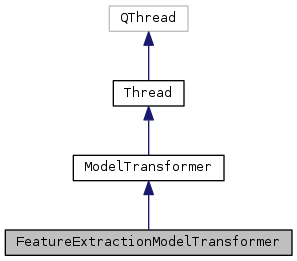
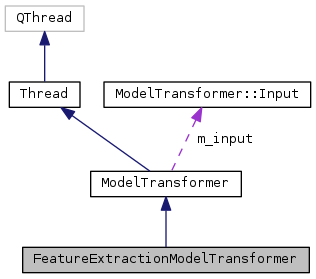
Public Types | |
typedef std::vector< ModelId > | Models |
enum | Type { RTThread, NonRTThread } |
Public Slots | |
void | start () |
Public Member Functions | |
FeatureExtractionModelTransformer (Input input, const Transform &transform) | |
FeatureExtractionModelTransformer (Input input, const Transforms &relatedTransforms) | |
Obtain outputs for a set of transforms that all use the same plugin and input (but with different outputs). More... | |
virtual | ~FeatureExtractionModelTransformer () |
Models | getAdditionalOutputModels () override |
Return any additional models that were created during processing. More... | |
bool | willHaveAdditionalOutputModels () override |
Return true if the current transform is one that may produce additional models (to be retrieved through getAdditionalOutputModels above). More... | |
void | abandon () |
Hint to the processing thread that it should give up, for example because the process is going to exit or the model/document context is being replaced. More... | |
bool | isAbandoned () const |
Return true if the processing thread is being or has been abandoned, i.e. More... | |
ModelId | getInputModel () |
Return the input model for the transform. More... | |
int | getInputChannel () |
Return the input channel spec for the transform. More... | |
Models | getOutputModels () |
Return the set of output model IDs created by the transform or transforms. More... | |
QString | getMessage () const |
Return a warning or error message. More... | |
Protected Types | |
typedef std::map< int, std::map< int, ModelId > > | AdditionalModelMap |
Protected Member Functions | |
bool | initialise () |
void | deinitialise () |
void | run () override |
void | createOutputModels (int n) |
ModelId | getAdditionalModel (int transformNo, int binNo) |
void | addFeature (int n, sv_frame_t blockFrame, const Vamp::Plugin::Feature &feature) |
void | setCompletion (int, int) |
void | getFrames (int channelCount, sv_frame_t startFrame, sv_frame_t size, float **buffer) |
void | awaitOutputModels () override |
template<typename T > | |
bool | isOutputType (int n) |
template<typename T > | |
bool | setOutputCompletion (int n, int completion) |
Protected Attributes | |
std::shared_ptr< Vamp::Plugin > | m_plugin |
std::vector< Vamp::Plugin::OutputDescriptor > | m_descriptors |
std::vector< int > | m_fixedRateFeatureNos |
std::vector< int > | m_outputNos |
std::map< int, bool > | m_needAdditionalModels |
AdditionalModelMap | m_additionalModels |
bool | m_haveOutputs |
QMutex | m_outputMutex |
QWaitCondition | m_outputsCondition |
Transforms | m_transforms |
Input | m_input |
Models | m_outputs |
bool | m_abandoned |
QString | m_message |
Detailed Description
Definition at line 33 of file FeatureExtractionModelTransformer.h.
Member Typedef Documentation
|
protected |
Definition at line 78 of file FeatureExtractionModelTransformer.h.
|
inherited |
Definition at line 42 of file ModelTransformer.h.
Member Enumeration Documentation
|
inherited |
Constructor & Destructor Documentation
FeatureExtractionModelTransformer::FeatureExtractionModelTransformer | ( | Input | input, |
const Transform & | transform | ||
) |
Definition at line 44 of file FeatureExtractionModelTransformer.cpp.
References ModelTransformer::m_transforms, and SVDEBUG.
FeatureExtractionModelTransformer::FeatureExtractionModelTransformer | ( | Input | input, |
const Transforms & | relatedTransforms | ||
) |
Obtain outputs for a set of transforms that all use the same plugin and input (but with different outputs).
i.e. run the plugin once only and collect more than one output from it.
Definition at line 53 of file FeatureExtractionModelTransformer.cpp.
References ModelTransformer::m_transforms, and SVDEBUG.
|
virtual |
Definition at line 558 of file FeatureExtractionModelTransformer.cpp.
Member Function Documentation
|
overridevirtual |
Return any additional models that were created during processing.
This might happen if, for example, a transform was configured to split a multi-bin output into separate single-bin models as it processed. These should not be queried until after the transform has completed.
Reimplemented from ModelTransformer.
Definition at line 566 of file FeatureExtractionModelTransformer.cpp.
References m_additionalModels.
|
overridevirtual |
Return true if the current transform is one that may produce additional models (to be retrieved through getAdditionalOutputModels above).
Reimplemented from ModelTransformer.
Definition at line 578 of file FeatureExtractionModelTransformer.cpp.
References m_needAdditionalModels.
|
protected |
Definition at line 75 of file FeatureExtractionModelTransformer.cpp.
