svcore
1.9
|
A ModelTransformer turns one data model into another. More...
#include <ModelTransformer.h>
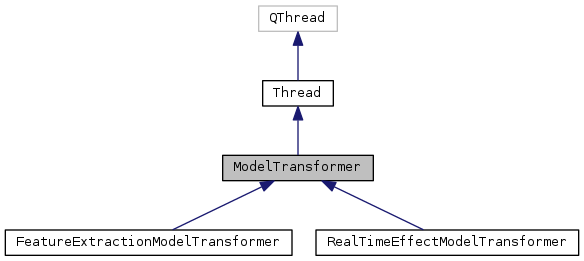
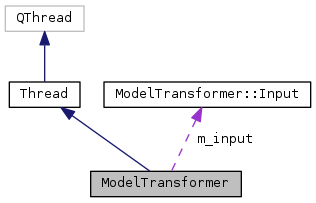
Classes | |
class | Input |
Public Types | |
typedef std::vector< ModelId > | Models |
enum | Type { RTThread, NonRTThread } |
Public Slots | |
void | start () |
Public Member Functions | |
virtual | ~ModelTransformer () |
void | abandon () |
Hint to the processing thread that it should give up, for example because the process is going to exit or the model/document context is being replaced. More... | |
bool | isAbandoned () const |
Return true if the processing thread is being or has been abandoned, i.e. More... | |
ModelId | getInputModel () |
Return the input model for the transform. More... | |
int | getInputChannel () |
Return the input channel spec for the transform. More... | |
Models | getOutputModels () |
Return the set of output model IDs created by the transform or transforms. More... | |
virtual Models | getAdditionalOutputModels () |
Return any additional models that were created during processing. More... | |
virtual bool | willHaveAdditionalOutputModels () |
Return true if the current transform is one that may produce additional models (to be retrieved through getAdditionalOutputModels above). More... | |
QString | getMessage () const |
Return a warning or error message. More... | |
Protected Member Functions | |
ModelTransformer (Input input, const Transform &transform) | |
ModelTransformer (Input input, const Transforms &transforms) | |
virtual void | awaitOutputModels ()=0 |
void | run () override=0 |
Protected Attributes | |
Transforms | m_transforms |
Input | m_input |
Models | m_outputs |
bool | m_abandoned |
QString | m_message |
Detailed Description
A ModelTransformer turns one data model into another.
Typically in this application, a ModelTransformer might have a DenseTimeValueModel as its input (e.g. an audio waveform) and a SparseOneDimensionalModel (e.g. detected beats) as its output.
The ModelTransformer typically runs in the background, as a separate thread populating the output model. The model is available to the user of the ModelTransformer immediately, but may be initially empty until the background thread has populated it.
Definition at line 37 of file ModelTransformer.h.
Member Typedef Documentation
typedef std::vector<ModelId> ModelTransformer::Models |
Definition at line 42 of file ModelTransformer.h.
Member Enumeration Documentation
|
inherited |
Constructor & Destructor Documentation
|
virtual |
Definition at line 32 of file ModelTransformer.cpp.
References m_abandoned.
Definition at line 18 of file ModelTransformer.cpp.
References m_transforms.
Referenced by getMessage().
|
protected |
Definition at line 25 of file ModelTransformer.cpp.
Member Function Documentation
|
inline |
Hint to the processing thread that it should give up, for example because the process is going to exit or the model/document context is being replaced.
Caller should still wait() to be sure that processing has ended.
Definition at line 66 of file ModelTransformer.h.
References m_abandoned.
Referenced by FeatureExtractionModelTransformer::addFeature(), RealTimeEffectModelTransformer::run(), and FeatureExtractionModelTransformer::run().
|
inline |
Return true if the processing thread is being or has been abandoned, i.e.
if abandon() has been called.
Definition at line 72 of file ModelTransformer.h.
References m_abandoned.
Referenced by ModelTransformerFactory::transformerFinished().
|
inline |
Return the input model for the transform.
Definition at line 77 of file ModelTransformer.h.
References ModelTransformer::Input::getModel(), and m_input.
Referenced by FeatureExtractionModelTransformer::addFeature(), FeatureExtractionModelTransformer::createOutputModels(), FeatureExtractionModelTransformer::getFrames(), FeatureExtractionModelTransformer::initialise(), RealTimeEffectModelTransformer::RealTimeEffectModelTransformer(), RealTimeEffectModelTransformer::run(), and FeatureExtractionModelTransformer::run().
|
inline |
Return the input channel spec for the transform.
