svcore
1.9
|
FeatureExtractionModelTransformer.h
Go to the documentation of this file.
bool m_haveOutputs
Definition: FeatureExtractionModelTransformer.h:93
void awaitOutputModels() override
Definition: FeatureExtractionModelTransformer.cpp:549
std::shared_ptr< Vamp::Plugin > m_plugin
Definition: FeatureExtractionModelTransformer.h:61
void addFeature(int n, sv_frame_t blockFrame, const Vamp::Plugin::Feature &feature)
Definition: FeatureExtractionModelTransformer.cpp:1004
ModelId getAdditionalModel(int transformNo, int binNo)
Definition: FeatureExtractionModelTransformer.cpp:587
std::vector< int > m_fixedRateFeatureNos
Definition: FeatureExtractionModelTransformer.h:67
std::map< int, std::map< int, ModelId > > AdditionalModelMap
Definition: FeatureExtractionModelTransformer.h:78
virtual ~FeatureExtractionModelTransformer()
Definition: FeatureExtractionModelTransformer.cpp:558
Definition: ModelTransformer.h:44
bool initialise()
Definition: FeatureExtractionModelTransformer.cpp:75
void run() override
Definition: FeatureExtractionModelTransformer.cpp:637
std::vector< Vamp::Plugin::OutputDescriptor > m_descriptors
Definition: FeatureExtractionModelTransformer.h:64
void deinitialise()
Definition: FeatureExtractionModelTransformer.cpp:264
bool setOutputCompletion(int n, int completion)
Definition: FeatureExtractionModelTransformer.h:106
QMutex m_outputMutex
Definition: FeatureExtractionModelTransformer.h:94
AdditionalModelMap m_additionalModels
Definition: FeatureExtractionModelTransformer.h:80
FeatureExtractionModelTransformer(Input input, const Transform &transform)
Definition: FeatureExtractionModelTransformer.cpp:44
bool willHaveAdditionalOutputModels() override
Return true if the current transform is one that may produce additional models (to be retrieved throu...
Definition: FeatureExtractionModelTransformer.cpp:578
void getFrames(int channelCount, sv_frame_t startFrame, sv_frame_t size, float **buffer)
Definition: FeatureExtractionModelTransformer.cpp:943
Models getAdditionalOutputModels() override
Return any additional models that were created during processing.
Definition: FeatureExtractionModelTransformer.cpp:566
std::vector< int > m_outputNos
Definition: FeatureExtractionModelTransformer.h:70
bool isOutputType(int n)
Definition: FeatureExtractionModelTransformer.h:98
void createOutputModels(int n)
Definition: FeatureExtractionModelTransformer.cpp:284
Definition: Transform.h:34
A model representing a wiggly-line plot with points at arbitrary intervals of the model resolution...
Definition: SparseTimeValueModel.h:34
Base class for models containing dense two-dimensional data (value against time). ...
Definition: DenseTimeValueModel.h:30
A ModelTransformer turns one data model into another.
Definition: ModelTransformer.h:37
std::map< int, bool > m_needAdditionalModels
Definition: FeatureExtractionModelTransformer.h:75
void setCompletion(int, int)
Definition: FeatureExtractionModelTransformer.cpp:1193
Definition: ById.h:115
QWaitCondition m_outputsCondition
Definition: FeatureExtractionModelTransformer.h:95
Generated by
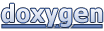