References areTransformsSimilar(), createOutputModels(), Transform::getBlockSize(), ModelTransformer::getInputModel(), TransformFactory::getInstance(), Transform::getPluginIdentifier(), Transform::getPluginVersion(), Transform::getStepSize(), in_range_for(), FeatureExtractionPluginFactory::instance(), FeatureExtractionPluginFactory::instantiatePlugin(), m_descriptors, m_fixedRateFeatureNos, m_haveOutputs, ModelTransformer::m_message, m_outputMutex, m_outputNos, m_outputsCondition, m_plugin, ModelTransformer::m_transforms, TransformFactory::makeContextConsistentWithPlugin(), setCompletion(), TransformFactory::setPluginParameters(), SVCERR, and SVDEBUG.
Referenced by run().
|
protected |
Definition at line 264 of file FeatureExtractionModelTransformer.cpp.
References m_descriptors, ModelTransformer::m_message, m_plugin, SVCERR, and SVDEBUG.
Referenced by run().
|
overrideprotectedvirtual |
Implements Thread.
Definition at line 637 of file FeatureExtractionModelTransformer.cpp.
References ModelTransformer::abandon(), addFeature(), deinitialise(), RealTime::frame2RealTime(), TypedById< Item, Id >::get(), Transform::getBlockSize(), ModelTransformer::Input::getChannel(), Transform::getDuration(), FFTModel::getError(), getFrames(), ModelTransformer::getInputModel(), Transform::getStartTime(), Transform::getStepSize(), Transform::getWindowType(), in_range_for(), initialise(), FFTModel::isOK(), ModelTransformer::m_abandoned, ModelTransformer::m_input, ModelTransformer::m_message, m_outputNos, ModelTransformer::m_outputs, m_plugin, ModelTransformer::m_transforms, RealTime::realTime2Frame(), setCompletion(), SVCERR, and SVDEBUG.
|
protected |
!! SV doesn't actually support display of models that have !! different underlying rates together – so we always set !! the model rate to be the input model's rate, and adjust !! the resolution appropriately. We can't properly display !! data with a higher resolution than the base model at all
Definition at line 284 of file FeatureExtractionModelTransformer.cpp.
References TypedById< Item, Id >::add(), NoteModel::FLEXI_NOTE, ModelTransformer::getInputModel(), PluginRDFDescription::getOutputEventTypeURI(), PluginRDFDescription::getOutputSignalTypeURI(), m_descriptors, m_needAdditionalModels, m_outputNos, ModelTransformer::m_outputs, m_plugin, ModelTransformer::m_transforms, NoteModel::NORMAL_NOTE, RegionModel::setScaleUnits(), SparseTimeValueModel::setScaleUnits(), NoteModel::setScaleUnits(), and SVDEBUG.
Referenced by initialise().
|
protected |
Definition at line 587 of file FeatureExtractionModelTransformer.cpp.
References TypedById< Item, Id >::add(), in_range_for(), m_additionalModels, m_needAdditionalModels, ModelTransformer::m_outputs, Model::setRDFTypeURI(), SparseTimeValueModel::setScaleUnits(), SVCERR, and SVDEBUG.
Referenced by addFeature().
|
protected |
Definition at line 1004 of file FeatureExtractionModelTransformer.cpp.
References ModelTransformer::abandon(), TypedById< Item, Id >::get(), getAdditionalModel(), TypedById< Item, Id >::getAs(), ModelTransformer::getInputModel(), in_range_for(), m_descriptors, m_fixedRateFeatureNos, m_needAdditionalModels, ModelTransformer::m_outputs, RealTime::realTime2Frame(), BasicCompressedDenseThreeDimensionalModel::setColumn(), SVDEBUG, and RealTime::toDouble().
Referenced by run().
|
protected |
Definition at line 1193 of file FeatureExtractionModelTransformer.cpp.
References SVDEBUG.
Referenced by initialise(), and run().
|
protected |
Definition at line 943 of file FeatureExtractionModelTransformer.cpp.
References ModelTransformer::Input::getChannel(), ModelTransformer::getInputModel(), in_range_for(), and ModelTransformer::m_input.
Referenced by run().
|
overrideprotectedvirtual |
Implements ModelTransformer.
Definition at line 549 of file FeatureExtractionModelTransformer.cpp.
References ModelTransformer::m_abandoned, m_haveOutputs, m_outputMutex, and m_outputsCondition.
|
inlineprotected |
Definition at line 98 of file FeatureExtractionModelTransformer.h.
References ModelTransformer::m_outputs.
|
inlineprotected |
Definition at line 106 of file FeatureExtractionModelTransformer.h.
References ModelTransformer::m_outputs.
|
inlineinherited |
Hint to the processing thread that it should give up, for example because the process is going to exit or the model/document context is being replaced.
Caller should still wait() to be sure that processing has ended.
Definition at line 66 of file ModelTransformer.h.
References ModelTransformer::m_abandoned.
