svcore
1.9
|
ModelTransformer.h
Go to the documentation of this file.
void abandon()
Hint to the processing thread that it should give up, for example because the process is going to exi...
Definition: ModelTransformer.h:66
int getInputChannel()
Return the input channel spec for the transform.
Definition: ModelTransformer.h:82
virtual void awaitOutputModels()=0
ModelTransformer(Input input, const Transform &transform)
Definition: ModelTransformer.cpp:18
Definition: ModelTransformer.h:44
virtual Models getAdditionalOutputModels()
Return any additional models that were created during processing.
Definition: ModelTransformer.h:103
virtual bool willHaveAdditionalOutputModels()
Return true if the current transform is one that may produce additional models (to be retrieved throu...
Definition: ModelTransformer.h:110
Definition: Transform.h:34
Models getOutputModels()
Return the set of output model IDs created by the transform or transforms.
Definition: ModelTransformer.h:91
Definition: Thread.h:24
A ModelTransformer turns one data model into another.
Definition: ModelTransformer.h:37
bool isAbandoned() const
Return true if the processing thread is being or has been abandoned, i.e.
Definition: ModelTransformer.h:72
Definition: ById.h:115
Generated by
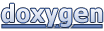