Definition at line 82 of file ModelTransformer.h.
References ModelTransformer::Input::getChannel(), and m_input.
|
inline |
Return the set of output model IDs created by the transform or transforms.
Returns an empty list if any transform could not be initialised; an error message may be available via getMessage() in this situation. The returned models have been added to ModelById.
Definition at line 91 of file ModelTransformer.h.
References awaitOutputModels(), and m_outputs.
Referenced by ModelTransformerFactory::transformMultiple().
|
inlinevirtual |
Return any additional models that were created during processing.
This might happen if, for example, a transform was configured to split a multi-bin output into separate single-bin models as it processed. These should not be queried until after the transform has completed.
Reimplemented in FeatureExtractionModelTransformer.
Definition at line 103 of file ModelTransformer.h.
Referenced by ModelTransformerFactory::transformerFinished().
|
inlinevirtual |
Return true if the current transform is one that may produce additional models (to be retrieved through getAdditionalOutputModels above).
Reimplemented in FeatureExtractionModelTransformer.
Definition at line 110 of file ModelTransformer.h.
Referenced by ModelTransformerFactory::transformerFinished().
|
inline |
Return a warning or error message.
If getOutputModel returned a null pointer, this should contain a fatal error message for the transformer; otherwise it may contain a warning to show to the user about e.g. suboptimal block size or whatever.
Definition at line 118 of file ModelTransformer.h.
References awaitOutputModels(), m_message, and ModelTransformer().
Referenced by ModelTransformerFactory::transformerFinished(), and ModelTransformerFactory::transformMultiple().
|
protectedpure virtual |
Implemented in FeatureExtractionModelTransformer, and RealTimeEffectModelTransformer.
Referenced by getMessage(), and getOutputModels().
|
slotinherited |
Definition at line 34 of file Thread.cpp.
References Thread::m_type, and Thread::RTThread.
Referenced by BQAFileReader::BQAFileReader(), DecodingWavFileReader::DecodingWavFileReader(), ReadOnlyWaveFileModel::fillCache(), MP3FileReader::MP3FileReader(), DSSIPluginInstance::requestNonRTThread(), and ModelTransformerFactory::transformMultiple().
|
overrideprotectedpure virtualinherited |
Member Data Documentation
|
protected |
Definition at line 126 of file ModelTransformer.h.
Referenced by FeatureExtractionModelTransformer::createOutputModels(), FeatureExtractionModelTransformer::FeatureExtractionModelTransformer(), FeatureExtractionModelTransformer::initialise(), ModelTransformer(), RealTimeEffectModelTransformer::RealTimeEffectModelTransformer(), RealTimeEffectModelTransformer::run(), and FeatureExtractionModelTransformer::run().
|
protected |
Definition at line 127 of file ModelTransformer.h.
Referenced by FeatureExtractionModelTransformer::getFrames(), getInputChannel(), getInputModel(), RealTimeEffectModelTransformer::run(), and FeatureExtractionModelTransformer::run().
|
protected |
Definition at line 128 of file ModelTransformer.h.
Referenced by FeatureExtractionModelTransformer::addFeature(), FeatureExtractionModelTransformer::createOutputModels(), FeatureExtractionModelTransformer::getAdditionalModel(), getOutputModels(), FeatureExtractionModelTransformer::isOutputType(), RealTimeEffectModelTransformer::RealTimeEffectModelTransformer(), RealTimeEffectModelTransformer::run(), FeatureExtractionModelTransformer::run(), and FeatureExtractionModelTransformer::setOutputCompletion().
|
protected |
Definition at line 129 of file ModelTransformer.h.
Referenced by abandon(), FeatureExtractionModelTransformer::awaitOutputModels(), isAbandoned(), RealTimeEffectModelTransformer::run(), FeatureExtractionModelTransformer::run(), and ~ModelTransformer().
|
protected |
Definition at line 130 of file ModelTransformer.h.
Referenced by FeatureExtractionModelTransformer::deinitialise(), getMessage(), FeatureExtractionModelTransformer::initialise(), and FeatureExtractionModelTransformer::run().
The documentation for this class was generated from the following files:
Generated by
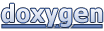