Referenced by addFeature(), RealTimeEffectModelTransformer::run(), and run().
|
inlineinherited |
Return true if the processing thread is being or has been abandoned, i.e.
if abandon() has been called.
Definition at line 72 of file ModelTransformer.h.
References ModelTransformer::m_abandoned.
Referenced by ModelTransformerFactory::transformerFinished().
|
inlineinherited |
Return the input model for the transform.
Definition at line 77 of file ModelTransformer.h.
References ModelTransformer::Input::getModel(), and ModelTransformer::m_input.
Referenced by addFeature(), createOutputModels(), getFrames(), initialise(), RealTimeEffectModelTransformer::RealTimeEffectModelTransformer(), RealTimeEffectModelTransformer::run(), and run().
|
inlineinherited |
Return the input channel spec for the transform.
Definition at line 82 of file ModelTransformer.h.
References ModelTransformer::Input::getChannel(), and ModelTransformer::m_input.
|
inlineinherited |
Return the set of output model IDs created by the transform or transforms.
Returns an empty list if any transform could not be initialised; an error message may be available via getMessage() in this situation. The returned models have been added to ModelById.
Definition at line 91 of file ModelTransformer.h.
References ModelTransformer::awaitOutputModels(), and ModelTransformer::m_outputs.
Referenced by ModelTransformerFactory::transformMultiple().
|
inlineinherited |
Return a warning or error message.
If getOutputModel returned a null pointer, this should contain a fatal error message for the transformer; otherwise it may contain a warning to show to the user about e.g. suboptimal block size or whatever.
Definition at line 118 of file ModelTransformer.h.
References ModelTransformer::awaitOutputModels(), ModelTransformer::m_message, and ModelTransformer::ModelTransformer().
Referenced by ModelTransformerFactory::transformerFinished(), and ModelTransformerFactory::transformMultiple().
|
slotinherited |
Definition at line 34 of file Thread.cpp.
References Thread::m_type, and Thread::RTThread.
Referenced by BQAFileReader::BQAFileReader(), DecodingWavFileReader::DecodingWavFileReader(), ReadOnlyWaveFileModel::fillCache(), MP3FileReader::MP3FileReader(), DSSIPluginInstance::requestNonRTThread(), and ModelTransformerFactory::transformMultiple().
Member Data Documentation
|
protected |
Definition at line 61 of file FeatureExtractionModelTransformer.h.
Referenced by createOutputModels(), deinitialise(), initialise(), and run().
|
protected |
Definition at line 64 of file FeatureExtractionModelTransformer.h.
Referenced by addFeature(), createOutputModels(), deinitialise(), and initialise().
|
protected |
Definition at line 67 of file FeatureExtractionModelTransformer.h.
Referenced by addFeature(), and initialise().
|
protected |
Definition at line 70 of file FeatureExtractionModelTransformer.h.
Referenced by createOutputModels(), initialise(), and run().
|
protected |
Definition at line 75 of file FeatureExtractionModelTransformer.h.
Referenced by addFeature(), createOutputModels(), getAdditionalModel(), and willHaveAdditionalOutputModels().
|
protected |
Definition at line 80 of file FeatureExtractionModelTransformer.h.
Referenced by getAdditionalModel(), and getAdditionalOutputModels().
|
protected |
Definition at line 93 of file FeatureExtractionModelTransformer.h.
Referenced by awaitOutputModels(), and initialise().
|
protected |
Definition at line 94 of file FeatureExtractionModelTransformer.h.
Referenced by awaitOutputModels(), and initialise().
|
protected |
Definition at line 95 of file FeatureExtractionModelTransformer.h.
Referenced by awaitOutputModels(), and initialise().
|
protectedinherited |
Definition at line 126 of file ModelTransformer.h.
Referenced by createOutputModels(), FeatureExtractionModelTransformer(), initialise(), ModelTransformer::ModelTransformer(), RealTimeEffectModelTransformer::RealTimeEffectModelTransformer(), RealTimeEffectModelTransformer::run(), and run().
|
protectedinherited |
Definition at line 127 of file ModelTransformer.h.
Referenced by getFrames(), ModelTransformer::getInputChannel(), ModelTransformer::getInputModel(), RealTimeEffectModelTransformer::run(), and run().
|
protectedinherited |
Definition at line 128 of file ModelTransformer.h.
Referenced by addFeature(), createOutputModels(), getAdditionalModel(), ModelTransformer::getOutputModels(), isOutputType(), RealTimeEffectModelTransformer::RealTimeEffectModelTransformer(), RealTimeEffectModelTransformer::run(), run(), and setOutputCompletion().
|
protectedinherited |
Definition at line 129 of file ModelTransformer.h.
Referenced by ModelTransformer::abandon(), awaitOutputModels(), ModelTransformer::isAbandoned(), RealTimeEffectModelTransformer::run(), run(), and ModelTransformer::~ModelTransformer().
|
protectedinherited |
Definition at line 130 of file ModelTransformer.h.
Referenced by deinitialise(), ModelTransformer::getMessage(), initialise(), and run().
The documentation for this class was generated from the following files:
Generated by
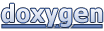