svcore
1.9
|
DecodingWavFileReader.cpp
Go to the documentation of this file.
static bool supportsContentType(QString type)
Definition: DecodingWavFileReader.cpp:193
Definition: ProgressReporter.h:22
void run() override
Definition: DecodingWavFileReader.cpp:125
static bool supports(FileSource &source)
Definition: DecodingWavFileReader.cpp:199
Definition: CodedAudioFileReader.h:40
static bool supportsExtension(QString ext)
Definition: WavFileReader.cpp:309
void addBlock(const floatvec_t &frames)
Definition: DecodingWavFileReader.cpp:161
static bool supportsContentType(QString type)
Definition: WavFileReader.cpp:317
std::vector< float, breakfastquay::StlAllocator< float > > floatvec_t
Definition: BaseTypes.h:53
DecodeThread * m_decodeThread
Definition: DecodingWavFileReader.h:86
static void getSupportedExtensions(std::set< QString > &extensions)
Definition: DecodingWavFileReader.cpp:181
virtual ~DecodingWavFileReader()
Definition: DecodingWavFileReader.cpp:107
FileSource is a class used to refer to the contents of a file that may be either local or at a remote...
Definition: FileSource.h:59
static void getSupportedExtensions(std::set< QString > &extensions)
Definition: WavFileReader.cpp:280
void addSamplesToDecodeCache(float **samples, sv_frame_t nframes)
Definition: CodedAudioFileReader.cpp:251
QString getError() const override
If isOK() is false, return an error string.
Definition: WavFileReader.h:52
virtual void setProgress(int percentage)=0
bool isOK() const
Return true if the file was opened successfully and no error has subsequently occurred.
Definition: AudioFileReader.h:38
DecodingWavFileReader(FileSource source, DecodeMode decodeMode, CacheMode cacheMode, sv_samplerate_t targetRate=0, bool normalised=false, ProgressReporter *reporter=0)
Definition: DecodingWavFileReader.cpp:26
void progress(int)
std::atomic< bool > m_cancelled
Definition: DecodingWavFileReader.h:67
void finishDecodeCache()
Definition: CodedAudioFileReader.cpp:321
QString getTitle() const override
Return the title of the work in the audio file, if known.
Definition: WavFileReader.h:54
ProgressReporter * m_reporter
Definition: DecodingWavFileReader.h:72
void initialiseDecodeCache()
Definition: CodedAudioFileReader.cpp:127
virtual void setMessage(QString text)=0
sv_frame_t getFrameCount() const
Return the number of audio sample frames (i.e.
Definition: AudioFileReader.h:49
floatvec_t getInterleavedFrames(sv_frame_t start, sv_frame_t count) const override
Must be safe to call from multiple threads with different arguments on the same object at the same ti...
Definition: WavFileReader.cpp:164
bool isDecodeCacheInitialised() const
Definition: CodedAudioFileReader.h:87
QString getMaker() const override
Return the "maker" of the work in the audio file, if known.
Definition: WavFileReader.h:55
static bool supportsExtension(QString ext)
Definition: DecodingWavFileReader.cpp:187
sv_samplerate_t getSampleRate() const
Return the samplerate at which the file is being read.
Definition: AudioFileReader.h:62
int getChannelCount() const
Return the number of channels in the file.
Definition: AudioFileReader.h:54
Generated by
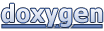